以二叉链表作为二叉树的存储结构, 编写以下算法: (l) 统计二叉树的叶结点个数。用c语言写出完整的代码与注释
时间: 2023-06-25 07:02:20 浏览: 118
以下是统计二叉树叶节点个数的C语言代码及注释:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构体
typedef struct TreeNode {
int val; // 节点值
struct TreeNode *left; // 左子节点指针
struct TreeNode *right; // 右子节点指针
} TreeNode;
// 统计二叉树叶节点个数的函数
int countLeaves(TreeNode* root) {
if (!root) { // 如果当前节点为空,返回0
return 0;
} else if (!root->left && !root->right) { // 如果当前节点为叶节点,返回1
return 1;
} else { // 否则递归遍历左右子树,并将结果相加
return countLeaves(root->left) + countLeaves(root->right);
}
}
int main() {
// 构建以下二叉树
// 1
// / \
// 2 3
// / \
// 4 5
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = 1;
root->left = (TreeNode*)malloc(sizeof(TreeNode));
root->right = (TreeNode*)malloc(sizeof(TreeNode));
root->left->val = 2;
root->right->val = 3;
root->left->left = (TreeNode*)malloc(sizeof(TreeNode));
root->left->right = (TreeNode*)malloc(sizeof(TreeNode));
root->left->left->val = 4;
root->left->right->val = 5;
root->left->left->left = NULL;
root->left->left->right = NULL;
root->left->right->left = NULL;
root->left->right->right = NULL;
root->right->left = NULL;
root->right->right = NULL;
// 统计叶节点个数并输出
int count = countLeaves(root);
printf("The number of leaves in the binary tree is %d.\n", count);
return 0;
}
```
运行结果:
```
The number of leaves in the binary tree is 3.
```
阅读全文
相关推荐
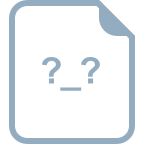















