IntentService 如何使用
时间: 2023-07-20 22:33:50 浏览: 114
IntentService是用来执行一些后台任务的服务,它可以在后台执行耗时操作,并且在操作完成后自动停止。以下是使用IntentService的基本步骤:
1.创建一个类继承IntentService,并重写它的onHandleIntent()方法,该方法将在IntentService接收到请求时被调用。
```java
public class MyIntentService extends IntentService {
public MyIntentService() {
super("MyIntentService");
}
@Override
protected void onHandleIntent(Intent intent) {
// 处理请求
}
}
```
2.在需要执行后台任务的地方,创建一个Intent对象,并将它发送给IntentService。
```java
Intent intent = new Intent(this, MyIntentService.class);
startService(intent);
```
需要注意的是,IntentService会自动创建一个工作线程来执行onHandleIntent()方法中的操作,并在操作完成后自动停止服务。因此,我们不需要手动管理线程和服务的生命周期,可以方便地执行后台任务。同时,如果需要在IntentService中执行多个任务,可以在onHandleIntent()方法中处理多个请求。
相关问题
android 在intentService使用mqtt
您好,如果您想在 IntentService 中使用 MQTT 协议进行消息传输,可以使用 Eclipse Paho Android 客户端库。以下是在 IntentService 中使用 Paho Android 客户端库实现 MQTT 的基本步骤:
1. 添加 Paho Android 客户端库到您的项目中。
2. 在 IntentService 的 `onCreate()` 方法中创建 MQTT 客户端并连接到 MQTT 代理服务器。您需要指定代理服务器的地址和端口号。可以使用 `MqttConnectOptions` 类设置连接选项,例如设置连接的用户名和密码、清除会话标志等。
3. 在 `onHandleIntent()` 方法中订阅主题、发布消息和处理接收到的消息。您需要实现 `MqttCallback` 接口,并在回调方法中处理接收到的消息。
以下是在 IntentService 中连接到 MQTT 代理服务器的示例代码:
```java
public class MyIntentService extends IntentService {
private static final String TAG = MyIntentService.class.getSimpleName();
private MqttAndroidClient client;
public MyIntentService() {
super(TAG);
}
@Override
public void onCreate() {
super.onCreate();
String brokerUrl = "tcp://mqtt.eclipse.org:1883";
String clientId = MqttClient.generateClientId();
client = new MqttAndroidClient(this, brokerUrl, clientId);
MqttConnectOptions options = new MqttConnectOptions();
options.setUserName("username");
options.setPassword("password".toCharArray());
options.setCleanSession(true);
try {
IMqttToken token = client.connect(options);
token.waitForCompletion();
} catch (MqttException e) {
Log.e(TAG, "Failed to connect to MQTT broker", e);
}
}
@Override
protected void onHandleIntent(Intent intent) {
// 在这里订阅主题、发布消息和处理接收到的消息
// ...
// 订阅主题
String topic = "my/topic";
int qos = 1;
try {
IMqttToken token = client.subscribe(topic, qos);
token.waitForCompletion();
} catch (MqttException e) {
Log.e(TAG, "Failed to subscribe to topic: " + topic, e);
}
// 发布消息
String message = "Hello, MQTT!";
try {
client.publish(topic, message.getBytes(), qos, false);
} catch (MqttException e) {
Log.e(TAG, "Failed to publish message: " + message, e);
}
}
@Override
public void onDestroy() {
super.onDestroy();
try {
IMqttToken token = client.disconnect();
token.waitForCompletion();
} catch (MqttException e) {
Log.e(TAG, "Failed to disconnect from MQTT broker", e);
}
}
}
```
您还需要实现 `MqttCallback` 接口并在回调方法中处理接收到的消息。例如:
```java
client.setCallback(new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
// 处理连接丢失事件
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
// 处理接收到的消息
String payload = new String(message.getPayload());
Log.d(TAG, "Received message: " + payload);
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
// 处理消息发送完成事件
}
});
```
在 `onDestroy()` 方法中断开 MQTT 客户端与代理服务器的连接。
希望这些信息能对您有所帮助。
android 在intentService使用mqtt 代码
以下是在 IntentService 中使用 MQTT 的示例代码:
```java
public class MqttService extends IntentService {
private MqttAndroidClient mqttClient;
public MqttService() {
super("MqttService");
}
@Override
protected void onHandleIntent(Intent intent) {
String clientId = MqttClient.generateClientId();
mqttClient = new MqttAndroidClient(this, "tcp://mqtt.eclipse.org:1883", clientId);
try {
mqttClient.connect().waitForCompletion();
mqttClient.subscribe("topic/test", 0);
mqttClient.setCallback(new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
// 连接丢失
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
// 收到消息
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
// 传输完成
}
});
} catch (MqttException e) {
e.printStackTrace();
}
}
@Override
public void onDestroy() {
super.onDestroy();
try {
mqttClient.disconnect().waitForCompletion();
} catch (MqttException e) {
e.printStackTrace();
}
}
}
```
在 `onHandleIntent` 方法中,我们创建了一个 MQTT 客户端,并连接到 MQTT 服务器。然后订阅了一个主题,并设置了回调函数来处理收到的消息。在 `onDestroy` 方法中,我们断开了 MQTT 客户端的连接。
需要注意的是,由于 IntentService 是单线程的,因此在 `onHandleIntent` 方法中不能进行耗时操作,否则可能会导致 IntentService 超时而被系统杀死。如果需要进行耗时操作,可以考虑使用 AsyncTask 或 HandlerThread 等方式。
阅读全文
相关推荐
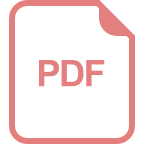
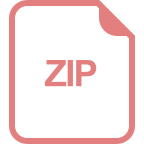
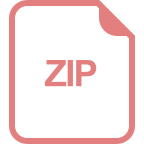
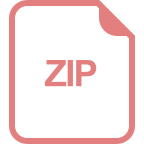
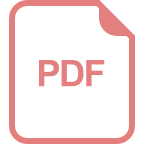


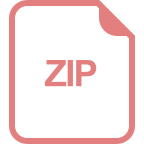
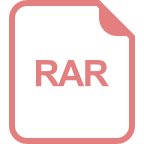
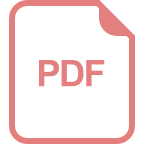
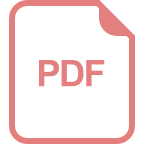
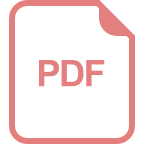

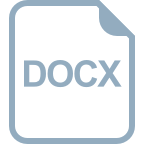
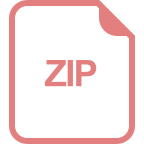