c#写一个拼图小游戏
时间: 2023-08-02 07:24:34 浏览: 54
好的,我可以为您提供一个简单的拼图小游戏的代码示例。这个示例使用Windows Forms创建游戏界面,并使用C#编写游戏逻辑,实现游戏的运行和控制。这个示例游戏包含3个难度级别,玩家需要将一张图片切成若干小块,然后重新拼成完整的图片。
以下是代码示例:
```c#
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Windows.Forms;
namespace PuzzleGame
{
public partial class MainForm : Form
{
private const int EasyLevel = 3;
private const int MediumLevel = 4;
private const int HardLevel = 5;
private int _level;
private int _blockSize;
private int _emptyIndex;
private int[] _indexes;
private Image _sourceImage;
private List<Image> _blocks;
private Random _rand;
public MainForm()
{
InitializeComponent();
_rand = new Random();
}
private void MainForm_Load(object sender, EventArgs e)
{
_sourceImage = Properties.Resources.puzzle_image;
cbLevel.SelectedIndex = 0;
StartGame();
}
private void cbLevel_SelectedIndexChanged(object sender, EventArgs e)
{
StartGame();
}
private void StartGame()
{
_level = cbLevel.SelectedIndex switch
{
0 => EasyLevel,
1 => MediumLevel,
2 => HardLevel,
_ => EasyLevel,
};
_blockSize = _sourceImage.Width / _level;
_indexes = new int[_level * _level];
_emptyIndex = _level * _level - 1;
_blocks = new List<Image>();
for (int i = 0; i < _level * _level; i++)
{
if (i == _emptyIndex)
{
_blocks.Add(null);
}
else
{
int x = i % _level;
int y = i / _level;
Bitmap bitmap = new Bitmap(_blockSize, _blockSize);
Graphics graphics = Graphics.FromImage(bitmap);
graphics.DrawImage(_sourceImage, new Rectangle(0, 0, _blockSize, _blockSize), new Rectangle(x * _blockSize, y * _blockSize, _blockSize, _blockSize), GraphicsUnit.Pixel);
_blocks.Add(bitmap);
_indexes[i] = i;
}
}
Shuffle();
DrawBlocks();
}
private void Shuffle()
{
for (int i = 0; i < _level * _level; i++)
{
int j = _rand.Next(i, _level * _level);
int temp = _indexes[i];
_indexes[i] = _indexes[j];
_indexes[j] = temp;
}
}
private void DrawBlocks()
{
panel.Controls.Clear();
for (int i = 0; i < _level * _level; i++)
{
int x = i % _level;
int y = i / _level;
PictureBox pictureBox = new PictureBox
{
Size = new Size(_blockSize, _blockSize),
Location = new Point(x * _blockSize, y * _blockSize),
Image = _blocks[_indexes[i]],
Tag = i,
};
pictureBox.Click += PictureBox_Click;
panel.Controls.Add(pictureBox);
}
}
private void PictureBox_Click(object sender, EventArgs e)
{
int index = (int)((PictureBox)sender).Tag;
if (index % _level > 0 && _indexes[index - 1] == _emptyIndex)
{
Swap(index, index - 1);
}
else if (index % _level < _level - 1 && _indexes[index + 1] == _emptyIndex)
{
Swap(index, index + 1);
}
else if (index / _level > 0 && _indexes[index - _level] == _emptyIndex)
{
Swap(index, index - _level);
}
else if (index / _level < _level - 1 && _indexes[index + _level] == _emptyIndex)
{
Swap(index, index + _level);
}
CheckWin();
}
private void Swap(int i, int j)
{
int temp = _indexes[i];
_indexes[i] = _indexes[j];
_indexes[j] = temp;
((PictureBox)panel.Controls[i]).Image = _blocks[_indexes[i]];
((PictureBox)panel.Controls[j]).Image = _blocks[_indexes[j]];
}
private void CheckWin()
{
for (int i = 0; i < _level * _level; i++)
{
if (_indexes[i] != i)
{
return;
}
}
MessageBox.Show("You Win!");
StartGame();
}
}
}
```
主要实现了以下功能:
1. 加载游戏界面和图片资源。
2. 实现了拼图游戏的难度选择功能。
3. 初始化游戏数据,包括随机打散拼图块的顺序。
4. 绘制拼图块。
5. 实现了玩家点击拼图块的交换功能。
6. 检查玩家是否完成了拼图任务。
请注意,这只是一个基本的示例,您可以根据自己的需要添加更多的功能和细节,以使游戏更加有趣。
相关推荐
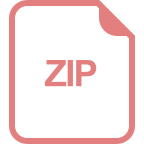














