如何快速的实时插入neo4j数据
时间: 2023-11-16 13:38:53 浏览: 34
要实现快速的实时插入neo4j数据,可以考虑以下几个方面:
1. 使用Cypher语句:Cypher是Neo4j的查询和操作语言,使用Cypher语句可以在Neo4j中快速地插入数据。
2. 批量导入:可以使用Neo4j提供的批量导入工具,将数据以CSV格式存储,再通过Cypher语句进行导入。
3. 使用索引:在插入数据之前,可以为数据模型创建索引,这样在插入数据时可以更快地找到对应节点和关系。
4. 使用Neo4j的事务:事务可以保证数据插入的完整性和一致性,同时也可以提高插入数据的效率。
综上所述,以上几种方法可以帮助快速实现实时插入Neo4j数据。
相关问题
如何使用sping data neo4j 快速实时插入大量节点数据?
使用Spring Data Neo4j可以通过以下步骤快速实时插入大量节点数据:
1. 配置Neo4j数据库连接:在application.properties文件中添加Neo4j数据库连接信息。
2. 创建节点实体类:使用@NodeEntity注解创建节点实体类,并在类中定义节点属性。
3. 创建节点仓库:使用@NodeRepository注解创建节点仓库,并继承Neo4jRepository接口。
4. 使用Neo4jTemplate插入数据:使用Neo4jTemplate的save方法插入节点数据。可以使用batch方法批量插入数据。
示例代码如下:
```java
// 配置Neo4j数据库连接
@Configuration
@EnableNeo4jRepositories(basePackages = "com.example.repository")
public class Neo4jConfig extends Neo4jConfiguration {
@Bean
public SessionFactory getSessionFactory() {
return new SessionFactory(configuration(), "com.example.entity");
}
@Bean
public org.neo4j.ogm.config.Configuration configuration() {
org.neo4j.ogm.config.Configuration configuration = new org.neo4j.ogm.config.Configuration.Builder()
.uri("bolt://localhost")
.credentials("neo4j", "password")
.build();
return configuration;
}
}
// 创建节点实体类
@NodeEntity
public class Person {
@Id
@GeneratedValue
private Long id;
private String name;
// getters and setters
}
// 创建节点仓库
@Repository
public interface PersonRepository extends Neo4jRepository<Person, Long> {
}
// 插入数据
@Service
public class PersonService {
@Autowired
private Neo4jTemplate neo4jTemplate;
@Autowired
private PersonRepository personRepository;
public void savePerson(Person person) {
// 使用Neo4jTemplate插入数据
neo4jTemplate.save(person);
// 批量插入数据
List<Person> persons = new ArrayList<>();
for (int i = 0; i < 10000; i++) {
Person p = new Person();
p.setName("person" + i);
persons.add(p);
}
neo4jTemplate.batchSave(persons);
}
}
```
以上是一个简单的示例,你可以按照自己的需求进行适当的修改和扩展。
如何使用spring-data-neo4j快速实时插入大量节点数据?
可以使用Spring Data Neo4j的Batch API来实现快速实时插入大量节点数据。具体步骤如下:
1. 创建一个节点实体类,使用`@NodeEntity`注解标注。
2. 在该实体类中定义节点属性。
3. 使用`@Repository`注解标注一个数据访问对象(DAO)接口。
4. 在DAO接口中定义一个继承自`Neo4jRepository`的子接口,该接口提供了一些基本的CRUD操作。
5. 在DAO接口中定义一个自定义方法,使用`@Query`注解指定Cypher语句,用于批量插入节点数据。
6. 在应用程序中,使用`@Autowired`注解自动注入DAO接口,调用自定义方法实现批量插入节点数据。
以下是一个示例代码:
```
@NodeEntity
public class MyNode {
@Id
@GeneratedValue
private Long id;
private String name;
// getters and setters
}
@Repository
public interface MyNodeRepository extends Neo4jRepository<MyNode, Long> {
@Query("UNWIND {nodes} AS node CREATE (n:MyNode) SET n=node")
void batchInsert(@Param("nodes") List<MyNode> nodes);
}
@Service
public class MyNodeService {
@Autowired
private MyNodeRepository repository;
public void batchInsertNodes(List<MyNode> nodes) {
repository.batchInsert(nodes);
}
}
```
在上面的示例代码中,`MyNode`类是一个节点实体类,`MyNodeRepository`是一个DAO接口,提供了批量插入节点数据的自定义方法`batchInsert`。`MyNodeService`是一个服务类,用于调用DAO接口中的方法实现批量插入节点数据。
调用`MyNodeService`的`batchInsertNodes`方法即可实现快速实时插入大量节点数据。
相关推荐
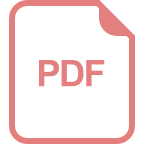
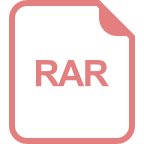
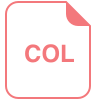











