echarts词云图设置背景的代码
时间: 2023-09-29 15:09:32 浏览: 178
以下是一个示例代码,展示如何在 ECharts 中设置词云图的背景:
```
// 引入 ECharts
import echarts from 'echarts';
// 初始化 ECharts 容器
const chartContainer = document.getElementById('myChart');
const chart = echarts.init(chartContainer);
// 设置 ECharts 配置项
const option = {
backgroundColor: '#f5f5f5', // 设置背景颜色
series: [{
type: 'wordCloud',
// 其他配置项
}]
};
// 绘制图表
chart.setOption(option);
// 设置容器样式,其中 bg.jpg 为背景图片地址
chartContainer.style.backgroundImage = 'url(bg.jpg)';
chartContainer.style.backgroundSize = 'cover';
```
在该示例代码中,我们通过设置 backgroundColor 属性和容器样式来设置词云图的背景。当然,你也可以只采用其中一种方式来设置背景,具体取决于你的需求。
相关问题
echarts词云图自定义形状
要在Echarts中使用自定义形状的词云图,需要使用Echarts提供的echarts-wordcloud插件。首先,需要在HTML中引入echarts和echarts-wordcloud插件的JS文件。然后在JS代码中定义词云图的数据和配置项,其中需要指定shape字段为'image',并在style字段中设置image字段为自定义形状的图片地址。例如:
```javascript
option = {
series: [{
type: 'wordCloud',
shape: 'image',
style: {
image: 'your-image-url.png'
},
data: [
{ name: 'word1', value: 100 },
{ name: 'word2', value: 50 },
{ name: 'word3', value: 80 },
// more data items
]
}]
};
```
通过以上配置,即可在Echarts中实现自定义形状的词云图。需要注意的是,自定义形状的图片应该是透明背景的PNG格式图片,且图片大小应该与词云图的大小相匹配。
echarts的词云给每个词添加背景图
Echarts 词云(WordCloud)默认不支持给每个词添加背景图,但可以通过以下方法实现:
1. 在词云中设置每个词的样式(包括颜色、字体大小等),并将每个词的位置和大小信息保存下来。
2. 在词云外部创建一个容器(例如 div 元素),并设置该容器的背景图。
3. 在容器中使用绝对定位(position: absolute)将每个词放置到对应的位置上,并设置每个词的 z-index 属性,使其位于背景图之上。
4. 可以使用 CSS3 的 clip-path 属性将每个词截取成对应的形状,以达到更好的视觉效果。
以下是一个实现的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Echarts 词云添加背景图</title>
<script src="https://cdn.jsdelivr.net/npm/echarts/dist/echarts.min.js"></script>
</head>
<body>
<div id="container" style="position: relative; width: 800px; height: 600px; background-image: url(background.jpg)">
<div id="wordcloud" style="position: absolute; top: 0; left: 0; width: 100%; height: 100%;"></div>
</div>
<script>
var myChart = echarts.init(document.getElementById('wordcloud'));
var option = {
series: [{
type: 'wordCloud',
shape: 'circle',
gridSize: 10,
sizeRange: [12, 50],
rotationRange: [-90, 90],
textStyle: {
normal: {
fontFamily: 'sans-serif',
color: function() {
return 'rgb(' + [
Math.round(Math.random() * 160),
Math.round(Math.random() * 160),
Math.round(Math.random() * 160)
].join(',') + ')';
}
}
},
data: [
{
name: '词语1',
value: 50,
textStyle: {
normal: {
fontSize: 30
}
},
// 设置背景图
itemStyle: {
normal: {
backgroundImage: 'url(word1.png)',
backgroundSize: '100% 100%'
}
}
},
{
name: '词语2',
value: 30,
textStyle: {
normal: {
fontSize: 20
}
},
// 设置背景图
itemStyle: {
normal: {
backgroundImage: 'url(word2.png)',
backgroundSize: '100% 100%'
}
}
},
// 其他词语...
]
}]
};
myChart.setOption(option);
</script>
</body>
</html>
```
需要注意的是,添加背景图会增加页面的加载时间和资源消耗,因此建议在使用时进行适当的优化。
阅读全文
相关推荐
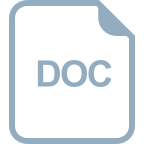
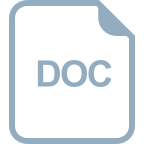
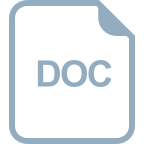
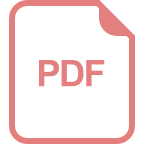


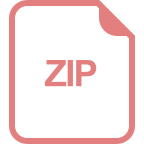
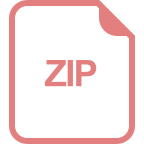
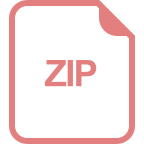
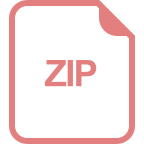
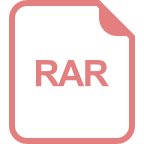
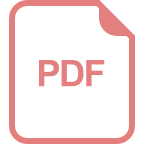
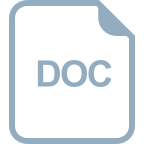
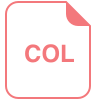
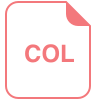
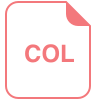

