python 调用路径带空格的文件
时间: 2023-06-15 10:03:24 浏览: 119
在 Python 中调用路径带空格的文件,可以使用双引号将路径括起来,例如:
```python
path_with_space = "C:/Program Files/My Folder/myfile.txt"
with open(path_with_space) as f:
# do something with the file
```
另外,也可以在路径中使用反斜杠来转义空格,例如:
```python
path_with_space = "C:/Program\ Files/My\ Folder/myfile.txt"
with open(path_with_space) as f:
# do something with the file
```
以上两种方法都可以成功调用路径中带空格的文件。
相关问题
如何在mach3调用python文件运行
要在Mach3中调用Python文件运行,请按照以下步骤操作:
1. 确保安装了Python和Mach3。
2. 打开Mach3,进入“Config”菜单下的“Ports and Pins”。
3. 在“Input Signals”选项卡下找到“Mcode”选项,勾选“Enabled”。
4. 在“Output Signals”选项卡下找到“Output#1”选项,勾选“Enabled”。
5. 保存设置并重启Mach3。
6. 在Python中编写需要运行的代码,并将其保存为.py文件。
7. 在Mach3中打开G代码编辑器,输入以下代码:
M1001 P1
8. 将代码中的“P1”替换为你的Python文件的路径和文件名(例如,“C:\Program Files\Python36\test.py”)。
9. 保存并运行G代码,Mach3会自动调用Python文件并执行其中的代码。
注意事项:
- 确保Python文件的路径和文件名不包含空格或特殊字符。
- 在Python文件中不要使用input()函数,因为它会阻塞Mach3的运行。
- 调用Python文件时,Mach3会暂停所有运动,直到Python代码执行完毕。因此,确保Python代码的执行时间不会过长,以免影响加工效率。
python调用可执行文件怎样读取return值
要使用Python调用可执行文件并获取其返回值,可以使用`subprocess`模块中的`run()`函数。下面是使用示例代码:
```python
import subprocess
result = subprocess.run(['path/to/exe', 'arg1', 'arg2'], capture_output=True)
output = result.stdout.decode('utf-8').strip()
return_code = result.returncode
if return_code == 0:
print('Success:', output)
else:
print('Error:', output)
```
在示例代码中,`['path/to/exe', 'arg1', 'arg2']`表示可执行文件路径和参数列表,`capture_output=True`表示捕获子进程的输出。
`result.stdout`包含子进程输出的原始字节串,需要使用`.decode('utf-8')`将其转换为字符串。`.strip()`方法用于去除字符串前后的空格和换行符。
`result.returncode`包含子进程的返回值。一般来说,返回值为0表示成功,非0值表示失败。
相关推荐
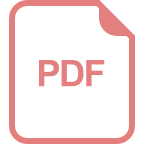
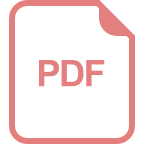
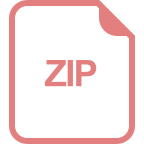












