用c语言实现music
时间: 2023-11-24 16:06:33 浏览: 152
实现音乐可以使用C语言的音频库,例如ALSA、PortAudio、SDL等库,这些库都可以在C语言中使用。你可以使用这些库来读取音频文件,将音频数据解码,并将其发送到音频设备进行播放。以下是使用ALSA库实现播放音乐的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
int main(int argc, char *argv[]) {
// 打开默认的PCM设备
snd_pcm_t *pcm_handle;
int err = snd_pcm_open(&pcm_handle, "default", SND_PCM_STREAM_PLAYBACK, 0);
if (err < 0) {
printf("Unable to open PCM device: %s\n", snd_strerror(err));
return 1;
}
// 配置PCM参数
snd_pcm_hw_params_t *params;
snd_pcm_hw_params_alloca(¶ms);
snd_pcm_hw_params_any(pcm_handle, params);
snd_pcm_hw_params_set_access(pcm_handle, params, SND_PCM_ACCESS_RW_INTERLEAVED);
snd_pcm_hw_params_set_format(pcm_handle, params, SND_PCM_FORMAT_S16_LE);
snd_pcm_hw_params_set_channels(pcm_handle, params, 2);
unsigned int rate = 44100;
snd_pcm_hw_params_set_rate_near(pcm_handle, params, &rate, 0);
err = snd_pcm_hw_params(pcm_handle, params);
if (err < 0) {
printf("Unable to configure PCM device: %s\n", snd_strerror(err));
return 1;
}
// 打开音频文件
FILE *fp = fopen(argv[1], "rb");
if (!fp) {
printf("Unable to open file: %s\n", argv[1]);
return 1;
}
// 读取音频数据并写入PCM设备
char buf[1024];
int read_count;
while ((read_count = fread(buf, 1, sizeof(buf), fp)) > 0) {
err = snd_pcm_writei(pcm_handle, buf, read_count / 4);
if (err < 0) {
printf("Unable to write PCM device: %s\n", snd_strerror(err));
break;
}
}
// 关闭音频文件和PCM设备
fclose(fp);
snd_pcm_close(pcm_handle);
return 0;
}
```
你可以将音频文件作为命令行参数传递给该程序,例如:
```
$ ./play_music music.wav
```
阅读全文
相关推荐
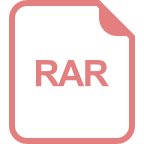
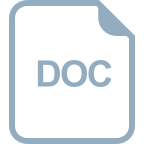
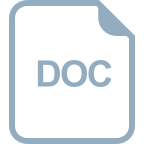

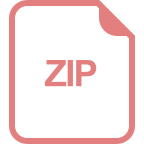
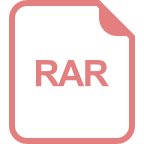
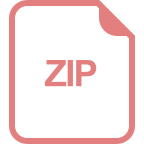
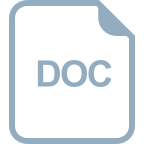
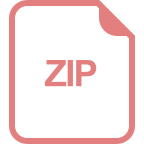
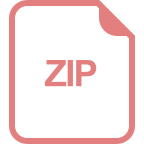
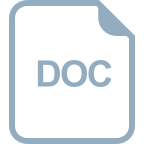
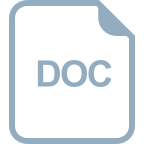
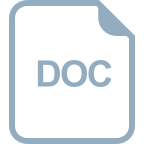
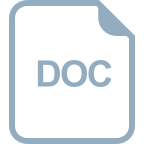

