优化代码 var userIdentity = await _userIdentityManager.GetDetail(userId); var staffInfo = await _enterpriseStaffManager.GetDetail(userId); if (userIdentity != null) { infoOutput.IdentityType = userIdentity.IdentityType; infoOutput.IsSelected = userIdentity.IsSelected; } if (staffInfo != null) { infoOutput.Code = staffInfo.EnterpriseCode; infoOutput.UserStatus = EnterpriseUserStatus.Authenticated; infoOutput.Level = (AppStaffLevelType)staffInfo.Level; } else { var curCreate = await _applicationManager.GetCreate(userId); var auditInfo = await _enterpriseAuditManager.GetNewAuditData(userId); infoOutput.UserStatus = GetAuditStateType(auditInfo); if (curCreate != null) { if (curCreate.Status == EnterpriseCreationApplicationStatus.UnderReview) { infoOutput.UserStatus = EnterpriseUserStatus.CreateAudit; } else if (auditInfo?.AuditState == EnterpriseAuditStateType.EmployeeRejected && curCreate.UpdatedDate > auditInfo?.UpdatedDate) { infoOutput.UserStatus = EnterpriseUserStatus.CreateFail; } } }
时间: 2024-02-10 07:14:25 浏览: 80
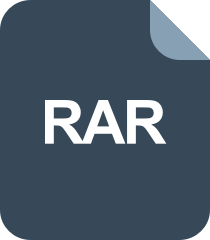
async_await_wait_result.rar
You can optimize the code by using the null-conditional operator (?.) and the null-coalescing operator (??) to simplify the nested if statements and reduce the amount of duplicated code. Here's an example:
```
var userIdentity = await _userIdentityManager.GetDetail(userId);
var staffInfo = await _enterpriseStaffManager.GetDetail(userId);
infoOutput.IdentityType = userIdentity?.IdentityType;
infoOutput.IsSelected = userIdentity?.IsSelected;
if (staffInfo != null)
{
infoOutput.Code = staffInfo.EnterpriseCode;
infoOutput.UserStatus = EnterpriseUserStatus.Authenticated;
infoOutput.Level = (AppStaffLevelType)staffInfo.Level;
}
else
{
var curCreate = await _applicationManager.GetCreate(userId);
var auditInfo = await _enterpriseAuditManager.GetNewAuditData(userId);
infoOutput.UserStatus = GetAuditStateType(auditInfo) ??
(curCreate?.Status == EnterpriseCreationApplicationStatus.UnderReview ?
EnterpriseUserStatus.CreateAudit :
(auditInfo?.AuditState == EnterpriseAuditStateType.EmployeeRejected && curCreate.UpdatedDate > auditInfo?.UpdatedDate ?
EnterpriseUserStatus.CreateFail :
infoOutput.UserStatus));
}
```
This code first uses the null-conditional operator to check if `userIdentity` is not null before setting the properties of `infoOutput`. Then, it checks if `staffInfo` is not null to set the remaining properties of `infoOutput`. If `staffInfo` is null, it uses the null-coalescing operator to check if `auditInfo` is not null and call `GetAuditStateType` to determine the user status. If `auditInfo` is null, it checks if `curCreate` is not null and if its status is `UnderReview` to set the user status to `CreateAudit`. If `auditInfo` is not null, it checks if its `AuditState` is `EmployeeRejected` and if `curCreate` has been updated since the last audit date to set the user status to `CreateFail`. If none of these conditions are met, it keeps the current user status.
阅读全文
相关推荐
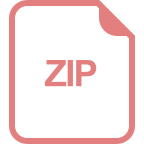
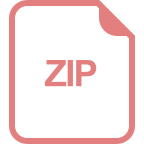
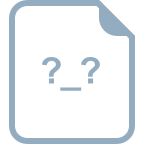
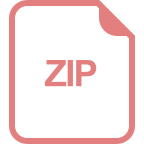
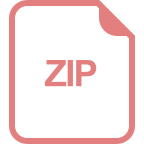
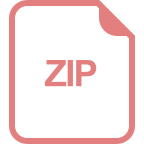
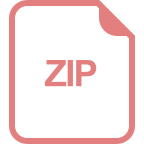
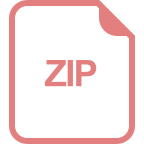
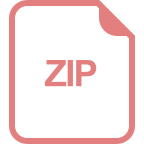
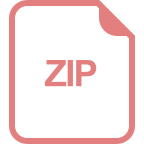
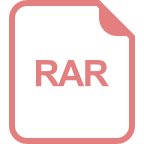
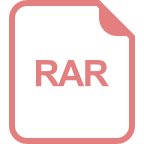
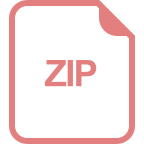
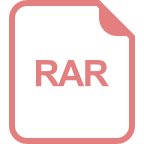
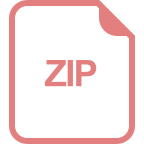
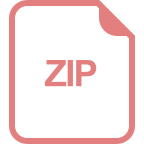
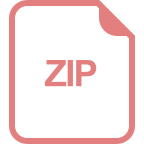
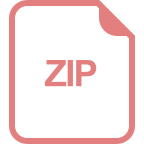