刷新的时候可以操作系统其他功能
时间: 2024-03-18 18:39:10 浏览: 73
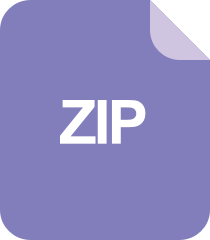
易语言刷新系统托盘
当使用动态实时更新时间的程序时,如果需要在刷新时间的同时进行其他操作,可以使用多线程来实现。例如,在上面的示例程序中,可以创建一个新的线程来处理用户输入,并在主线程中调用 update_time() 函数来实现实时更新时间的功能。以下是一个示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <unistd.h>
#include <pthread.h>
#define BUFFER_SIZE 1024
void *input_thread(void *arg)
{
while (1) {
char buffer[BUFFER_SIZE];
printf("Enter a command: ");
fgets(buffer, BUFFER_SIZE, stdin);
// 处理用户输入
// ...
// 输出提示信息
printf("Command: %s\n", buffer);
}
return NULL;
}
void *update_time_thread(void *arg)
{
while (1) {
time_t now = time(NULL);
char *time_str = ctime(&now);
// 删除字符串末尾的换行符
time_str[strlen(time_str) - 1] = '\0';
// 清空屏幕
system("clear");
// 输出时间
printf("Current time: %s\n", time_str);
// 等待一秒钟
sleep(1);
}
return NULL;
}
int main()
{
// 创建一个线程来更新时间
pthread_t update_thread;
int ret = pthread_create(&update_thread, NULL, update_time_thread, NULL);
if (ret != 0) {
printf("Error: %d\n", ret);
return 1;
}
// 创建一个线程来处理用户输入
pthread_t input_thread;
ret = pthread_create(&input_thread, NULL, input_thread, NULL);
if (ret != 0) {
printf("Error: %d\n", ret);
return 1;
}
// 等待两个线程结束
pthread_join(update_thread, NULL);
pthread_join(input_thread, NULL);
return 0;
}
```
该程序创建了两个线程,一个用于实时更新时间,另一个用于处理用户输入。在主线程中,等待两个线程结束。使用多线程可以同时进行多项任务,提高了程序的效率和灵活性。
阅读全文
相关推荐
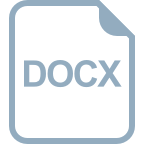
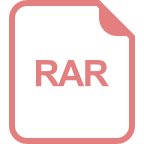
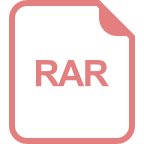
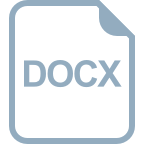
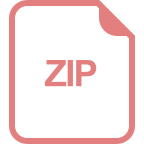
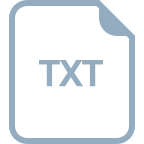
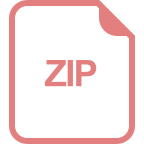
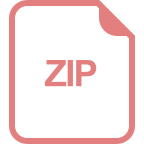
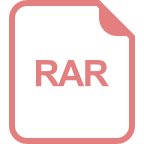
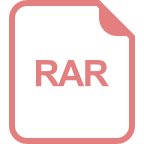
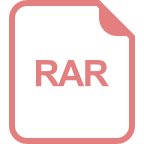
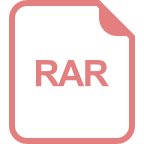
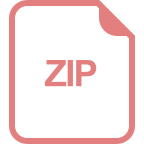
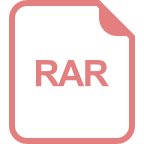
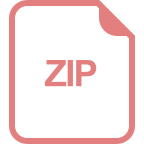
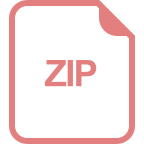
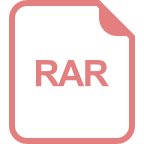
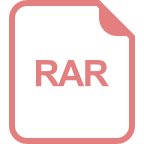