用c++stl算法实现计算一个整数的阿尔法乘积。对于一个整数x来说,它的阿尔法乘积是这样来计算的:如果x是一个个位数,那么它的阿尔法乘积就是它本身;否则的话,x的阿 尔法乘积就等于它的各位非0的数字相乘所得到的那个整数的阿尔法乘积。例如:4018224312的阿尔法乘积等于8,它是按照以下的步骤来计算的: 4018224312 → 4*1*8*2*2*4*3*1*2 → 3072 → 3*7*2 → 42 → 4*2 → 8 编写一个程序,输入一个正整数(该整数不会超过6,000,000),输出它的阿尔法乘积。
时间: 2023-11-28 17:06:43 浏览: 85
可以使用以下代码实现:
```cpp
#include <iostream>
#include <algorithm>
#include <numeric>
#include <vector>
int main() {
int n;
std::cin >> n;
std::vector<int> digits;
while (n > 0) {
int digit = n % 10;
if (digit != 0) {
digits.push_back(digit);
}
n /= 10;
}
int alpha_product = std::accumulate(digits.begin(), digits.end(), 1, std::multiplies<int>());
while (alpha_product >= 10) {
digits.clear();
while (alpha_product > 0) {
digits.push_back(alpha_product % 10);
alpha_product /= 10;
}
alpha_product = std::accumulate(digits.begin(), digits.end(), 1, std::multiplies<int>());
}
std::cout << alpha_product << std::endl;
return 0;
}
```
首先,我们将输入的整数按照位数拆分成数字,并且将非零数字存储在一个 vector 中。然后,我们对这些数字进行乘积计算,得到一个新的数字。如果这个新的数字的位数大于1,我们就重复以上步骤,直到得到一个个位数的数字,即为阿尔法乘积。最后,我们输出这个阿尔法乘积即可。
相关推荐
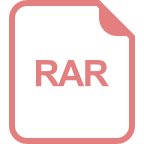
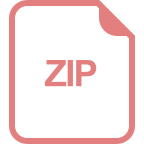
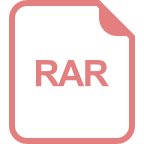
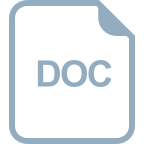
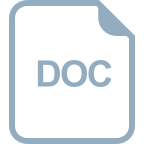
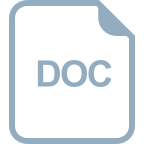
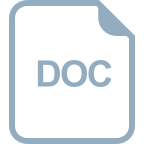
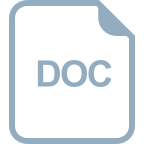
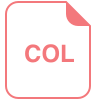
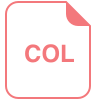
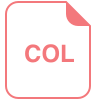
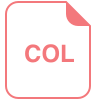
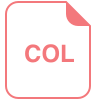




