#include<bits/stdc++.h> using namespace std; struct node { int l,r; }nodes[32]; int pre[32],in[32]; int n; int build(int a,int b,int c,int d) { int root=pre[a]; int m=c; while(in[m]!=root) m++; if(m==c) nodes[root].l=-1; else nodes[root].l=build(a+1,a+m-c,c,m-1); if(m==d) nodes[root].r=-1; else nodes[root].r=build(a+m-c+1,b,m+1,d); return root; } void bfs(int root) { if(root==-1) return; queue<int> q; q.push(root); while(!q.empty()) { int m=q.front(); if(m==root) cout<<m; else cout<<" "<<m; if(nodes[m].l!=-1) q.push(nodes[m].l); if(nodes[m].r!=-1) q.push(nodes[m].r); q.pop(); } } void Swap(int root) { int tmp=nodes[root].l; nodes[root].l=nodes[root].r; nodes[root].r=tmp; } void revswap(int root) { if(root==-1) return; revswap(nodes[root].l); revswap(nodes[root].r); Swap(root); } int main() { cin>>n; for(int i=0;i<n;i++) cin>>in[i]; for(int i=0;i<n;i++) cin>>pre[i]; int root=build(0,n-1,0,n-1); revswap(root); bfs(root); return 0; }读一下代码
时间: 2024-03-29 18:42:10 浏览: 134
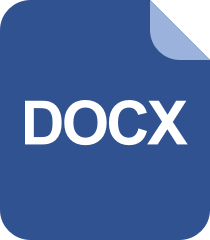
万能头文件#include“bitsstdc++.h”.docx
这是一个二叉树的遍历和操作代码。具体来说,它实现了以下功能:
1. 通过前序遍历序列和中序遍历序列构建二叉树,build函数实现了这个功能。
2. 对二叉树进行广度优先遍历,bfs函数实现了这个功能。
3. 对二叉树进行翻转操作,Swap函数实现了这个功能。
4. 对二叉树进行反转和翻转操作,revswap函数实现了这个功能。
在main函数中,它首先读入了树的节点数n,以及树的前序遍历序列pre和中序遍历序列in。然后通过调用build函数构建了二叉树,接着调用revswap函数进行反转和翻转操作,最后调用bfs函数进行广度优先遍历输出结果。
阅读全文
相关推荐
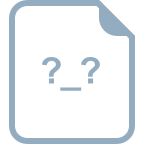




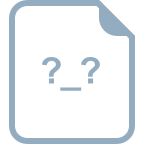
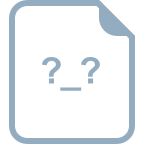
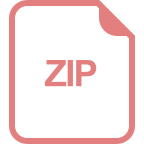
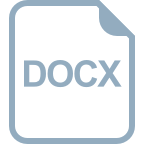
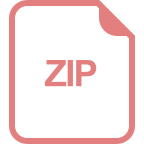
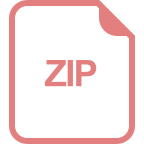
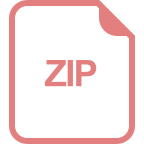
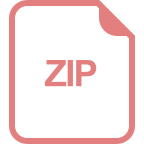
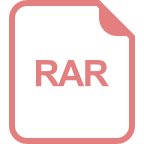
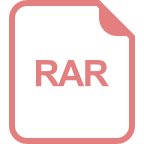
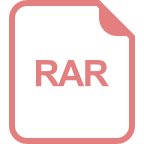