Qt打开固定路径下的所有的.xlsx文件,并且读取每个文件的表格中是否含有“时间”字符,如果有打印位置到控件text Browser中
时间: 2024-03-17 09:41:31 浏览: 21
好的,我可以帮你解决这个问题。首先,你需要使用Qt的文件操作类QDir和QFile,遍历指定路径下的所有.xlsx文件,并读取每个文件的表格内容。然后,你需要使用Qt的QXlsx类来解析Excel文件,并查找表格中是否包含“时间”字符。
以下是实现此功能的示例代码:
```cpp
#include <QDir>
#include <QFile>
#include <QTextBrowser>
#include <QXlsx/xlsxdocument.h>
void readExcelFiles(QString path, QTextBrowser* textBrowser)
{
QDir dir(path);
QStringList filters;
filters << "*.xlsx"; // 只遍历.xlsx文件
dir.setNameFilters(filters);
QStringList fileList = dir.entryList();
foreach(QString file, fileList) {
QString filePath = path + "/" + file;
QFile qFile(filePath);
if (!qFile.open(QIODevice::ReadOnly)) {
qDebug() << "Can't open file: " << filePath;
continue;
}
QXlsx::Document xlsx(&qFile);
QList<QXlsx::Worksheet*> sheets = xlsx.worksheets();
foreach(QXlsx::Worksheet* sheet, sheets) {
QXlsx::CellRange range = sheet->dimension();
for (int i = range.firstRow(); i <= range.lastRow(); i++) {
for (int j = range.firstColumn(); j <= range.lastColumn(); j++) {
QXlsx::Cell* cell = sheet->cellAt(i, j);
if (cell && cell->dataType() == QXlsx::Cell::SharedStringType) {
QString text = cell->value().toString();
if (text.contains("时间")) {
QString position = QString("%1:%2").arg(i).arg(j);
textBrowser->append(filePath + " : " + position);
}
}
}
}
}
qFile.close();
}
}
```
你可以在你的Qt项目中创建一个按钮或其他控件来调用`readExcelFiles()`函数,并将路径和textBrowser对象作为参数传递。例如:
```cpp
void MainWindow::on_pushButton_clicked()
{
QString path = "/your/excel/files/path";
readExcelFiles(path, ui->textBrowser);
}
```
注意,这个代码示例只是一个简单的演示,如果你需要处理大量的Excel文件,你可能需要使用多线程来加快处理速度。
相关推荐
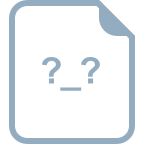









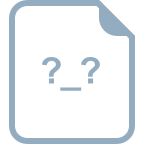
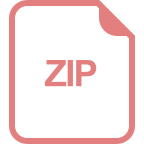
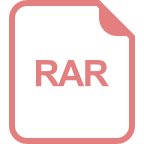
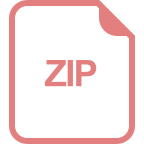
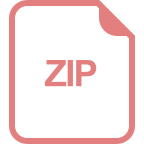