用Java写一个贪吃蛇游戏
时间: 2024-01-02 15:03:05 浏览: 172
很高兴为您提供一个用Java写的贪吃蛇游戏代码。这个游戏是一个控制台应用程序,您可以在控制台上玩这个游戏。下面是完整的代码:
```java
import java.util.ArrayList;
import java.util.Random;
import java.util.Scanner;
public class SnakeGame {
private final int width;
private final int height;
private int score;
private final ArrayList<Point> snake;
private Point food;
private final Random rand = new Random();
private Direction direction = Direction.RIGHT;
private boolean gameOver = false;
public SnakeGame(int width, int height) {
this.width = width;
this.height = height;
this.score = 0;
this.snake = new ArrayList<>();
this.snake.add(new Point(width / 2, height / 2));
this.food = generateFood();
}
public void play() {
Scanner scanner = new Scanner(System.in);
while (!gameOver) {
System.out.println("Score: " + score);
draw();
System.out.print("Enter direction (up/down/left/right): ");
String input = scanner.nextLine();
switch (input) {
case "up":
direction = Direction.UP;
break;
case "down":
direction = Direction.DOWN;
break;
case "left":
direction = Direction.LEFT;
break;
case "right":
direction = Direction.RIGHT;
break;
default:
System.out.println("Invalid direction.");
break;
}
move();
checkCollision();
if (!gameOver && snake.get(0).equals(food)) {
score++;
snake.add(food);
food = generateFood();
}
}
System.out.println("Game over! Final score: " + score);
}
private void draw() {
char[][] grid = new char[height][width];
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
grid[i][j] = '.';
}
}
for (Point p : snake) {
grid[p.getY()][p.getX()] = 'o';
}
grid[food.getY()][food.getX()] = 'x';
for (int i = 0; i < width + 2; i++) {
System.out.print("-");
}
System.out.println();
for (int i = 0; i < height; i++) {
System.out.print("|");
for (int j = 0; j < width; j++) {
System.out.print(grid[i][j]);
}
System.out.println("|");
}
for (int i = 0; i < width + 2; i++) {
System.out.print("-");
}
System.out.println();
}
private void move() {
Point head = snake.get(0);
Point newHead;
switch (direction) {
case UP:
newHead = new Point(head.getX(), head.getY() - 1);
break;
case DOWN:
newHead = new Point(head.getX(), head.getY() + 1);
break;
case LEFT:
newHead = new Point(head.getX() - 1, head.getY());
break;
case RIGHT:
newHead = new Point(head.getX() + 1, head.getY());
break;
default:
throw new IllegalStateException("Invalid direction.");
}
snake.add(0, newHead);
snake.remove(snake.size() - 1);
}
private void checkCollision() {
Point head = snake.get(0);
if (head.getX() < 0 || head.getX() >= width || head.getY() < 0 || head.getY() >= height) {
gameOver = true;
return;
}
for (int i = 1; i < snake.size(); i++) {
if (head.equals(snake.get(i))) {
gameOver = true;
return;
}
}
}
private Point generateFood() {
Point food;
do {
int x = rand.nextInt(width);
int y = rand.nextInt(height);
food = new Point(x, y);
} while (snake.contains(food));
return food;
}
public static void main(String[] args) {
SnakeGame game = new SnakeGame(20, 10);
game.play();
}
private enum Direction {
UP, DOWN, LEFT, RIGHT
}
private static class Point {
private final int x;
private final int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Point point = (Point) o;
return x == point.x && y == point.y;
}
@Override
public int hashCode() {
return Objects.hash(x, y);
}
}
}
```
这个游戏的核心是一个 `SnakeGame` 类,它包含了贪吃蛇游戏的逻辑。在 `main` 方法中,我们创建了一个 `SnakeGame` 对象并调用 `play` 方法来启动游戏。在 `play` 方法中,我们从控制台读取用户输入的方向,并根据方向移动贪吃蛇。在每一次移动后,我们检查是否发生了碰撞,如果发生了碰撞,游戏结束。如果贪吃蛇吃到了食物,我们增加分数并且在贪吃蛇的身体上增加一个新的格子。
这个游戏也使用了一个 `Point` 类来表示贪吃蛇的身体和食物的位置。我们使用一个二维字符数组来表示游戏的画面,并使用 `draw` 方法来在控制台上输出这个画面。在 `checkCollision` 方法中,我们检查是否有任何碰撞发生,包括贪吃蛇是否碰到了游戏边界或者碰到了它自己。
这个贪吃蛇游戏是一个简单而有趣的练手项目,它可以帮助您加深对Java编程语言的理解。
阅读全文
相关推荐
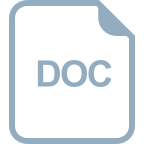
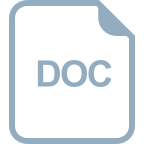
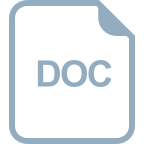







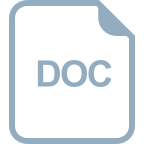
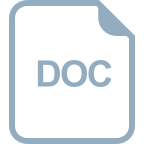
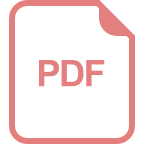
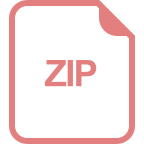
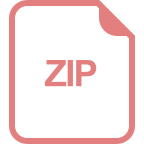