在FISCO-BCOS智能合约中使用SM4算法源码
时间: 2024-05-04 13:19:09 浏览: 90
FISCO-BCOS智能合约支持使用C++编写智能合约,因此可以在智能合约中使用SM4算法的C++源码实现。以下是一个简单的示例:
```cpp
#include <openssl/evp.h>
#include <string.h>
extern "C" {
#include "sm4.h"
}
// 使用OpenSSL库实现SM4算法加密
std::string sm4_encrypt_openssl(const std::string& key, const std::string& iv, const std::string& data) {
EVP_CIPHER_CTX *ctx = EVP_CIPHER_CTX_new();
EVP_CIPHER_CTX_init(ctx);
// 设置加密算法和模式
EVP_EncryptInit_ex(ctx, EVP_sms4_cbc(), NULL, (const unsigned char*)key.c_str(), (const unsigned char*)iv.c_str());
// 执行加密操作并获取加密后的数据
int len = data.length();
int ciphertext_len = len + EVP_MAX_BLOCK_LENGTH;
unsigned char* ciphertext = new unsigned char[ciphertext_len];
int outlen;
EVP_EncryptUpdate(ctx, ciphertext, &outlen, (const unsigned char*)data.c_str(), len);
ciphertext_len = outlen;
EVP_EncryptFinal_ex(ctx, ciphertext + outlen, &outlen);
ciphertext_len += outlen;
// 转换为十六进制字符串返回
std::string result;
for (int i=0; i<ciphertext_len; i++) {
char buf[3];
snprintf(buf, sizeof(buf), "%02x", ciphertext[i]);
result.append(buf);
}
delete[] ciphertext;
return result;
}
// 使用国密SM4算法加密
std::string sm4_encrypt_sm(const std::string& key, const std::string& iv, const std::string& data) {
unsigned char* plaintext = new unsigned char[data.length() + 1];
strcpy((char*)plaintext, data.c_str());
// 加密
unsigned char* ciphertext = new unsigned char[data.length() + 1];
memset(ciphertext, 0, data.length() + 1);
unsigned char* tmp_key = new unsigned char[key.length() + 1];
strcpy((char*)tmp_key, key.c_str());
unsigned char* tmp_iv = new unsigned char[iv.length() + 1];
strcpy((char*)tmp_iv, iv.c_str());
sm4_context ctx;
sm4_setkey_enc(&ctx, tmp_key);
sm4_crypt_cbc(&ctx, SM4_ENCRYPT, data.length(), tmp_iv, plaintext, ciphertext);
// 转换为十六进制字符串返回
std::string result;
for (int i=0; i<data.length(); i++) {
char buf[3];
snprintf(buf, sizeof(buf), "%02x", ciphertext[i]);
result.append(buf);
}
delete[] plaintext;
delete[] ciphertext;
delete[] tmp_key;
delete[] tmp_iv;
return result;
}
```
其中,`sm4_encrypt_openssl`使用OpenSSL库实现SM4算法加密,`sm4_encrypt_sm`使用国密SM4算法加密。在使用国密SM4算法加密时,需要包含国密SM4算法的头文件,并调用其API实现加密操作。
请注意,由于国密SM4算法的限制,其密钥长度必须为16个字节,IV长度必须为16个字节。在实际使用时,需要根据实际情况生成合适的密钥和IV。
阅读全文
相关推荐
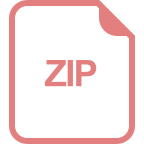
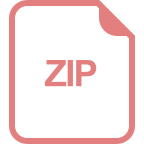
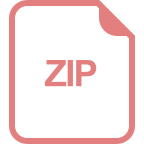
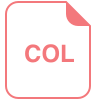
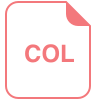
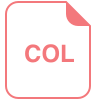

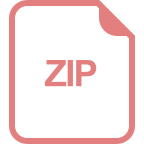
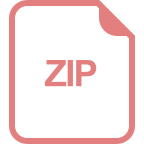
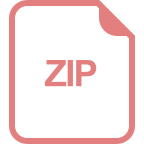
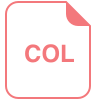
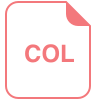
