将Student对象(属性:int id, String name(不多于10个字符),int age,double grade)写入文件、读出文件,分别尝试使用以下方式实现: (1)DataInputStream, DataOutputStream; (2)Scanner(或BufferedReader), PrintWriter; (3)ObjectInputStream, ObjectOutputStream。 问题:1、2、3方法生成的文件大小各是多少?尝试分析1和2输出的文件大小。 要求:以方法的形式进行封装(使用方法重载),例如: public static void writeStudent(Student s, DataOutputStream dos){}; 读取时类似: public static Student readStudent(DataInputStream dis){};
时间: 2023-12-03 21:43:06 浏览: 49
以下是三种方法的实现代码:
使用 DataInputStream 和 DataOutputStream:
```java
public static void writeStudent(Student s, DataOutputStream dos) throws IOException{
dos.writeInt(s.getId());
dos.writeUTF(s.getName());
dos.writeInt(s.getAge());
dos.writeDouble(s.getGrade());
}
public static Student readStudent(DataInputStream dis) throws IOException{
int id = dis.readInt();
String name = dis.readUTF();
int age = dis.readInt();
double grade = dis.readDouble();
return new Student(id, name, age, grade);
}
```
使用 Scanner(或 BufferedReader)和 PrintWriter:
```java
public static void writeStudent(Student s, PrintWriter pw){
pw.println(s.getId());
pw.println(s.getName());
pw.println(s.getAge());
pw.println(s.getGrade());
}
public static Student readStudent(Scanner scanner){
int id = scanner.nextInt();
String name = scanner.nextLine();
int age = scanner.nextInt();
double grade = scanner.nextDouble();
return new Student(id, name, age, grade);
}
```
使用 ObjectInputStream 和 ObjectOutputStream:
```java
public static void writeStudent(Student s, ObjectOutputStream oos) throws IOException{
oos.writeObject(s);
}
public static Student readStudent(ObjectInputStream ois) throws IOException, ClassNotFoundException{
return (Student) ois.readObject();
}
```
其中,Student 类的代码如下:
```java
public class Student implements Serializable{
private int id;
private String name;
private int age;
private double grade;
public Student(int id, String name, int age, double grade) {
this.id = id;
this.name = name;
this.age = age;
this.grade = grade;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public double getGrade() {
return grade;
}
}
```
使用方法重载,我们可以将这些方法写在一个类中,并提供以下方法:
```java
public static void writeStudent(Student s, String filePath, int methodType) throws IOException{
switch (methodType){
case 1:
DataOutputStream dos = new DataOutputStream(new FileOutputStream(filePath));
writeStudent(s, dos);
dos.close();
break;
case 2:
PrintWriter pw = new PrintWriter(new FileWriter(filePath));
writeStudent(s, pw);
pw.close();
break;
case 3:
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(filePath));
writeStudent(s, oos);
oos.close();
break;
default:
System.out.println("Invalid method type!");
break;
}
}
public static Student readStudent(String filePath, int methodType) throws IOException, ClassNotFoundException{
switch (methodType){
case 1:
DataInputStream dis = new DataInputStream(new FileInputStream(filePath));
Student s1 = readStudent(dis);
dis.close();
return s1;
case 2:
Scanner scanner = new Scanner(new File(filePath));
Student s2 = readStudent(scanner);
scanner.close();
return s2;
case 3:
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filePath));
Student s3 = readStudent(ois);
ois.close();
return s3;
default:
System.out.println("Invalid method type!");
return null;
}
}
```
我们可以通过调用 `writeStudent` 方法将 Student 对象写入文件,例如:
```java
Student s = new Student(123, "Alice", 18, 95.0);
writeStudent(s, "student.dat", 1);
```
这将使用 DataOutputStream 将 Student 对象写入名为 `student.dat` 的文件中。同样地,我们可以通过调用 `readStudent` 方法从文件中读取 Student 对象,例如:
```java
Student s = readStudent("student.dat", 1);
```
这将使用 DataInputStream 从名为 `student.dat` 的文件中读取 Student 对象。同样的方法也适用于使用其他两种方式写入和读取 Student 对象。
相关推荐
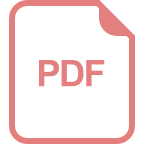
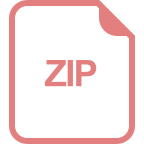
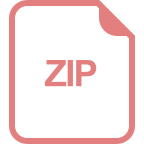














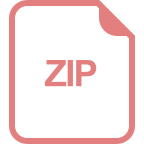