python实现基于前馈神经网络的数据预测
时间: 2023-12-10 14:41:59 浏览: 43
要实现基于前馈神经网络的数据预测,可以按照以下步骤进行:
1. 准备数据集:将需要进行预测的数据整理成一个数据集,包括输入数据和对应的输出数据。
2. 数据预处理:将数据集进行预处理,包括数据归一化、数据标准化、数据降维等处理方式。
3. 构建神经网络模型:选择合适的神经网络结构,设置模型的超参数,如学习率、迭代次数、批量大小等。
4. 训练模型:使用给定的训练数据集对神经网络进行训练,通过反向传播算法来更新模型中的权重和偏置。
5. 验证模型:使用验证数据集对训练好的模型进行验证,评估模型的性能和泛化能力。
6. 使用模型进行预测:使用训练好的模型对新数据进行预测,得到预测结果。
下面是一个简单的代码示例,实现基于前馈神经网络的数据预测:
```python
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.neural_network import MLPRegressor
# 准备数据集
X = np.array([[1, 2, 3], [2, 4, 6], [3, 6, 9], [4, 8, 12], [5, 10, 15]])
y = np.array([2, 4, 6, 8, 10])
# 数据预处理
scaler = StandardScaler()
X = scaler.fit_transform(X)
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 构建神经网络模型
model = MLPRegressor(hidden_layer_sizes=(100, 50), activation='relu', solver='adam', max_iter=1000, learning_rate_init=0.01)
# 训练模型
model.fit(X_train, y_train)
# 验证模型
score = model.score(X_test, y_test)
print('模型得分:', score)
# 使用模型进行预测
X_new = np.array([[6, 12, 18]])
X_new = scaler.transform(X_new)
y_pred = model.predict(X_new)
print('预测结果:', y_pred)
```
在这个示例中,我们使用了 `MLPRegressor` 类来构建前馈神经网络模型,使用了 `StandardScaler` 类来进行数据标准化处理。在训练好模型后,我们使用 `score` 方法来评估模型的性能,使用 `predict` 方法来进行新数据的预测。
相关推荐
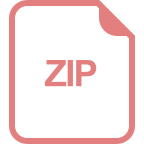
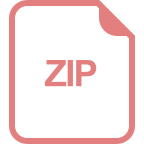














