通过RTSP连接海康网络摄像头,如何通过C语言实现拍照功能并把照片存为jpg格式,请详细回答并注释
时间: 2024-06-09 13:08:03 浏览: 139
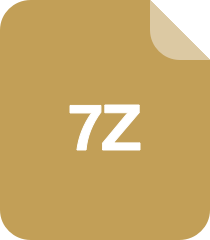
NetVideoActiveX23开发包
要通过RTSP连接海康网络摄像头并实现拍照功能,需要进行以下步骤:
1. 使用C语言中支持RTSP协议的库,如libcurl进行连接。
2. 通过RTSP连接获取视频流数据。
3. 使用视频编解码库,如FFmpeg,将视频流数据解码为图像数据。
4. 将图像数据保存为JPG格式的图片。
下面是具体实现步骤:
1. 引入头文件和库文件
```c
#include <stdio.h>
#include <stdlib.h>
#include <curl/curl.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <jpeglib.h>
```
2. 定义RTSP连接参数和回调函数
```c
#define RTSP_URL "rtsp://192.168.1.100:554/h264/ch1/main/av_stream"
#define IMAGE_WIDTH 640
#define IMAGE_HEIGHT 480
static size_t write_data(void *ptr, size_t size, size_t nmemb, void *stream) {
return fwrite(ptr, size, nmemb, (FILE *)stream);
}
static int interrupt_cb(void *ctx) {
return 0;
}
```
3. 实现连接和获取视频流数据
```c
int main(int argc, char **argv) {
CURL *curl;
CURLcode res;
FILE *fp;
AVFormatContext *pFormatCtx = NULL;
AVCodecContext *pCodecCtx = NULL;
AVCodec *pCodec = NULL;
AVPacket packet;
AVFrame *pFrame = NULL;
AVFrame *pFrameRGB = NULL;
struct SwsContext *img_convert_ctx = NULL;
uint8_t *buffer = NULL;
int videoStreamIndex = -1;
int numBytes;
int frameFinished;
char jpgFilename[] = "image.jpg";
// 初始化libcurl
curl = curl_easy_init();
if (curl) {
// 设置RTSP连接参数
curl_easy_setopt(curl, CURLOPT_URL, RTSP_URL);
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_data); // 设置回调函数
curl_easy_setopt(curl, CURLOPT_WRITEDATA, fp);
curl_easy_setopt(curl, CURLOPT_NOSIGNAL, 1L);
curl_easy_setopt(curl, CURLOPT_CONNECTTIMEOUT, 10L);
curl_easy_setopt(curl, CURLOPT_TIMEOUT, 10L);
curl_easy_setopt(curl, CURLOPT_NOPROGRESS, 1L);
curl_easy_setopt(curl, CURLOPT_USERAGENT, "curl/7.47.0");
curl_easy_setopt(curl, CURLOPT_RTSP_STREAM_URI, RTSP_URL);
curl_easy_setopt(curl, CURLOPT_RTSP_SESSION_ID, "");
curl_easy_setopt(curl, CURLOPT_RTSP_TRANSPORT, "udp");
curl_easy_setopt(curl, CURLOPT_RTSP_HEADER, NULL);
curl_easy_setopt(curl, CURLOPT_RANGE, NULL);
curl_easy_setopt(curl, CURLOPT_RTSP_REQUEST, CURL_RTSPREQ_OPTIONS);
// 打开文件用于保存图片
fp = fopen(jpgFilename, "wb");
if (fp == NULL) {
printf("Error: failed to open file %s\n", jpgFilename);
return -1;
}
// 开始连接
res = curl_easy_perform(curl);
if (res != CURLE_OK) {
printf("Error: curl_easy_perform failed: %s\n", curl_easy_strerror(res));
return -1;
}
// 关闭文件
fclose(fp);
}
// 初始化libavformat
av_register_all();
avformat_network_init();
// 打开RTSP流并获取流信息
if (avformat_open_input(&pFormatCtx, RTSP_URL, NULL, NULL) != 0) {
printf("Error: failed to open RTSP stream\n");
return -1;
}
if (avformat_find_stream_info(pFormatCtx, NULL) < 0) {
printf("Error: failed to get RTSP stream info\n");
return -1;
}
// 查找视频流
for (int i = 0; i < pFormatCtx->nb_streams; i++) {
if (pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
break;
}
}
if (videoStreamIndex == -1) {
printf("Error: failed to find video stream\n");
return -1;
}
// 获取视频流编解码器
pCodecCtx = avcodec_alloc_context3(NULL);
if (pCodecCtx == NULL) {
printf("Error: failed to allocate codec context\n");
return -1;
}
avcodec_parameters_to_context(pCodecCtx, pFormatCtx->streams[videoStreamIndex]->codecpar);
pCodec = avcodec_find_decoder(pCodecCtx->codec_id);
if (pCodec == NULL) {
printf("Error: failed to find codec\n");
