用js设置两个按钮切换文本的两种颜色
时间: 2024-02-17 12:05:10 浏览: 23
可以通过以下代码实现:
HTML代码:
```html
<button id="btn1">按钮1</button>
<button id="btn2">按钮2</button>
<p id="text">这是一段文本</p>
```
JS代码:
```javascript
const btn1 = document.getElementById('btn1');
const btn2 = document.getElementById('btn2');
const text = document.getElementById('text');
btn1.addEventListener('click', function() {
text.style.color = 'red';
});
btn2.addEventListener('click', function() {
text.style.color = 'blue';
});
```
以上代码中,我们通过获取按钮元素和文本元素的引用,然后给按钮元素添加点击事件监听器,在点击事件中改变文本元素的颜色。当点击按钮1时,文本颜色变为红色;当点击按钮2时,文本颜色变为蓝色。
相关问题
用js设置两个按钮点击不同按钮切换文本的颜色
可以使用以下代码实现:
HTML代码:
```
<button id="btn1" onclick="changeText('btn1')">按钮1</button>
<button id="btn2" onclick="changeText('btn2')">按钮2</button>
<p id="text">这是一段文本</p>
```
JS代码:
```
function changeText(btnId) {
var clickedBtn = document.getElementById(btnId);
var otherBtn;
if (btnId === 'btn1') {
otherBtn = document.getElementById('btn2');
} else {
otherBtn = document.getElementById('btn1');
}
var text = document.getElementById('text');
if (clickedBtn.style.color !== 'red') {
clickedBtn.style.color = 'red';
otherBtn.style.color = '';
text.style.color = 'red';
} else {
clickedBtn.style.color = '';
text.style.color = '';
}
}
```
当点击按钮1时,按钮1和文本的颜色会变成红色,按钮2的颜色变成默认颜色;当点击按钮2时,按钮2和文本的颜色会变成红色,按钮1的颜色变成默认颜色。如果再次点击已经变成红色的按钮,则按钮和文本的颜色会变回默认颜色。
用js设置两个按钮点击哪个按钮切换哪个按钮的颜色
可以使用以下代码实现:
HTML代码:
```
<button id="btn1" onclick="changeColor('btn1')">按钮1</button>
<button id="btn2" onclick="changeColor('btn2')">按钮2</button>
```
JS代码:
```
function changeColor(btnId) {
var clickedBtn = document.getElementById(btnId);
var otherBtn;
if (btnId === 'btn1') {
otherBtn = document.getElementById('btn2');
} else {
otherBtn = document.getElementById('btn1');
}
clickedBtn.style.backgroundColor = 'red';
otherBtn.style.backgroundColor = '';
}
```
当点击按钮1时,按钮1的背景色会变成红色,按钮2的背景色变成默认颜色;当点击按钮2时,按钮2的背景色会变成红色,按钮1的背景色变成默认颜色。
相关推荐
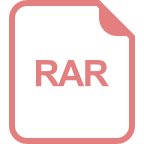
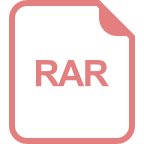
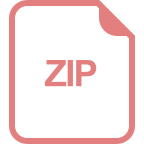












