如何使用c++新开线程做某事,并等待全部线程结束
时间: 2023-08-05 21:33:42 浏览: 174
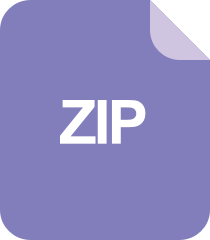
C++ 等待线程结束

在 C++ 中,可以使用 `<thread>` 头文件中的 `std::thread` 类来创建新线程。
为了等待所有线程结束,可以使用 `std::thread` 的 `join()` 方法,它会阻塞当前线程,直到对应的线程执行完毕。
下面是一个示例代码,创建了多个线程,每个线程都会打印出自己的线程 ID,然后等待所有线程执行完毕:
```cpp
#include <iostream>
#include <thread>
#include <vector>
void worker(int id) {
std::cout << "Thread " << id << " started" << std::endl;
// do some work
std::cout << "Thread " << id << " finished" << std::endl;
}
int main() {
std::vector<std::thread> threads;
const int num_threads = 5;
for (int i = 0; i < num_threads; ++i) {
threads.emplace_back(worker, i);
}
// Wait for all threads to finish
for (auto& t : threads) {
t.join();
}
std::cout << "All threads finished" << std::endl;
return 0;
}
```
在上面的示例代码中,`std::vector<std::thread> threads` 是一个线程数组,我们创建了 `num_threads` 个线程,并将它们放入数组中。每个线程调用 `worker()` 函数,并传入自己的线程 ID。
在主线程中,我们使用 `join()` 方法等待所有线程执行完毕,然后打印出提示信息。
阅读全文
相关推荐
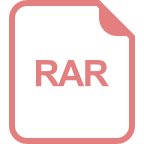
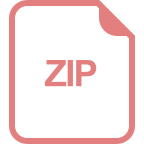
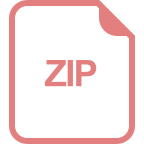
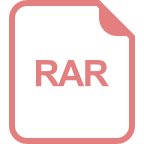
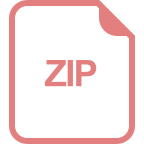
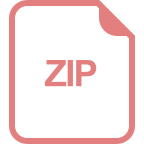
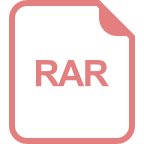
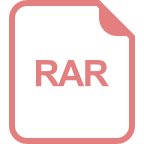
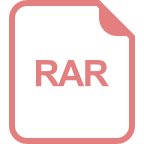
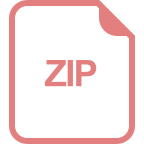
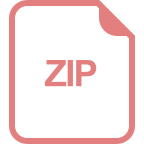
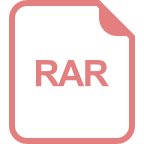
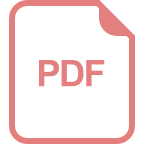
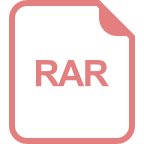
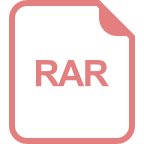