(1)在aspx页面中使用标签控件、文本控件、按钮控件、列表控件、图片控件、文件上传控件、验证控件 。 (2)给每个控件制定校验规则并在提交表单时进行校验,校验通过后才可提交到后台,后台接收到表单数据后,把数据打印到控制台(文件控件不需要打印)。
时间: 2023-12-14 21:38:00 浏览: 95
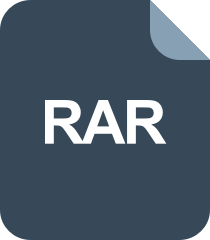
aspx控件

好的,下面是一个简单的示例代码,实现了上述要求:
```
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="WebApplication1.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="lblName" runat="server" Text="姓名:"></asp:Label>
<asp:TextBox ID="txtName" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator ID="rfvName" runat="server" ControlToValidate="txtName"
ErrorMessage="请输入姓名" ValidationGroup="vgSubmit"></asp:RequiredFieldValidator>
</div>
<div>
<asp:Label ID="lblAge" runat="server" Text="年龄:"></asp:Label>
<asp:TextBox ID="txtAge" runat="server"></asp:TextBox>
<asp:RangeValidator ID="rvAge" runat="server" ControlToValidate="txtAge" Type="Integer"
MinimumValue="0" MaximumValue="150" ErrorMessage="请输入正确的年龄" ValidationGroup="vgSubmit"></asp:RangeValidator>
</div>
<div>
<asp:Label ID="lblGender" runat="server" Text="性别:"></asp:Label>
<asp:RadioButtonList ID="rblGender" runat="server">
<asp:ListItem Text="男" Value="M"></asp:ListItem>
<asp:ListItem Text="女" Value="F"></asp:ListItem>
</asp:RadioButtonList>
</div>
<div>
<asp:Label ID="lblHobbies" runat="server" Text="爱好:"></asp:Label>
<asp:CheckBoxList ID="cblHobbies" runat="server">
<asp:ListItem Text="游泳" Value="swimming"></asp:ListItem>
<asp:ListItem Text="跑步" Value="running"></asp:ListItem>
<asp:ListItem Text="阅读" Value="reading"></asp:ListItem>
</asp:CheckBoxList>
</div>
<div>
<asp:Label ID="lblAvatar" runat="server" Text="头像:"></asp:Label>
<asp:FileUpload ID="fupAvatar" runat="server"></asp:FileUpload>
</div>
<div>
<asp:Button ID="btnSubmit" runat="server" Text="提交" ValidationGroup="vgSubmit" OnClick="btnSubmit_Click" />
</div>
</form>
</body>
</html>
```
在以上代码中,我们使用了标签控件(Label)、文本控件(TextBox)、按钮控件(Button)、列表控件(RadioButtonList、CheckBoxList)和文件上传控件(FileUpload),并为每个控件都添加了校验规则(如必填规则、年龄范围规则等)。
在提交按钮上,我们添加了一个 ValidationGroup 属性,用于指定进行校验时所使用的校验组。在单击提交按钮时,会触发 btnSubmit_Click 事件,我们在这个事件中获取表单数据,并将其打印到控制台:
```
protected void btnSubmit_Click(object sender, EventArgs e)
{
if (Page.IsValid)
{
string name = txtName.Text;
int age = Convert.ToInt32(txtAge.Text);
string gender = rblGender.SelectedValue;
string hobbies = string.Join(",", cblHobbies.Items.Cast<ListItem>().Where(item => item.Selected).Select(item => item.Value));
Console.WriteLine("姓名:{0}", name);
Console.WriteLine("年龄:{0}", age);
Console.WriteLine("性别:{0}", gender);
Console.WriteLine("爱好:{0}", hobbies);
}
}
```
在提交事件中,我们首先使用 Page.IsValid 属性判断是否通过校验,如果通过校验,则获取表单数据,并将其打印到控制台。
阅读全文
相关推荐
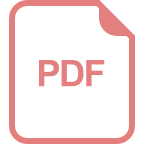
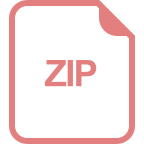

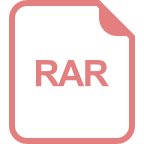
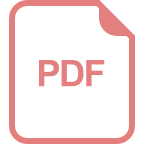

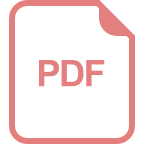
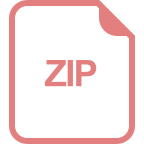
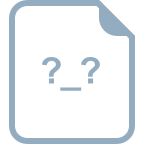
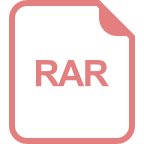
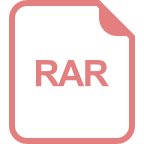
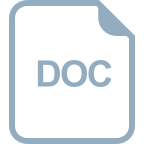
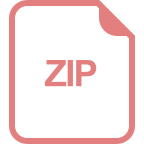