C++ 给定一个字符串 s 和一个字符串数组 words。 words 中所有字符串 长度相同。 s 中的 串联子串 是指一个包含 words 中所有字符串以任意顺序排列连接起来的子串。 例如,如果 words = ["ab","cd","ef"], 那么 "abcdef", "abefcd","cdabef", "cdefab","efabcd", 和 "efcdab" 都是串联子串。 "acdbef" 不是串联子串,因为他不是任何 words 排列的连接。 返回所有串联字串在 s 中的开始索引。你可以以 任意顺序 返回答案。
时间: 2023-07-08 08:52:46 浏览: 30
这是一个经典的字符串问题,可以使用滑动窗口和哈希表来解决。
具体实现如下:
```c++
vector<int> findSubstring(string s, vector<string>& words) {
vector<int> res;
if (s.empty() || words.empty()) {
return res;
}
int word_len = words[0].size();
int total_len = words.size() * word_len;
unordered_map<string, int> word_dict;
for (const auto& word : words) {
++word_dict[word];
}
for (int i = 0; i <= (int)s.size() - total_len; ++i) {
unordered_map<string, int> cur_dict;
int j = i;
while (j < i + total_len) {
string cur_word = s.substr(j, word_len);
if (word_dict.count(cur_word) == 0) {
break;
}
++cur_dict[cur_word];
if (cur_dict[cur_word] > word_dict[cur_word]) {
break;
}
j += word_len;
}
if (j == i + total_len) {
res.push_back(i);
}
}
return res;
}
```
其中,word_dict 是存储 words 数组中所有字符串的哈希表,cur_dict 是存储当前子串中所有字符串的哈希表。在每次移动滑动窗口时,需要将相应的单词从哈希表中删除或加入。由于需要求出所有符合条件的子串,因此需要遍历所有可能的起始位置,并将符合条件的起始位置存入结果数组中。
相关推荐
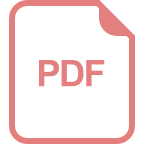
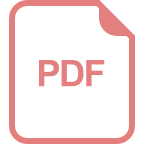















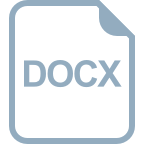