python 解决websocket服务端
时间: 2023-09-28 17:04:16 浏览: 167
在 Python 中,可以使用 `websocket` 模块来实现 WebSocket 服务端。下面是一个简单的例子:
```python
import asyncio
import websockets
async def echo(websocket, path):
async for message in websocket:
await websocket.send(message)
async def main():
async with websockets.serve(echo, "localhost", 8765):
await asyncio.Future() # run forever
asyncio.run(main())
```
这个例子实现了一个简单的 Echo 服务器,它会将客户端发来的消息原封不动地返回给客户端。你只需要将 `echo` 函数替换成你自己的处理逻辑即可。
要运行这个服务器,只需要在命令行中执行以下命令:
```
$ python3 server.py
```
其中 `server.py` 是你编写的 Python 文件名。服务器将会在本地的 `8765` 端口上监听 WebSocket 连接。你可以使用任何支持 WebSocket 的客户端连接到这个服务器。
相关问题
python实现websocket服务端
以下是使用Pywss模块实现WebSocket服务端的Python代码示例:
```python
from pywss import PywsServer
class MyServer(PywsServer):
def example_1(self, request, data):
# 处理客户端发送的数据
# ...
# 向客户端发送数据
self.send(request, 'Hello, client!')
server = MyServer('127.0.0.1', 8001)
server.start()
```
上述代码中,我们首先导入了Pywss模块,然后定义了一个名为MyServer的WebSocket服务端类,该类继承自PywsServer类。在MyServer类中,我们定义了一个名为example_1的方法,该方法用于处理客户端发送的数据,并向客户端发送数据。最后,我们创建了一个MyServer对象,并启动了WebSocket服务端。
用python实现websocket服务端
要使用Python实现WebSocket服务器,可以使用Tornado库。下面是一个简单的示例:
```python
import tornado.websocket
import tornado.web
import tornado.ioloop
class WebSocketHandler(tornado.websocket.WebSocketHandler):
def open(self):
print("WebSocket opened")
def on_message(self, message):
print("Received message: {0}".format(message))
self.write_message("You said: " + message)
def on_close(self):
print("WebSocket closed")
app = tornado.web.Application([
(r"/websocket", WebSocketHandler),
])
if __name__ == "__main__":
app.listen(8888)
tornado.ioloop.IOLoop.instance().start()
```
在上面的代码中,我们创建了一个名为WebSocketHandler的类,它继承自Tornado的WebSocketHandler类。我们覆盖了open()、on_message()和on_close()方法,以处理WebSocket的连接、消息和关闭事件。
然后,我们创建了一个名为app的Tornado应用程序,并将WebSocketHandler绑定到路径“/websocket”。最后,我们启动了Tornado的I/O循环,开始监听传入的连接。
要测试WebSocket服务器,请使用浏览器或WebSocket客户端连接到“ws://localhost:8888/websocket”。您应该能够发送和接收消息。
阅读全文
相关推荐
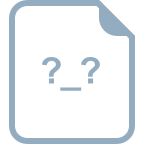
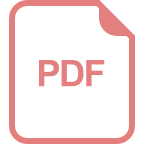




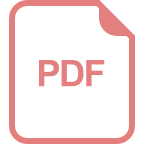






