android不通过proc获取应用的so模块的基地址
时间: 2023-12-06 21:39:32 浏览: 35
在 Android 中,可以通过链接器的动态链接库(Dynamic Linker)获取应用的 SO 模块的基地址。下面是一些示例代码:
```java
// 获取当前应用的进程 ID
int pid = android.os.Process.myPid();
// 获取链接器的动态链接库
String linkerPath = "/system/bin/linker";
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
linkerPath = "/system/bin/linker64";
}
RandomAccessFile linkerFile = new RandomAccessFile(linkerPath, "r");
FileChannel channel = linkerFile.getChannel();
MappedByteBuffer buffer = channel.map(FileChannel.MapMode.READ_ONLY, 0, channel.size());
linkerFile.close();
// 在链接器中搜索符号,根据符号的位置计算出模块的基地址
String symbol = "libm.so";
long base = 0;
int index = 0;
while (base == 0) {
index = buffer.indexOf(symbol.getBytes(), index + 1);
if (index < 0) {
break;
}
base = getModuleBaseAddress(pid, index);
}
```
其中,`getModuleBaseAddress` 函数用于计算模块的基地址,示例代码如下:
```java
private static long getModuleBaseAddress(int pid, int index) {
try {
// 读取进程的 /proc/pid/maps 文件,获取模块的内存映射信息
String mapsPath = String.format("/proc/%d/maps", pid);
FileReader fr = new FileReader(mapsPath);
BufferedReader reader = new BufferedReader(fr);
String line;
while ((line = reader.readLine()) != null) {
String[] fields = line.split(" ");
if (fields.length < 6) {
continue;
}
String name = fields[5];
if (!name.endsWith(".so")) {
continue;
}
if (name.contains("linker") || name.contains("libc")) {
continue;
}
// 获取模块的地址范围
String[] range = fields[0].split("-");
long start = Long.parseLong(range[0], 16);
long end = Long.parseLong(range[1], 16);
// 判断符号是否在模块内部
if (start <= index && index < end) {
return start;
}
}
reader.close();
fr.close();
} catch (Exception e) {
e.printStackTrace();
}
return 0;
}
```
需要注意的是,这种方法需要读取进程的 `/proc/pid/maps` 文件,因此需要具有 root 权限,而且不保证在所有 Android 版本和设备上都可行。建议在实际使用时进行充分测试。
相关推荐
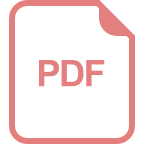
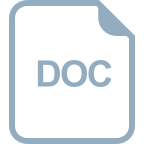














