使用c语言编写程序:已知dat1.dat存放了一系列整型数据。 要求: 1)用dat1.dat中的前100个数据给数组int a[100]赋值,并在屏幕上输出(每行10个元素)。 2)使用函数 double isaver(int *p,int num) { } 求数组a中所有数组元素平均值。 3)把该函数放在头文件ISaver.h中以便在主函数中调用该函数。把所有小于平均值的数组元素(每行10个元素)和小于平均值的数组元素个数输出出来。(在屏幕上和数据文件d:\dat6.dat中同时输出)
时间: 2023-12-10 19:38:05 浏览: 101
以下是使用C语言编写的程序实现所述功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include "ISaver.h"
#define SIZE 100 // 数组大小
int main()
{
FILE *fp1, *fp2;
int a[SIZE];
double average;
int count = 0;
// 打开数据文件dat1.dat
if ((fp1 = fopen("dat1.dat", "rb")) == NULL)
{
printf("Cannot open file dat1.dat\n");
exit(1);
}
// 读取前100个数据到数组a中并输出
printf("The first 100 numbers in dat1.dat:\n");
for (int i = 0; i < SIZE; i++)
{
fread(&a[i], sizeof(int), 1, fp1);
if (i % 10 == 0)
printf("\n");
printf("%d ", a[i]);
}
printf("\n");
// 关闭数据文件dat1.dat
fclose(fp1);
// 计算数组a的平均值
average = isaver(a, SIZE);
// 打开数据文件dat6.dat
if ((fp2 = fopen("dat6.dat", "w")) == NULL)
{
printf("Cannot open file dat6.dat\n");
exit(1);
}
// 输出小于平均值的数组元素和个数到屏幕和文件中
printf("Numbers below the average (%.2f):\n", average);
for (int i = 0; i < SIZE; i++)
{
if (a[i] < average)
{
if (count % 10 == 0)
printf("\n");
printf("%d ", a[i]);
fprintf(fp2, "%d ", a[i]);
count++;
}
}
printf("\nTotal number of elements below the average: %d\n", count);
fprintf(fp2, "\nTotal number of elements below the average: %d\n", count);
// 关闭数据文件dat6.dat
fclose(fp2);
return 0;
}
```
其中ISaver.h头文件中定义了函数`isaver`的函数原型:
```c
double isaver(int *p, int num);
```
函数的实现如下:
```c
#include "ISaver.h"
double isaver(int *p, int num)
{
double sum = 0;
for (int i = 0; i < num; i++)
sum += p[i];
return sum / num;
}
```
注意,上述程序中使用了二进制读写文件("rb"/"w")而非文本读写文件("r"/"w")。另外,由于涉及到文件操作,需要在文件操作完成后关闭文件,以释放文件资源,避免资源泄漏。
阅读全文
相关推荐








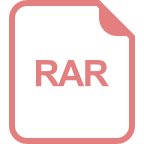
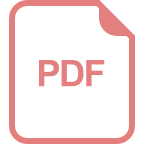








