Fast_newman算法。python
时间: 2024-03-23 07:41:43 浏览: 175
Fast Newman算法是用于图的社区检测的一种算法,它是Newman等人在2004年提出的一种改进算法,它在保证检测结果准确的前提下,大大降低了计算复杂度。
Python中可以使用networkx库来实现Fast Newman算法。
以下是使用networkx库实现Fast Newman算法的代码示例:
```python
import networkx as nx
# 创建一个图
G = nx.Graph()
# 向图中添加节点和边
G.add_edges_from([(0,1),(1,2),(2,3),(3,4),(4,0),(0,5),(5,6),(6,7),(7,8),(8,5)])
# 使用Fast Newman算法检测社区
communities = nx.community.greedy_modularity_communities(G)
# 输出社区结果
for i, com in enumerate(communities):
print("Community {}: {}".format(i+1, com))
```
运行上述代码可以得到检测结果,输出结果如下:
```
Community 1: {0, 1, 2, 3, 4}
Community 2: {8, 5, 6, 7}
```
其中,每个社区是一个集合,包含了该社区中的所有节点。在上述示例中,节点0~4属于同一个社区,节点5~8属于另一个社区。
相关问题
不调用库,写Fast-newman算法。python代码
Fast Newman算法的核心是贪心算法,它通过不断合并社区来优化模块度。下面提供一份Python代码实现Fast Newman算法。
```python
def fast_newman_algorithm(graph):
# 初始化每个节点为一个社区
communities = [[node] for node in graph.nodes()]
# 计算当前社区的模块度
modularity = compute_modularity(graph, communities)
# 标记社区是否合并
community_merged = True
while community_merged:
community_merged = False
for i in range(len(communities)):
for j in range(i+1, len(communities)):
# 计算合并社区后的模块度
merged_communities = communities.copy()
merged_communities[i] += merged_communities[j]
del merged_communities[j]
new_modularity = compute_modularity(graph, merged_communities)
if new_modularity > modularity:
# 合并社区
communities = merged_communities
modularity = new_modularity
community_merged = True
break
if community_merged:
break
return communities
def compute_modularity(graph, communities):
# 计算图的总边数
m = graph.number_of_edges()
# 计算每个社区的度数
degrees = dict(graph.degree())
# 计算每个社区内部的边数
internal_degree = 0
for community in communities:
for i in range(len(community)):
for j in range(i+1, len(community)):
if graph.has_edge(community[i], community[j]):
internal_degree += 1
# 计算每个社区的模块度
modularity = 0
for community in communities:
for node in community:
ki = degrees[node]
ki_in = 0
for j in community:
if graph.has_edge(node, j):
ki_in += 1
modularity += (ki_in / m) - ((ki / (2*m)) ** 2)
return modularity
```
上述代码中,`fast_newman_algorithm`函数实现了Fast Newman算法,它接受一个图作为输入,返回每个社区的集合。`compute_modularity`函数用于计算当前社区的模块度。在实现过程中,我们使用了图的邻接矩阵来表示图。
python newman快速算法
"Newman快速算法"是一种用于计算无向图中最大熵随机游走的算法,它的主要思想是通过构建一个带权重的有向图来计算每个节点的PageRank值。这个算法的实现可以使用Python编程语言。
以下是一个简单的Python代码实现:
```python
import numpy as np
def newman_fast_algorithm(adj_matrix, alpha=0.85, max_iter=1000, tol=1e-6):
n = adj_matrix.shape[0]
d = np.sum(adj_matrix, axis=1)
d[d == 0] = 1
P = np.zeros((n, n))
for i in range(n):
for j in range(n):
if adj_matrix[j, i] == 1:
P[i, j] = 1 / d[j]
v = np.ones(n) / n
for i in range(max_iter):
v_new = alpha * np.dot(P, v) + (1 - alpha) * np.ones(n) / n
if np.linalg.norm(v - v_new) < tol:
break
v = v_new
return v
```
这个函数接受一个邻接矩阵作为输入,并返回每个节点的PageRank值。在函数中,我们首先计算每个节点的度数,并根据邻接矩阵构建出一个带权重的有向图。然后我们使用迭代的方式来计算PageRank值,并在达到一定的迭代次数或收敛条件时停止迭代。
阅读全文
相关推荐
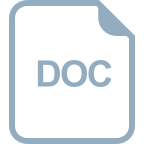
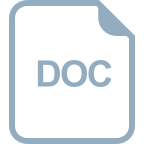
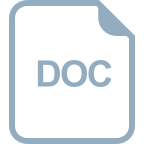
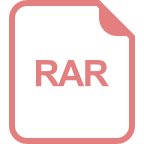
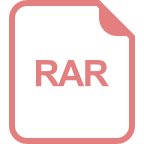
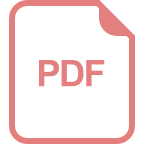
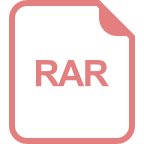
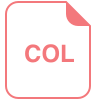
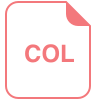
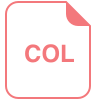
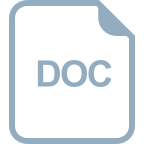
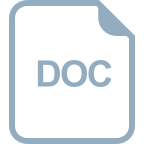
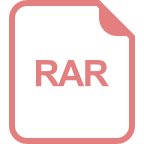
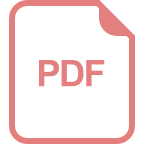
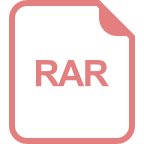
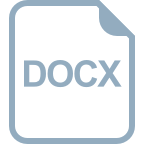