gobject的g_object_new_with_properties使用示例
时间: 2023-07-29 11:14:37 浏览: 222
g_object_new_with_properties 是一个创建 GObject 对象的函数,它可以使用属性列表来设置对象的属性。以下是一个示例:
```c
#include <glib-object.h>
typedef struct _MyObject MyObject;
struct _MyObject {
GObject parent_instance;
gint int_prop;
gchar* string_prop;
};
enum {
PROP_INT_PROP = 1,
PROP_STRING_PROP,
N_PROPERTIES
};
static GParamSpec* obj_properties[N_PROPERTIES] = { NULL, };
static void my_object_set_property(GObject* object, guint prop_id, const GValue* value, GParamSpec* pspec)
{
MyObject* self = MY_OBJECT(object);
switch (prop_id) {
case PROP_INT_PROP:
self->int_prop = g_value_get_int(value);
break;
case PROP_STRING_PROP:
g_free(self->string_prop);
self->string_prop = g_strdup(g_value_get_string(value));
break;
default:
G_OBJECT_WARN_INVALID_PROPERTY_ID(object, prop_id, pspec);
break;
}
}
static void my_object_get_property(GObject* object, guint prop_id, GValue* value, GParamSpec* pspec)
{
MyObject* self = MY_OBJECT(object);
switch (prop_id) {
case PROP_INT_PROP:
g_value_set_int(value, self->int_prop);
break;
case PROP_STRING_PROP:
g_value_set_string(value, self->string_prop);
break;
default:
G_OBJECT_WARN_INVALID_PROPERTY_ID(object, prop_id, pspec);
break;
}
}
static void my_object_class_init(MyObjectClass* klass)
{
GObjectClass* object_class = G_OBJECT_CLASS(klass);
object_class->set_property = my_object_set_property;
object_class->get_property = my_object_get_property;
obj_properties[PROP_INT_PROP] = g_param_spec_int("int-prop",
"Int property",
"An integer property",
G_MININT,
G_MAXINT,
0,
G_PARAM_READWRITE);
obj_properties[PROP_STRING_PROP] = g_param_spec_string("string-prop",
"String property",
"A string property",
"",
G_PARAM_READWRITE);
g_object_class_install_properties(object_class, N_PROPERTIES, obj_properties);
}
static void my_object_init(MyObject* self)
{
self->int_prop = 0;
self->string_prop = g_strdup("");
}
int main(int argc, char** argv)
{
g_type_init();
MyObject* obj = g_object_new_with_properties(MY_TYPE_OBJECT,
"int-prop", 42,
"string-prop", "Hello, world!",
NULL);
g_object_unref(obj);
return 0;
}
```
这个示例定义了一个名为 MyObject 的 GObject 子类,它包含一个整数属性 int_prop 和一个字符串属性 string_prop。在 my_object_class_init 函数中,我们使用 g_param_spec_int 和 g_param_spec_string 函数来定义这些属性的参数规范。然后,我们使用 g_object_class_install_properties 将这些属性添加到对象类中。
在 my_object_set_property 和 my_object_get_property 函数中,我们实现了属性的设置和获取方法。在 main 函数中,我们使用 g_object_new_with_properties 函数创建一个 MyObject 实例,并设置 int_prop 和 string_prop 属性的值。最后,我们必须调用 g_object_unref 函数来释放对象的引用。
阅读全文
相关推荐
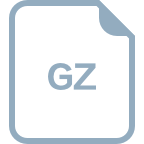
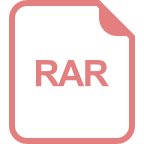
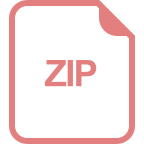










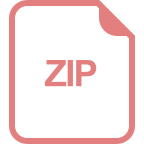




