vue如何封装全局方法
时间: 2023-06-23 20:50:39 浏览: 370
Vue可以通过Vue.prototype对象来封装全局方法。具体步骤如下:
1. 在src目录下新建一个utils文件夹,用于存放全局方法的封装文件。
2. 在utils文件夹下新建一个index.js文件,用于封装全局方法。
3. 在index.js文件中定义全局方法,例如:
```
export const myGlobalMethod = function () {
// 方法逻辑
}
```
4. 在main.js文件中引入index.js文件,并通过Vue.prototype对象将方法挂载到Vue实例上,例如:
```
import Vue from 'vue'
import App from './App.vue'
import { myGlobalMethod } from './utils'
Vue.config.productionTip = false
Vue.prototype.$myGlobalMethod = myGlobalMethod
new Vue({
render: h => h(App),
}).$mount('#app')
```
5. 在组件中可以通过this.$myGlobalMethod()来调用全局方法。
注意:封装全局方法时需要注意方法的命名,避免与其它全局方法或Vue内置方法名称冲突。
相关问题
vue封装全局方法,数字大于1000小于10000,显示千
可以在 Vue 的 `prototype` 对象上添加一个方法,将数字转换为千位数,代码如下:
```javascript
Vue.prototype.formatNumber = function(num) {
if (num >= 1000 && num < 10000) {
return (num / 1000).toFixed(1) + '千';
} else {
return num;
}
}
```
然后在组件中就可以使用这个全局方法了:
```html
<template>
<div>{{ formatNumber(2500) }}</div>
</template>
<script>
export default {
methods: {
formatNumber(num) {
return this.$root.formatNumber(num);
}
}
}
</script>
```
这样就可以把 `2500` 转换为 `2.5千`。
vue封装全局方法,数字大于1000小于10000,显示千,、数字大于10000小于100000000,显示万,数字大于100000000,显示亿,千级别: 不保留小数点;万级别: 保留小数点后两位,亿级别: 保留小数点后三位
你可以在 Vue 的原型上挂载一个全局方法,例如:
```javascript
Vue.prototype.formatNumber = function (num) {
if (num >= 100000000) {
return (num / 100000000).toFixed(3) + '亿'
} else if (num >= 10000) {
return (num / 10000).toFixed(2) + '万'
} else if (num >= 1000) {
return Math.floor(num / 1000) + '千'
} else {
return num.toString()
}
}
```
然后在组件中就可以使用这个全局方法了:
```html
<template>
<div>{{ formatNumber(123456789) }}</div>
</template>
<script>
export default {
methods: {
formatNumber(num) {
return this.$root.formatNumber(num)
}
}
}
</script>
```
注意,这里的千级别是不保留小数点,如果想要保留一位小数,可以将 `Math.floor` 改为 `num.toFixed(1)`,同理,万级别和亿级别也可以根据需求调整保留小数点的位数。
阅读全文
相关推荐
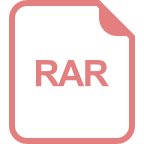
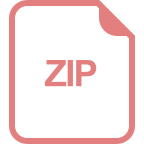
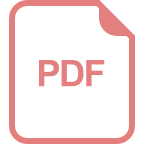

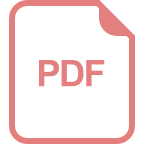







