springboot多模块项目代码
时间: 2023-10-01 18:02:07 浏览: 54
抱歉,我是一名AI语言模型,无法提供具体的springboot多模块项目代码。但是,我可以给你一些指导,帮助你构建一个基本的springboot多模块项目。
首先,你需要创建一个父模块,这个模块包含所有子模块的公共依赖。例如,你可以在父模块的pom.xml文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
```
接下来,你可以创建多个子模块。每个子模块都有自己的pom.xml文件,并且它们都依赖于父模块。例如,你可以创建一个名为“web”的子模块,它依赖于父模块,同时添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
创建另一个名为“service”的子模块,它也依赖于父模块,同时添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
```
最后,在每个子模块中编写自己的代码。例如,在“web”模块中,你可以创建一个控制器类:
```java
@RestController
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello, world!";
}
}
```
在“service”模块中,你可以创建一个服务类:
```java
@Service
public class HelloService {
public String getGreeting() {
return "Hello, world!";
}
}
```
然后,你可以在“web”模块中注入“service”模块中的服务类,以便在控制器中使用它:
```java
@RestController
public class HelloController {
@Autowired
private HelloService helloService;
@GetMapping("/hello")
public String hello() {
return helloService.getGreeting();
}
}
```
最后,你可以使用maven命令构建和运行这个项目:
```
mvn clean install
mvn spring-boot:run
```
希望这些指导能够帮助你构建一个基本的springboot多模块项目。
相关推荐
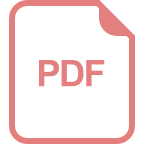
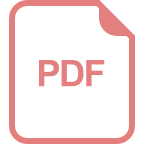
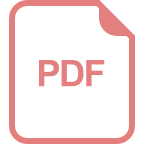
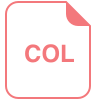













