利用android studio设计一个记事本详细图文教程
时间: 2023-07-04 22:13:29 浏览: 66
好的,下面我会为您提供利用Android Studio设计一个记事本的详细图文教程,敬请参考。
1.创建新项目
打开Android Studio并创建一个新项目。选择“Empty Activity”作为您的模板。然后选择一个项目名称和位置,然后单击“Finish”按钮。
2.设计布局
设计记事本的布局。您需要添加一个EditText控件和一些按钮,例如保存,打开和新建。在XML文件中添加以下代码:
```
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:inputType="textMultiLine"
android:gravity="top|left"
android:padding="10dp"/>
<Button
android:id="@+id/newFile"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="New"/>
<Button
android:id="@+id/openFile"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Open"/>
<Button
android:id="@+id/saveFile"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Save"/>
```
3.处理按钮事件
接下来,您需要处理按钮的单击事件。在MainActivity.java文件中添加以下代码:
```
public class MainActivity extends AppCompatActivity {
private EditText editText;
private Button newFileButton, openFileButton, saveFileButton;
private String fileName = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = (EditText) findViewById(R.id.editText);
newFileButton = (Button) findViewById(R.id.newFile);
openFileButton = (Button) findViewById(R.id.openFile);
saveFileButton = (Button) findViewById(R.id.saveFile);
newFileButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
editText.setText("");
fileName = "";
}
});
openFileButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(Intent.ACTION_OPEN_DOCUMENT);
intent.addCategory(Intent.CATEGORY_OPENABLE);
intent.setType("text/plain");
startActivityForResult(intent, 1);
}
});
saveFileButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (fileName.equals("")) {
Intent intent = new Intent(Intent.ACTION_CREATE_DOCUMENT);
intent.addCategory(Intent.CATEGORY_OPENABLE);
intent.setType("text/plain");
intent.putExtra(Intent.EXTRA_TITLE, "Untitled.txt");
startActivityForResult(intent, 2);
} else {
saveFile(fileName);
}
}
});
}
private void saveFile(String fileName) {
try {
FileOutputStream fos = openFileOutput(fileName, MODE_PRIVATE);
fos.write(editText.getText().toString().getBytes());
fos.close();
Toast.makeText(this, "File saved.", Toast.LENGTH_SHORT).show();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
switch (requestCode) {
case 1:
try {
Uri uri = data.getData();
InputStream is = getContentResolver().openInputStream(uri);
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
StringBuilder stringBuilder = new StringBuilder();
String line = "";
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
}
is.close();
editText.setText(stringBuilder.toString());
fileName = uri.getLastPathSegment();
Toast.makeText(this, "File opened.", Toast.LENGTH_SHORT).show();
} catch (IOException e) {
e.printStackTrace();
}
break;
case 2:
Uri uri = data.getData();
fileName = uri.getLastPathSegment();
saveFile(fileName);
break;
}
}
}
}
```
4.运行应用程序
现在,您已经成功创建了一个简单的记事本应用程序。您可以运行该应用程序并使用它来编辑,保存和打开文本文件。
总结
以上就是利用Android Studio设计一个记事本的详细图文教程,希望对您有所帮助。如果您有任何疑问,请随时联系我。
相关推荐
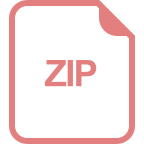














