遍历Excel表格中的关键词,如果doc格式文件中有这些关键词的一个,就输出该信息分类为服装行业,直接写python代码
时间: 2023-02-07 08:02:22 浏览: 75
您好,我可以为您提供一份基本的代码,请注意这份代码仅供参考,因为您的需求并没有很清楚的描述。
首先,您需要安装 openpyxl 库来读取 Excel 表格。这可以通过运行以下命令来完成:
```
pip install openpyxl
```
然后,您可以使用以下代码来遍历 Excel 表格中的每一行:
```python
import openpyxl
# 读取 Excel 文件
wb = openpyxl.load_workbook('keywords.xlsx')
# 选择第一个表单
sheet = wb.active
# 遍历每一行
for row in sheet.rows:
# 获取单元格内容
keyword = row[0].value
# 判断关键词是否在 doc 文件中出现
if keyword_in_doc(keyword):
print(f'{keyword} 属于服装行业')
```
需要注意的是,上述代码中的 `keyword_in_doc()` 函数尚未实现。您需要自行实现这个函数来检查关键词是否在 doc 文件中出现。
希望这份代码能够帮助您开始编写您的程序。如果您有任何其他问题,请随时告诉我。
相关问题
遍历Excel表格中的关键词,如果doc格式文件中有这些关键词的一个,就输出txt格式文件内容为:该信息分类为服装行业,直接写python代码
### 回答1:
您可以使用 Python 中的 xlrd 库来遍历 Excel 表格中的关键词。使用 python-docx 库来检查 doc 格式文件中是否有这些关键词。如果 doc 文件中有这些关键词之一,就使用 Python 的 open() 函数来写入 txt 文件中。
下面是一个例子:
```
import xlrd
import docx
# 打开 Excel 文件
workbook = xlrd.open_workbook('keywords.xlsx')
# 选择第一个工作表
worksheet = workbook.sheet_by_index(0)
# 遍历工作表中的每一行
for row in range(worksheet.nrows):
# 获取第一列的关键词
keyword = worksheet.cell(row, 0).value
# 打开 doc 文件
doc = docx.Document('document.docx')
# 检查 doc 文件中是否包含关键词
if keyword in doc.paragraphs:
# 如果有,写入 txt 文件
with open('output.txt', 'w') as f:
f.write('该信息分类为服装行业')
```
希望这能帮到您!
### 回答2:
import xlrd
import os
def search_keywords():
# 打开Excel表格
workbook = xlrd.open_workbook('keywords.xlsx')
sheet = workbook.sheet_by_index(0)
# 获取关键词列表
keywords = sheet.col_values(0)
# 遍历doc格式文件夹中的文件
doc_folder = 'doc_folder' # doc格式文件所在的文件夹路径
txt_folder = 'txt_folder' # 输出txt文件所在的文件夹路径
for filename in os.listdir(doc_folder):
if filename.endswith('.doc'):
doc_path = os.path.join(doc_folder, filename)
with open(doc_path, 'r') as f:
content = f.read()
# 检查是否包含关键词
for keyword in keywords:
if keyword in content:
# 输出txt文件
txt_path = os.path.join(txt_folder, filename.split('.')[0]+'.txt')
with open(txt_path, 'w') as txt_file:
txt_file.write('该信息分类为服装行业')
break # 只输出一个txt文件即可,跳出当前循环
search_keywords()
### 回答3:
使用Python可以通过openpyxl库和python-docx库来实现遍历Excel表格中的关键词,并判断doc格式文件中是否包含这些关键词。
首先需要安装openpyxl和python-docx库:
```
pip install openpyxl
pip install python-docx
```
然后编写如下Python代码实现遍历Excel表格和判断doc格式文件:
```python
import openpyxl
import docx
# 读取Excel表格中的关键词
def read_keywords_from_excel(filename):
keywords = []
workbook = openpyxl.load_workbook(filename)
worksheet = workbook.active
for row in worksheet.iter_rows():
for cell in row:
keywords.append(cell.value)
workbook.close()
return keywords
# 判断doc格式文件是否包含关键词
def has_keyword_in_doc(keyword, filename):
doc = docx.Document(filename)
for paragraph in doc.paragraphs:
if keyword in paragraph.text:
return True
return False
# 输出txt格式文件内容为特定信息分类
def output_text_content(filename, content):
with open(filename, 'w') as file:
file.write(content)
if __name__ == '__main__':
excel_filename = 'keywords.xlsx'
doc_filename = 'document.docx'
txt_filename = 'output.txt'
keywords = read_keywords_from_excel(excel_filename)
for keyword in keywords:
if has_keyword_in_doc(keyword, doc_filename):
output_text_content(txt_filename, '该信息分类为服装行业')
break
```
需要将Excel表格中的关键词存储在名为keywords.xlsx的文件中,doc格式文件存储在名为document.docx的文件中,输出的txt格式文件名为output.txt。
以上代码将遍历Excel表格中的关键词,并检查doc格式文件中是否存在这些关键词,如果其中一个关键词存在,则将输出的txt文件内容设为“该信息分类为服装行业”。如果多个关键词都存在,则只输出一次该信息分类。
从doc文件中抽取含有多个关键词的句子,将抽取的句子保存到txt文件,关键词从Excel表格中匹配,直接写python完整代码
首先,您需要安装 Python 中的 docx 库,这样才能读取 docx 文件。然后,您可以使用以下代码来实现您的需求:
```python
import docx
import openpyxl
# 读取 docx 文件
doc = docx.Document('file.docx')
# 读取 Excel 表格中的关键词
excel_wb = openpyxl.load_workbook('keywords.xlsx')
sheet = excel_wb['Sheet1']
keywords = [cell.value for row in sheet.rows for cell in row]
# 打开一个 txt 文件,用于保存抽取的句子
with open('extracted_sentences.txt', 'w') as f:
# 遍历 docx 中的每一个段落
for paragraph in doc.paragraphs:
# 将段落文本拆分成单独的词
words = paragraph.text.split()
# 遍历每一个词
for word in words:
# 如果该词是关键词,则保存整个段落到 txt 文件
if word in keywords:
f.write(paragraph.text)
f.write('\n')
break
```
这段代码首先会读取 Excel 表格中的关键词,然后打开一个 txt 文件,并遍历 docx 文件中的每一个段落。对于每一个段落,它会将文本拆分成单独的词,并遍历每一个词。如果该词是关键词,则会将整个段落保存到 txt 文件中。
相关推荐
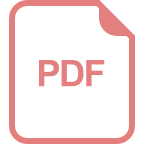







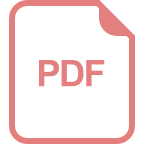
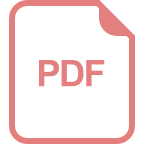
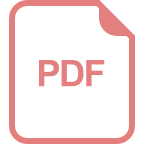
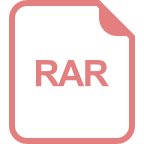
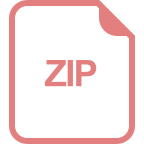
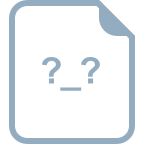