绘制横向条状显示整个磁盘,并显示分区大小;支持鼠标拖动控制分区容量大小,同时支持输入大小精准控制分区大小
时间: 2023-06-18 14:03:57 浏览: 109
这个功能可以通过使用Python的Tkinter库来实现。下面是一个简单的示例代码,可以绘制一个横向条状图,并且支持通过鼠标拖动和输入来控制分区大小:
```python
import tkinter as tk
class DiskPartition:
def __init__(self, size, color):
self.size = size
self.color = color
class DiskPartitionGUI:
def __init__(self, master, partitions):
self.master = master
self.partitions = partitions
self.width = 400
self.height = 50
self.canvas = tk.Canvas(master, width=self.width, height=self.height)
self.canvas.pack()
self.canvas.bind("<Button-1>", self.on_click)
self.canvas.bind("<B1-Motion>", self.on_drag)
self.canvas.bind("<ButtonRelease-1>", self.on_release)
self.draw()
def draw(self):
total_size = sum(p.size for p in self.partitions)
x0 = 0
for p in self.partitions:
x1 = x0 + self.width * p.size / total_size
self.canvas.create_rectangle(x0, 0, x1, self.height, fill=p.color, width=0)
x0 = x1
def on_click(self, event):
self.dragging = True
self.x0 = event.x
def on_drag(self, event):
if self.dragging:
x = max(0, min(self.width, event.x))
dx = x - self.x0
total_size = sum(p.size for p in self.partitions)
p0 = self.partitions[0]
p1 = self.partitions[-1]
if dx > 0 and p1.size + dx > total_size:
dx = total_size - p1.size
elif dx < 0 and p0.size - dx < 0:
dx = p0.size
p0.size -= dx
p1.size += dx
self.x0 = x
self.canvas.delete("all")
self.draw()
def on_release(self, event):
self.dragging = False
def main():
partitions = [
DiskPartition(100, "red"),
DiskPartition(200, "green"),
DiskPartition(50, "blue")
]
root = tk.Tk()
gui = DiskPartitionGUI(root, partitions)
root.mainloop()
if __name__ == "__main__":
main()
```
这个代码会创建一个包含三个分区的磁盘条状图,并且可以通过鼠标拖动来调整分区大小。你可以根据自己的需要修改代码来添加更多的分区和自定义颜色。
阅读全文
相关推荐
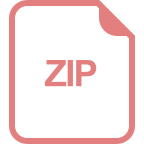
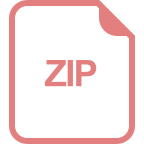
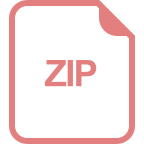
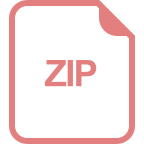
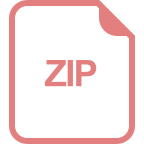
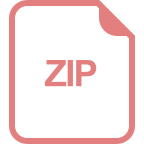
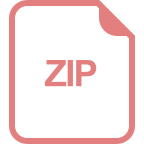
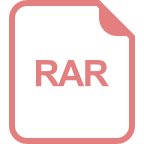
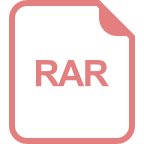
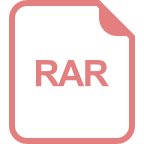
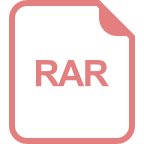
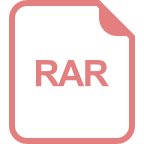
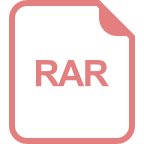
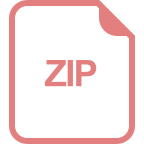
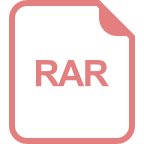