public static HttpResult AutoSend(this HttpRequest http) { HttpResult _result = http.Send(); StringBuilder _errMsg = new StringBuilder(); if (!_result.State) { _errMsg.AppendLine("Http-" + _result.Method + "错误:"); _errMsg.AppendLine("请求地址:" + _result.Url); _errMsg.Append("错误信息:" + _result.ErrMsg); Fn.Log.WriteInfo(_errMsg.ToStr()); throw Oops.Bah(_errMsg.ToString()); } else { if (_result.ContentType == HttpResponseContentType.Stream) { return _result; } else { if (_result.ResultJson == null) { _errMsg.AppendLine("Http-" + _result.Method + "错误:"); _errMsg.AppendLine("请求地址:" + _result.Url); _errMsg.AppendLine("错误信息:目标接口无返回数据!"); _errMsg.Append(_result.Result); throw Oops.Bah(_errMsg.ToString()); } if (_result.ResultJson["code"].ToInt() != 200) { if (_result.ResultJson["msg"].GetType() == typeof(JObject)) { JToken _msg = _result.ResultJson["msg"]; foreach (JProperty _jp in _msg.Cast<JProperty>()) { if (_jp.Value.GetType() == typeof(JArray)) { throw Oops.Oh(_jp.Value[0].ToStr()); } } } throw new Exception(_result.ResultJson["msg"].ToStr()); //_errMsg.AppendLine("Http-" + _result.Method + "错误:"); //_errMsg.AppendLine("请求地址:" + _result.Url); //_errMsg.AppendLine("错误信息:"+ _result.Result); //throw new Exception(_errMsg.ToStr()); } else { if (_result.ContentType == HttpResponseContentType.JSON) _result.ResultJson = _result.ResultJson["data"]; } return _result; } } }
时间: 2024-02-10 12:20:27 浏览: 29
这段代码看起来是一个扩展方法,将HttpRequest类型的实例对象扩展了一个名为AutoSend的方法。AutoSend方法似乎是用于自动发送HTTP请求并处理响应结果的。
具体来说,这个方法会先调用http对象的Send方法发送HTTP请求,然后根据响应结果的状态码、内容类型等信息进行处理。如果响应结果状态码不为200,就抛出异常;如果状态码为200,就在返回结果中提取"data"字段的值并返回。
需要注意的是,这段代码中有一些自定义的类型和方法,比如HttpResult、HttpResponseContentType、Oops.Bah等,这些类型和方法的具体实现需要结合上下文来理解。
相关问题
优化这个方法:private void saveFileBrowseRecord(LayoutElementParcelable file) { // 当前的目录 String currentPath = file.desc.substring(0, file.desc.lastIndexOf(File.separator)); String basePath = mSourceRootPath; if (mTransferType == U_FTP_TO_FAB_FTP) { basePath = mSourcePath; } XLog.tag(TAG).i("mCurrentPath:" + currentPath + " basePath:" + basePath); HybridFileParcelable hybridFileParcelable = new HybridFileParcelable(currentPath, basePath, file.desc, file.permissions, file.date, file.longSize, file.isDirectory, file.getMode(), ""); if (!TextUtils.isEmpty(file.title)) { hybridFileParcelable.setName(file.title); } Map<String, Object> map = new HashMap<>(); // 文件路径全名称 map.put("dirFullName", hybridFileParcelable.getRelativePath()); // 文件服务器id map.put("fileServerId", mStoreServerId); RequestBody body = HttpClient.getRequestBody(map); TransferApi api = HttpClient.api(TransferApi.class, false); if (api == null) { XLog.tag(TAG).i("save file browse record api is null"); return; } // recordType 记录类型:默认0-最近浏览,1-收藏 api.saveFileBrowseRecord(body, 1) .compose(HttpClient.observableIoToMain()) .as(HttpClient.bindLifecycle(this)) .subscribe(new HttpDefaultObserver<HttpResult<String>>() { @Override public void start() { } @Override public void success(HttpResult<String> result) { mCollects.put(file.desc, file.desc); } @Override public void fail(HttpError e) { showWhiteFailToast(e.getCode(), e.getMessage(), null); } @Override public void finish() { } }); }
