UPDATE alert_tag <set> <if test="area_id != null and area_id != 0 "> area_id = #{area_id}, </if> <if test="tag_name != null and tag_name != '' "> tag_name = #{tag_name}, </if> <if test="tID != null and tID != '' "> tID = #{tID}, </if> <if test="addition != null and addition != '' "> addition = #{addition}, </if> <if test="api_key != null and api_key != '' "> api_key = #{api_key}, </if> <if test="ctime != null and ctime != '' "> ctime = #{ctime}, </if> <if test="alertState != null and alertState != '' "> alertState = #{alertState}, </if> <if test="alertContent != null and alertContent != '' "> alertContent = alertContent+"| closed by"+#{alertContent}, </if> </set> WHERE tID=#{tID} 哪里错误
时间: 2024-03-26 11:42:11 浏览: 13
这段SQL UPDATE语句中存在一个错误,即在 SET 子句中对 alertContent 进行赋值时,使用了加号 "+" 连接 alertContent 和字符串 " closed by" 及其后面的变量 #{alertContent}。这样写会导致语法错误,因为在 SET 子句中不能使用加号运算符。
正确的写法是使用 CONCAT 函数来将 alertContent 和字符串 " closed by" 及其后面的变量 #{alertContent} 进行连接,如下所示:
```
UPDATE alert_tag
SET
area_id = #{area_id},
tag_name = #{tag_name},
tID = #{tID},
addition = #{addition},
api_key = #{api_key},
ctime = #{ctime},
alertState = #{alertState},
alertContent = CONCAT(alertContent, ' closed by', #{alertContent})
WHERE tID = #{tID}
```
这样就能正确地将 alertContent 和字符串 " closed by" 及其后面的变量 #{alertContent} 进行连接了。
相关问题
优化语句<update id="updateByPrimaryKey" parameterType="com.example.demo.domain.Permission"> update sys_permission <set> <if test="permission_id!=null"> permission_id = #{permission_id}, </if> <if test="pid!=null"> pid = #{pid}, </if> <if test="name!=null"> name = #{name}, </if> <if test="type!=null"> type = #{type}, </if> <if test="permission_value!=null"> permission_value = #{permission_value}, </if> <if test="uri!=null"> uri = #{uri}, </if> <if test="icon!=null"> icon = #{icon}, </if> <if test="status!=null"> status = #{status}, </if> <if test="ctime!=null"> ctime = #{ctime}, </if> <if test="orders!=null"> orders = #{orders}, </if> <if test="system_id!=null"> system_id = #{system_id} </if> </set> where permission_id = #{permission_id} </update>
这段MyBatis XML中的SQL语句看起来已经比较优化了,但还有一些可以改进的地方。下面是一些建议:
1. 使用`<trim>`标签:在`<set>`标签中,每个`<if>`标签都会生成一个逗号,最后一个`<if>`标签需要特殊处理。可以使用`<trim>`标签来解决这个问题,示例代码如下:
```xml
<set>
<trim suffixOverrides="," suffix=" ">
<if test="permission_id!=null">
permission_id = #{permission_id},
</if>
<if test="pid!=null">
pid = #{pid},
</if>
<!-- 其他属性 -->
<if test="system_id!=null">
system_id = #{system_id}
</if>
</trim>
</set>
```
上面的代码中,使用了`suffixOverrides`属性来删除每个`<if>`标签生成的逗号。同时,使用了`suffix`属性来在最后一个属性后面添加一个空格。
2. 使用`<foreach>`标签:如果要更新的字段比较多,可以使用`<foreach>`标签来生成`<if>`标签,减少重复代码。示例代码如下:
```xml
<set>
<foreach collection="updateFields" item="field">
<if test="field=='permission_id'">
permission_id = #{permission_id},
</if>
<if test="field=='pid'">
pid = #{pid},
</if>
<!-- 其他属性 -->
<if test="field=='system_id'">
system_id = #{system_id}
</if>
</foreach>
</set>
```
上面的代码中,`updateFields`是一个`List<String>`类型的变量,存储需要更新的字段名。使用`<foreach>`标签遍历`updateFields`,生成对应的`<if>`标签。
3. 使用动态SQL:如果更新的条件和更新的字段都不确定,可以使用动态SQL来生成SQL语句。示例代码如下:
```xml
<update id="updatePermission" parameterType="Map">
UPDATE sys_permission
<set>
<foreach collection="updateFields" item="field">
${field} = #{${field}},
</foreach>
</set>
<where>
1=1
<foreach collection="conditions" item="condition" separator="AND">
${condition.field} ${condition.operator} #{condition.value}
</foreach>
</where>
</update>
```
上面的代码中,`updateFields`和`conditions`都是存储在`Map`中的变量,分别表示需要更新的字段和更新的条件。使用`${}`语法来插入动态SQL。
为什么以下代码会导致上述错误 <select id="selectList" resultMap="BaseResultMap" parameterType="com.wego.entity.query.CartItemQuery"> select <include refid="Base_Column_List"/> from tb_cart_item <where> <if test="id != null"> and id = #{id} </if> <if test="goodsId != null"> and goodsId= #{goodsId} </if> <if test="amount != null"> and amount= #{amount} </if> <if test="money != null"> and money= #{money} </if> </where> order by update_time desc </select>
根据你提供的代码片段,错误可能出现在以下位置:
```xml
<if test="id != null">
and id = #{id}
</if>
```
```xml
<if test="goodsId != null">
and goodsId = #{goodsId}
</if>
```
```xml
<if test="amount != null">
and amount = #{amount}
</if>
```
```xml
<if test="money != null">
and money = #{money}
</if>
```
这些 `<if>` 标签用于根据条件动态生成 SQL 查询。然而,在生成的 SQL 查询中,如果这些条件都不满足(即对应的参数为 null),那么会导致生成的 SQL 查询中存在多余的 "and" 关键字,从而导致 SQL 语法错误。
为了解决这个问题,你可以在每个 `<if>` 标签的末尾添加一个空格,以确保在条件不满足时生成的 SQL 查询仍然是有效的。例如:
```xml
<if test="id != null">
and id = #{id}
</if>
```
改为:
```xml
<if test="id != null">
and id = #{id}
</if>
```
通过这样的修改,生成的 SQL 查询将不会存在多余的 "and" 关键字,从而避免了 SQL 语法错误。
相关推荐
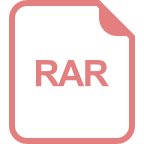
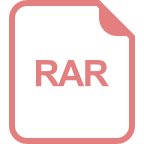
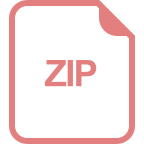













