Java通过Aggregation和lookup进行多字段匹配连表
时间: 2024-02-01 11:15:12 浏览: 39
Aggregation和lookup是MongoDB中的两个操作符,用于进行多字段匹配连表查询。在Java中可以使用MongoDB的Java Driver库来实现这些操作。
首先,我们需要创建一个MongoClient实例,并选择要操作的数据库和集合:
```java
MongoClient mongoClient = new MongoClient("localhost", 27017);
MongoCollection<Document> ordersCollection = mongoClient.getDatabase("mydb").getCollection("orders");
```
接下来,我们可以使用Aggregation操作符来进行多字段匹配查询。例如,我们可以根据订单号和客户ID来查询订单项:
```java
List<Bson> pipeline = Arrays.asList(
Aggregates.match(Filters.eq("customer_id", customer_id)),
Aggregates.lookup("order_items", "order_id", "_id", "items"),
Aggregates.unwind("$items"),
Aggregates.match(Filters.eq("items.product_id", product_id))
);
AggregateIterable<Document> result = ordersCollection.aggregate(pipeline);
```
在这个例子中,我们首先使用match操作符来筛选出客户ID为指定值的订单。然后,我们使用lookup操作符来连接order_items集合,并将它们映射到订单项数组中。接下来,我们使用unwind操作符将订单项数组展开为单独的文档对象,并使用match操作符来筛选出指定产品ID的订单项。
另外,我们也可以使用lookup操作符来进行多字段匹配查询。例如,我们可以根据订单项中的产品ID和供应商ID来查询产品信息:
```java
List<Bson> pipeline = Arrays.asList(
Aggregates.match(Filters.eq("supplier_id", supplier_id)),
Aggregates.lookup("products", "product_id", "_id", "product"),
Aggregates.unwind("$product"),
Aggregates.match(Filters.and(
Filters.eq("product.product_id", product_id),
Filters.eq("product.supplier_id", supplier_id)
))
);
AggregateIterable<Document> result = ordersCollection.aggregate(pipeline);
```
在这个例子中,我们首先使用match操作符来筛选出供应商ID为指定值的订单项。然后,我们使用lookup操作符来连接products集合,并将它们映射到产品文档对象中。接下来,我们使用unwind操作符将产品文档对象展开为单独的文档对象,并使用match操作符来筛选出指定产品ID和供应商ID的产品信息。
相关推荐
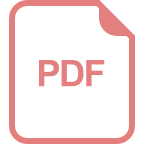
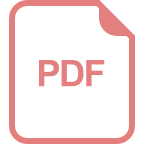














