matlab中id3
时间: 2023-08-26 18:14:02 浏览: 72
ID3(Iterative Dichotomiser 3)是一种经典的决策树生成算法之一,用于处理分类问题。在 MATLAB 中,可以使用 Statistics and Machine Learning Toolbox 中的 `fitctree` 函数来实现 ID3 决策树的生成。
具体实现步骤如下:
1. 准备数据集,将其分为训练集和测试集。
2. 使用 `fitctree` 函数来生成决策树模型。其中,需要指定训练数据集和相应的标签。
3. 可以使用 `view` 函数来可视化生成的决策树模型。
4. 使用测试数据集来评估模型的性能。
下面是一个简单的示例代码:
```matlab
% 准备数据集
load fisheriris
X = meas(:,1:2);
Y = categorical(species);
% 将数据集分为训练集和测试集
cv = cvpartition(Y,'holdout',0.2);
X_train = X(training(cv),:);
Y_train = Y(training(cv));
X_test = X(test(cv),:);
Y_test = Y(test(cv));
% 生成决策树模型
tree = fitctree(X_train,Y_train);
% 可视化决策树
view(tree,'Mode','graph')
% 使用测试数据集评估模型性能
Y_pred = predict(tree,X_test);
accuracy = sum(Y_pred == Y_test)/numel(Y_test);
disp(['Accuracy: ', num2str(accuracy)])
```
在上面的示例代码中,我们使用了鸢尾花数据集进行训练和测试,生成了一个二维特征的决策树模型,并计算了模型在测试数据集上的准确率。
相关推荐
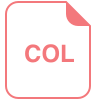
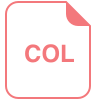
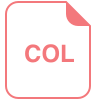
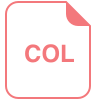
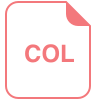