首先,可以将以下代码段:
```
HybridFileParcelable hybridFileParcelable = new HybridFileParcelable(currentPath,
basePath, file.desc, file.permissions, file.date,
file.longSize, file.isDirectory, file.getMode(), "");
if (!TextUtils.isEmpty(file.title)) {
hybridFileParcelable.setName(file.title);
}
```
替换为以下代码:
```
HybridFileParcelable hybridFileParcelable = new HybridFileParcelable(currentPath,
basePath, TextUtils.isEmpty(file.title) ? file.desc : file.title, file.permissions, file.date,
file.longSize, file.isDirectory, file.getMode(), "");
```
这样可以避免在判断 file.title 是否为空时多次调用 TextUtils.isEmpty() 方法。
其次,可以将 Map 的初始化改为以下方式:
```
Map<String, Object> map = new HashMap<>(2);
```
这样可以避免后续 put 操作导致 Map 扩容。
最后,建议对网络请求进行异常捕获处理,避免出现异常时程序崩溃。可以将以下代码:
```
api.saveFileBrowseRecord(body, 1)
.compose(HttpClient.observableIoToMain())
.as(HttpClient.bindLifecycle(this))
.subscribe(new HttpDefaultObserver<HttpResult<String>>() {
@Override
public void start() {
}
@Override
public void success(HttpResult<String> result) {
mCollects.put(file.desc, file.desc);
}
@Override
public void fail(HttpError e) {
showWhiteFailToast(e.getCode(), e.getMessage(), null);
}
@Override
public void finish() {
}
});
```
替换为以下代码:
```
try {
api.saveFileBrowseRecord(body, 1)
.compose(HttpClient.observableIoToMain())
.as(HttpClient.bindLifecycle(this))
.subscribe(new HttpDefaultObserver<HttpResult<String>>() {
@Override
public void start() {
}
@Override
public void success(HttpResult<String> result) {
mCollects.put(file.desc, file.desc);
}
@Override
public void fail(HttpError e) {
showWhiteFailToast(e.getCode(), e.getMessage(), null);
}
@Override
public void finish() {
}
});
} catch (Exception e) {
e.printStackTrace();
}
```
这样可以捕获网络请求中可能出现的异常,并在程序中进行处理。
@GetMapping(value = "/citys") public HttpResult findCitys(@RequestParam String provinceCode) { return HttpResult.ok(sysRegionService.findCitys(provinceCode)); }这段代码是什么意思
这段代码是在 Java 中的一个方法。这个方法的名称是 `findCitys`,它使用了 `@GetMapping` 注解,表示这个方法对应的是 HTTP 的 GET 请求,URL 为 `/citys`。方法中的参数 `provinceCode` 使用了 `@RequestParam` 注解,表示这个参数会从 HTTP 请求中的查询参数中获取。方法体内调用了 `sysRegionService.findCitys(provinceCode)` 方法,并将返回结果作为参数返回一个 `HttpResult` 对象。最后,方法使用 `HttpResult.ok()` 静态方法将这个 `HttpResult` 对象包装为一个表示成功的 HTTP 响应。
简而言之,这个方法处理 HTTP GET 请求 `/citys`,并根据传入的参数 `provinceCode` 获取一些信息,然后将这些信息作为 HTTP 响应的内容返回给客户端。
相关推荐
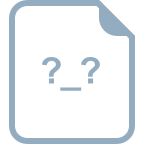








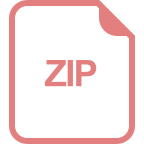
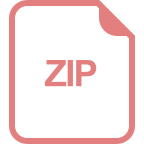