请编写程序,读入CSV文件中(const.csv)数据。根据用户输入的出生日期(格式为YYYYMMDD),输出对应星座名称。如果输入的格式有误,请输出“输入日期错误!”
时间: 2024-06-10 12:05:20 浏览: 107
好的,我可以帮您编写这个程序。以下是Python代码实现:
```python
import csv
const_data = [] # 保存星座信息的列表
# 读取CSV文件
with open('const.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
const_data.append(row)
# 输入出生日期
birthdate = input('请输入出生日期(格式为YYYYMMDD): ')
# 判断日期格式是否正确
if not birthdate.isdigit() or len(birthdate) != 8:
print('输入日期错误!')
else:
# 获取月份和日期
month = int(birthdate[4:6])
day = int(birthdate[6:])
# 根据月份和日期确定星座
const_name = ''
if (month == 1 and day >= 20) or (month == 2 and day <= 18):
const_name = const_data[0][1]
elif (month == 2 and day >= 19) or (month == 3 and day <= 20):
const_name = const_data[1][1]
elif (month == 3 and day >= 21) or (month == 4 and day <= 19):
const_name = const_data[2][1]
elif (month == 4 and day >= 20) or (month == 5 and day <= 20):
const_name = const_data[3][1]
elif (month == 5 and day >= 21) or (month == 6 and day <= 21):
const_name = const_data[4][1]
elif (month == 6 and day >= 22) or (month == 7 and day <= 22):
const_name = const_data[5][1]
elif (month == 7 and day >= 23) or (month == 8 and day <= 22):
const_name = const_data[6][1]
elif (month == 8 and day >= 23) or (month == 9 and day <= 22):
const_name = const_data[7][1]
elif (month == 9 and day >= 23) or (month == 10 and day <= 23):
const_name = const_data[8][1]
elif (month == 10 and day >= 24) or (month == 11 and day <= 22):
const_name = const_data[9][1]
elif (month == 11 and day >= 23) or (month == 12 and day <= 21):
const_name = const_data[10][1]
elif (month == 12 and day >= 22) or (month == 1 and day <= 19):
const_name = const_data[11][1]
# 输出结果
if const_name:
print('您的星座是:', const_name)
else:
print('输入日期错误!')
```
注意,此程序需要在同一目录下保存一个名为`const.csv`的CSV文件,其中包含了每个星座的信息,格式如下:
```
1,Aquarius,1月20日-2月18日
2,Pisces,2月19日-3月20日
3,Aries,3月21日-4月19日
4,Taurus,4月20日-5月20日
5,Gemini,5月21日-6月21日
6,Cancer,6月22日-7月22日
7,Leo,7月23日-8月22日
8,Virgo,8月23日-9月22日
9,Libra,9月23日-10月23日
10,Scorpio,10月24日-11月22日
11,Sagittarius,11月23日-12月21日
12,Capricorn,12月22日-1月19日
```
您可以将此内容复制到一个`const.csv`文件中保存。
阅读全文
相关推荐














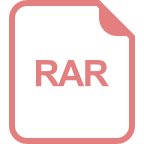

