Python写一个爬虫代码,爬取网易音乐
时间: 2023-11-21 15:52:12 浏览: 71
好的,以下是一个简单的爬虫代码,可以爬取网易云音乐的歌曲信息(包括歌曲名、歌手、专辑名、歌曲链接等):
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头信息
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 构造URL
url = 'https://music.163.com/discover/toplist?id=3778678'
# 发送请求,获取响应
response = requests.get(url, headers=headers)
# 解析响应内容
soup = BeautifulSoup(response.text, 'html.parser')
song_list = soup.find('ul', {'class': 'f-hide'}).find_all('a')
# 循环遍历歌曲列表,输出歌曲信息
for song in song_list:
song_name = song.text
song_url = 'https://music.163.com' + song['href']
song_id = song['href'].split('=')[-1]
singer_url = 'https://music.163.com/artist?id=' + song_id
album_url = 'https://music.163.com/album?id=' + song_id
# 发送歌手页面请求,获取歌手姓名
singer_response = requests.get(singer_url, headers=headers)
singer_soup = BeautifulSoup(singer_response.text, 'html.parser')
singer_name = singer_soup.find('h2', {'id': 'artist-name'}).text.strip()
# 发送专辑页面请求,获取专辑名称
album_response = requests.get(album_url, headers=headers)
album_soup = BeautifulSoup(album_response.text, 'html.parser')
album_name = album_soup.find('h2', {'class': 'f-ff2'}).text.strip()
print('歌曲名:', song_name)
print('歌手:', singer_name)
print('专辑名:', album_name)
print('歌曲链接:', song_url)
print('\n')
```
这段代码使用了 requests 库和 BeautifulSoup 库来发送请求和解析响应内容。具体实现过程如下:
1. 设置请求头信息,模拟浏览器发送请求,避免被网站识别为爬虫。
2. 构造 URL,这里以网易云音乐“飙升榜”的页面为例。
3. 发送请求,获取响应。
4. 解析响应内容,通过 BeautifulSoup 库找到歌曲列表的 ul 标签,再遍历其中的每一个 li 标签,获取歌曲名、歌曲链接等信息。
5. 对于每一个歌曲,需要再发送两个请求,分别获取该歌曲的歌手姓名和专辑名称。这里直接构造歌手和专辑的 URL,发送请求并解析响应内容即可。
6. 最后输出歌曲的相关信息。
需要注意的是,网站会对频繁的爬取行为进行限制,因此在实际应用中需要加上适当的延时和异常处理,避免被封禁。同时,也需要遵循网站的 robots.txt 文件,避免爬取不允许的内容。
相关推荐
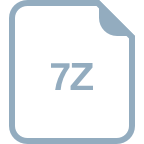
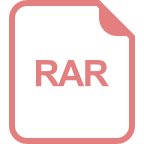













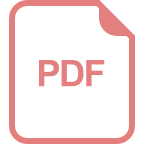
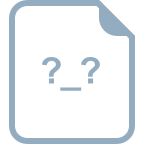