java中string类型判空的方法是什么
时间: 2023-05-19 15:04:18 浏览: 142
在Java中,判断字符串是否为空可以使用以下方法:
1. 使用isEmpty()方法判断字符串是否为空,如果为空则返回true,否则返回false。
例如:
String str = "";
if(str.isEmpty()){
System.out.println("字符串为空");
}
2. 使用length()方法判断字符串长度是否为0,如果为0则表示字符串为空。
例如:
String str = "";
if(str.length() == 0){
System.out.println("字符串为空");
}
3. 使用StringUtils.isBlank()方法判断字符串是否为空或者只包含空格,如果是则返回true,否则返回false。
例如:
String str = "";
if(StringUtils.isBlank(str)){
System.out.println("字符串为空");
}
注意:StringUtils是Apache Commons Lang库中的一个工具类,需要导入相关的jar包才能使用。
相关问题
java的string类型如何判等
在 Java 中,判断两个 String 对象是否相等,通常有两种情况:
1. **使用 "==" 运算符**:这实际上是比较对象引用,即检查它们是否指向内存中的同一个对象。如果两个字符串是通过 new 关键字创建的不同实例,即使内容相同,也会返回 false。例如:
```java
String str1 = "hello";
String str2 = "hello";
System.out.println(str1 == str2); // 输出 false,因为 str1 和 str2 是两个不同的对象
```
2. **使用 ".equals()" 或者 "== .intern()"**:这两个方法用于比较字符串的内容。".equals()" 方法会比较字符串的字符序列,而 ".intern()" 方法会在常量池查找已经存在的字符串,如果找到了就直接返回,如果没有则插入并返回。两者结果一致:
```java
String str1 = "hello";
String str2 = "hello";
System.out.println(str1.equals(str2)); // 输出 true,因为两个字符串内容相同
// 使用 intern() 方法优化
String str3 = new String("hello");
str3 = str3.intern();
System.out.println(str1.equals(str3)); // 输出 true,字符串常量池里已有一个 hello,所以也是相等的
```
java 集合判空方法
Java集合类有一个isEmpty()方法,用于判断集合是否为空。该方法返回一个boolean类型的值,如果集合为空则返回true,否则返回false。以下是示例代码:
```
List<String> list = new ArrayList<>();
if (list.isEmpty()) {
System.out.println("List is empty");
}
Set<Integer> set = new HashSet<>();
if (set.isEmpty()) {
System.out.println("Set is empty");
}
Map<String, Integer> map = new HashMap<>();
if (map.isEmpty()) {
System.out.println("Map is empty");
}
```
上述代码展示了如何使用isEmpty()方法来判断List、Set和Map是否为空。
阅读全文
相关推荐
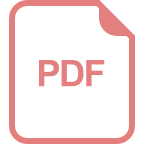
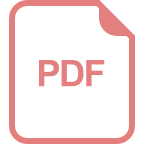
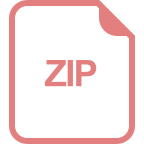
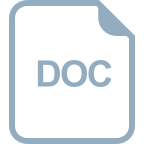