return -1;
}
if (avcodec_open2(pCodecCtx, pCodec, NULL) < 0) {
printf("Error: failed to open codec\n");
return -1;
}
// 创建AVFrame对象
pFrame = av_frame_alloc();
if (pFrame == NULL) {
printf("Error: failed to allocate frame\n");
return -1;
}
// 创建AVFrame对象用于保存RGB图像
pFrameRGB = av_frame_alloc();
if (pFrameRGB == NULL) {
printf("Error: failed to allocate RGB frame\n");
return -1;
}
numBytes = av_image_get_buffer_size(AV_PIX_FMT_RGB24, IMAGE_WIDTH, IMAGE_HEIGHT, 1);
buffer = (uint8_t*)av_malloc(numBytes * sizeof(uint8_t));
av_image_fill_arrays(pFrameRGB->data, pFrameRGB->linesize, buffer, AV_PIX_FMT_RGB24, IMAGE_WIDTH, IMAGE_HEIGHT, 1);
// 创建SwsContext对象用于像素格式转换
img_convert_ctx = sws_getContext(pCodecCtx->width, pCodecCtx->height, pCodecCtx->pix_fmt, IMAGE_WIDTH, IMAGE_HEIGHT, AV_PIX_FMT_RGB24, SWS_BICUBIC, NULL, NULL, NULL);
if (img_convert_ctx == NULL) {
printf("Error: failed to create SwsContext\n");
return -1;
}
// 读取视频帧并保存为图片
while (av_read_frame(pFormatCtx, &packet) >= 0) {
if (packet.stream_index == videoStreamIndex) {
// 解码视频帧
avcodec_decode_video2(pCodecCtx, pFrame, &frameFinished, &packet);
if (frameFinished) {
// 转换像素格式
sws_scale(img_convert_ctx, (const uint8_t * const *)pFrame->data, pFrame->linesize, 0, pCodecCtx->height, pFrameRGB->data, pFrameRGB->linesize);
// 保存为JPEG图片
struct jpeg_compress_struct cinfo;
struct jpeg_error_mgr jerr;
JSAMPROW row_pointer[1];
int row_stride;
FILE *outfile = fopen(jpgFilename, "wb");
if (outfile == NULL) {
printf("Error: failed to open file %s\n", jpgFilename);
return -1;
}
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_compress(&cinfo);
jpeg_stdio_dest(&cinfo, outfile);
cinfo.image_width = IMAGE_WIDTH;
cinfo.image_height = IMAGE_HEIGHT;
cinfo.input_components = 3;
cinfo.in_color_space = JCS_RGB;
jpeg_set_defaults(&cinfo);
jpeg_set_quality(&cinfo, 80, TRUE);
jpeg_start_compress(&cinfo, TRUE);
row_stride = IMAGE_WIDTH * 3;
while (cinfo.next_scanline < cinfo.image_height) {
row_pointer[0] = &buffer[cinfo.next_scanline * row_stride];
(void)jpeg_write_scanlines(&cinfo, row_pointer, 1);
}
jpeg_finish_compress(&cinfo);
fclose(outfile);
}
}
av_packet_unref(&packet);
}
// 释放资源
av_free(buffer);
av_frame_free(&pFrameRGB);
av_frame_free(&pFrame);
avcodec_close(pCodecCtx);
avformat_close_input(&pFormatCtx);
sws_freeContext(img_convert_ctx);
curl_easy_cleanup(curl);
return 0;
}
```
上述代码通过libcurl库连接海康网络摄像头的RTSP流并获取视频流数据,使用FFmpeg库将视频流数据解码为图像数据,再使用libjpeg库将图像数据保存为JPG格式的图片。需要注意的是,保存图片的过程在视频帧解码完成后进行,否则保存的图片会是一张黑屏。
阅读全文
相关推荐
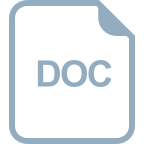
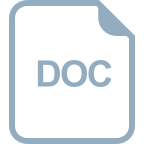
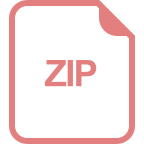
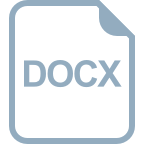
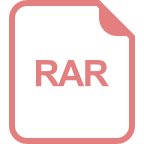
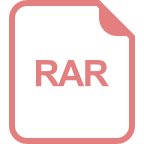
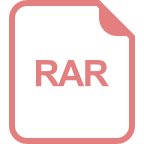
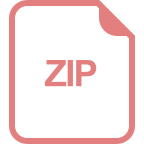
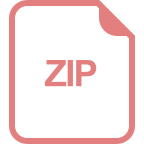
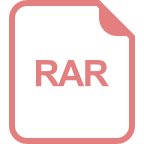
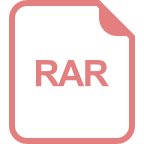
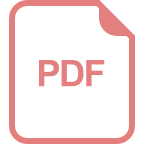
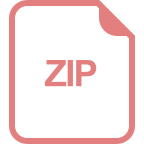
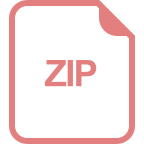
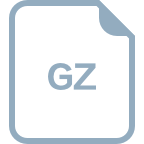
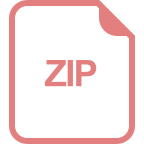
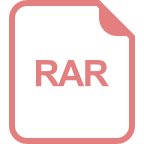