运用指针在字符串中插入字符串
时间: 2023-09-24 09:09:25 浏览: 90
在字符串中插入字符串需要进行动态内存分配,可以按照以下步骤进行:
1. 计算插入字符串的长度和原字符串的长度,得到新字符串的长度。
2. 申请新字符串的内存空间,大小为新字符串的长度加上1(用于存储字符串结束符'\0')。
3. 将原字符串的前半部分复制到新字符串中。
4. 将插入字符串复制到新字符串的对应位置。
5. 将原字符串的后半部分复制到新字符串中。
6. 释放原字符串的内存空间。
7. 返回新字符串的指针。
以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char* insertString(char* str1, char* str2, int pos) {
int len1 = strlen(str1);
int len2 = strlen(str2);
int newLen = len1 + len2;
char* newStr = (char*)malloc(sizeof(char) * (newLen + 1)); // 加1用于存储字符串结束符'\0'
if (newStr == NULL) {
printf("Error: memory allocation failed.\n");
exit(1);
}
int i;
for (i = 0; i < pos; i++) {
newStr[i] = str1[i];
}
for (i = 0; i < len2; i++) {
newStr[pos + i] = str2[i];
}
for (i = pos; i < len1; i++) {
newStr[i + len2] = str1[i];
}
newStr[newLen] = '\0';
free(str1);
return newStr;
}
int main() {
char str1[] = "Hello, world!";
char str2[] = "beautiful ";
char* newStr = insertString(str1, str2, 7);
printf("%s\n", newStr); // 输出 "Hello, beautiful world!"
free(newStr);
return 0;
}
```
阅读全文
相关推荐
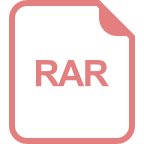
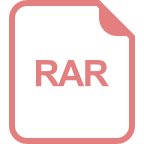
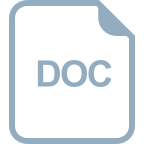
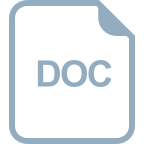
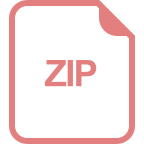
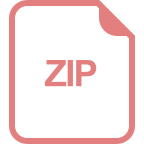
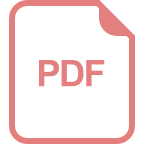
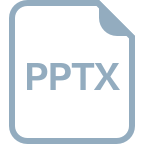
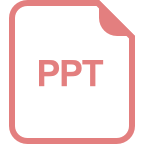
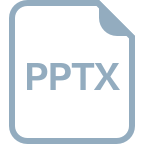
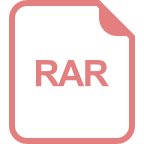
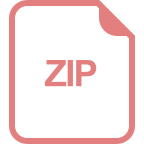
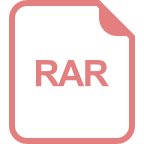
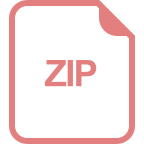
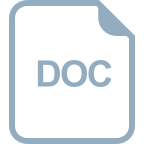
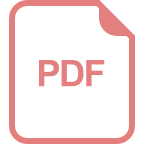