一、实验目的 运用BERT预训练模型实现抽取式阅读理解。 二、实验要求 1、理解BERT抽取式阅读理解的模型框架及原理; 2、代码实现BERT抽取式阅读理解
时间: 2023-08-02 08:06:50 浏览: 117
的模型,并在特定的数据集上进行训练和测试; 3、根据实验结果分析模型的性能和优缺点。 三、实验步骤 1、准备数据集:选择适合的数据集,例如SQuAD数据集; 2、预处理数据集:将数据集转化为模型可处理的格式,包括将文本转化为数字表示、对答案进行标注等; 3、构建BERT模型:使用预训练的BERT模型或自己训练的BERT模型,加入适当的层和参数,构建适合阅读理解任务的模型; 4、训练模型:利用训练集对模型进行训练,不断调整模型的参数和超参数,以达到最佳的性能; 5、测试模型:使用测试集对训练好的模型进行测试,评估模型的性能和精度; 6、分析结果:根据实验结果分析模型的性能和优缺点,进一步改进模型。 四、实验工具和环境 实验工具:Python、PyTorch、Hugging Face Transformers 环境要求:Python 3.x、PyTorch 1.x、Hugging Face Transformers库。
相关问题
代码实现BERT抽取式阅读理解
BERT模型在自然语言处理领域中有着广泛的应用,其中之一就是抽取式阅读理解。本文将介绍如何使用BERT模型实现抽取式阅读理解任务。
抽取式阅读理解任务是指给定一篇文章和相关问题,需要从文章中找出与问题相关的答案。这种任务在问答系统、机器翻译等领域中有着广泛的应用。
下面是使用BERT模型实现抽取式阅读理解的步骤:
1. 数据预处理
首先需要对数据进行预处理,将文章和问题转换成BERT模型的输入格式。BERT模型的输入是一组token序列,其中每个token都对应一个编号。因此需要将文章和问题中的每个词转换成对应的编号。同时,还需要加入一些特殊的token,如[CLS]表示序列的开始,[SEP]表示序列的结束。
2. BERT模型
接下来需要使用BERT模型对输入序列进行编码。BERT模型使用Transformer结构对输入序列进行编码,得到一个表示序列的向量。可以使用预训练好的BERT模型,也可以使用自己的数据进行微调。
3. 输出层
得到编码后的序列向量后,需要使用输出层将其转换成答案的开始和结束位置。输出层通常由两个全连接层组成,其中第一个全连接层将编码后的序列向量转换成一个表示开始位置的向量,第二个全连接层将其转换成一个表示结束位置的向量。
4. 训练和预测
最后需要对模型进行训练和预测。在训练时,可以使用交叉熵损失函数来计算模型的损失,然后使用反向传播算法更新模型的参数。在预测时,需要使用模型预测出答案的开始和结束位置,并从原始输入序列中抽取出答案。
下面是使用PyTorch实现BERT抽取式阅读理解的代码示例:
```python
import torch
from transformers import BertTokenizer, BertForQuestionAnswering
# 加载预训练的BERT模型和tokenizer
model = BertForQuestionAnswering.from_pretrained('bert-base-chinese')
tokenizer = BertTokenizer.from_pretrained('bert-base-chinese')
# 文章和问题
context = '自然语言处理是人工智能和语言学领域的交叉学科,旨在研究计算机如何处理和理解自然语言。'
question = '自然语言处理属于哪个学科?'
# 将文章和问题转换成token序列
tokens = tokenizer.encode_plus(question, context, add_special_tokens=True, return_tensors='pt')
# 使用BERT模型得到编码后的序列向量
outputs = model(**tokens)
# 将序列向量转换成答案的开始和结束位置
start_logits, end_logits = outputs.start_logits, outputs.end_logits
# 从原始输入序列中抽取出答案
start_index = torch.argmax(start_logits)
end_index = torch.argmax(end_logits)
answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(tokens['input_ids'][0][start_index:end_index+1]))
print(answer)
```
在上面的代码中,首先加载了预训练的BERT模型和tokenizer。然后将文章和问题转换成token序列,并使用BERT模型得到编码后的序列向量。最后将序列向量转换成答案的开始和结束位置,并从原始输入序列中抽取出答案。
bert预训练模型抽取文本特征
BERT(Bidirectional Encoder Representations from Transformers)是一种预训练模型,可以用于文本特征提取。BERT模型通过在大规模文本数据上进行无监督的预训练来学习通用的文本表示,在此基础上可以进行各种下游任务的微调或特征提取。
要使用BERT模型抽取文本特征,首先需要将输入的文本转换为BERT所需的格式。对于英文文本,可以使用WordPiece标记化方法将文本拆分为词片段,并添加特殊的标记(如[CLS]和[SEP])来表示句子的开始和结束。对于中文文本,可以使用字级别的标记化方法。
然后,将标记化后的文本输入到BERT模型中,获取模型的隐藏状态。BERT模型通常有多层Transformer编码器,每层都会输出相应的隐藏状态。可以选择使用最后一层的隐藏状态,或者将多个层的隐藏状态进行融合,得到最终的文本特征表示。
一种常用的方法是将最后一层的隐藏状态与特定位置的标记(如[CLS])进行连接,得到表示整个句子的特征向量。这个特征向量可以用作文本分类、句子相似度计算等下游任务的输入。
除了最后一层的隐藏状态,BERT模型还可以提供其他层的隐藏状态,这些隐藏状态可以更细粒度地表示文本的各个方面。可以根据具体的任务需求选择相应的隐藏状态进行特征提取。
需要注意的是,由于BERT模型参数较多,对大规模文本数据进行完整的特征提取可能会比较耗时。为了提高效率,可以使用批量化技术对多个文本样本进行并行处理,或者使用模型压缩等方法减少模型的大小和计算量。
相关推荐
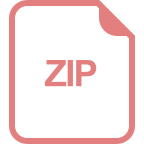
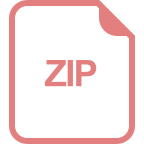
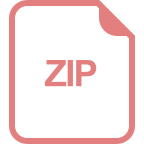












