实验内容(附源代码和运行结果的截图)自行设计一个数据库项目,分别采用Druid、Mybaties、JDBCTemplate的整合技术,通过定义xml依赖文件和properties属性配置文件,定义实体类,编写持久层、Service层、controller层,完成系统的CRUD功能。
时间: 2024-01-21 20:17:55 浏览: 117
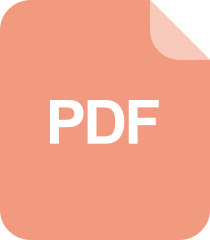
Spring MVC配置双数据源实现一个java项目同时连接两个数据库的方法
很好,这是一个非常实用的项目。我可以给您提供一些思路和参考代码。
首先,我们需要定义一个实体类,例如一个学生类:
```java
public class Student {
private int id;
private String name;
private int age;
// 省略getter和setter方法
}
```
接下来,我们需要编写持久层的代码。我们可以使用JDBCTemplate来操作数据库。在这个例子中,我们创建一个名为StudentDao的接口,包含了增删改查的方法:
```java
public interface StudentDao {
public void addStudent(Student student);
public void updateStudent(Student student);
public void deleteStudent(int id);
public Student getStudentById(int id);
public List<Student> getAllStudents();
}
```
然后我们可以实现这个接口,在实现类中使用JDBCTemplate来进行数据库操作:
```java
public class StudentDaoImpl implements StudentDao {
private JdbcTemplate jdbcTemplate;
public void setDataSource(DataSource dataSource) {
this.jdbcTemplate = new JdbcTemplate(dataSource);
}
public void addStudent(Student student) {
String sql = "INSERT INTO student (name, age) VALUES (?, ?)";
jdbcTemplate.update(sql, student.getName(), student.getAge());
}
public void updateStudent(Student student) {
String sql = "UPDATE student SET name=?, age=? WHERE id=?";
jdbcTemplate.update(sql, student.getName(), student.getAge(), student.getId());
}
public void deleteStudent(int id) {
String sql = "DELETE FROM student WHERE id=?";
jdbcTemplate.update(sql, id);
}
public Student getStudentById(int id) {
String sql = "SELECT id, name, age FROM student WHERE id=?";
return jdbcTemplate.queryForObject(sql, new Object[] { id }, new StudentMapper());
}
public List<Student> getAllStudents() {
String sql = "SELECT id, name, age FROM student";
return jdbcTemplate.query(sql, new StudentMapper());
}
private static final class StudentMapper implements RowMapper<Student> {
public Student mapRow(ResultSet rs, int rowNum) throws SQLException {
Student student = new Student();
student.setId(rs.getInt("id"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
return student;
}
}
}
```
在这个实现类中,我们使用JdbcTemplate来进行数据库操作。我们还定义了一个private static final class,它是一个内部类,用来将查询结果映射到一个Student对象。
接下来,我们需要编写Service层的代码,这里我们可以使用Spring框架来管理我们的Bean。我们创建一个名为StudentService的接口:
```java
public interface StudentService {
public void addStudent(Student student);
public void updateStudent(Student student);
public void deleteStudent(int id);
public Student getStudentById(int id);
public List<Student> getAllStudents();
}
```
然后我们可以实现这个接口,在实现类中调用StudentDao来进行数据库操作:
```java
@Service
@Transactional
public class StudentServiceImpl implements StudentService {
@Autowired
private StudentDao studentDao;
public void addStudent(Student student) {
studentDao.addStudent(student);
}
public void updateStudent(Student student) {
studentDao.updateStudent(student);
}
public void deleteStudent(int id) {
studentDao.deleteStudent(id);
}
public Student getStudentById(int id) {
return studentDao.getStudentById(id);
}
public List<Student> getAllStudents() {
return studentDao.getAllStudents();
}
}
```
在这个实现类中,我们使用了Spring框架的@Autowired注解来自动注入StudentDao对象。我们还使用了@Transactional注解来开启事务。
最后,我们需要编写Controller层的代码。我们创建一个名为StudentController的类:
```java
@Controller
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@RequestMapping(value = "/add", method = RequestMethod.POST)
public String addStudent(@ModelAttribute("student") Student student, ModelMap model) {
studentService.addStudent(student);
model.addAttribute("message", "Student added successfully");
return "redirect:/student/list";
}
@RequestMapping(value = "/update", method = RequestMethod.POST)
public String updateStudent(@ModelAttribute("student") Student student, ModelMap model) {
studentService.updateStudent(student);
model.addAttribute("message", "Student updated successfully");
return "redirect:/student/list";
}
@RequestMapping(value = "/delete/{id}", method = RequestMethod.GET)
public String deleteStudent(@PathVariable("id") int id, ModelMap model) {
studentService.deleteStudent(id);
model.addAttribute("message", "Student deleted successfully");
return "redirect:/student/list";
}
@RequestMapping(value = "/edit/{id}", method = RequestMethod.GET)
public String editStudent(@PathVariable("id") int id, ModelMap model) {
Student student = studentService.getStudentById(id);
model.addAttribute("student", student);
return "edit";
}
@RequestMapping(value = "/list", method = RequestMethod.GET)
public String listStudents(ModelMap model) {
List<Student> students = studentService.getAllStudents();
model.addAttribute("students", students);
return "list";
}
@RequestMapping(value = "/add", method = RequestMethod.GET)
public String showAddForm(ModelMap model) {
Student student = new Student();
model.addAttribute("student", student);
return "add";
}
}
```
在这个Controller中,我们使用了Spring框架的@RequestMapping注解来映射HTTP请求。我们还使用了@Autowired注解来自动注入StudentService对象。
最后,我们需要编写一些JDBC和Spring的配置文件。我们可以使用Druid作为数据源,创建一个名为db.properties的文件:
```properties
jdbc.driverClassName=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/test
jdbc.username=root
jdbc.password=root
# druid
druid.initialSize=5
druid.minIdle=5
druid.maxActive=20
druid.maxWait=60000
druid.timeBetweenEvictionRunsMillis=60000
druid.minEvictableIdleTimeMillis=300000
druid.validationQuery=SELECT 1 FROM DUAL
druid.testWhileIdle=true
druid.testOnBorrow=false
druid.testOnReturn=false
druid.poolPreparedStatements=true
druid.maxPoolPreparedStatementPerConnectionSize=20
druid.filters=stat,wall
druid.connectionProperties=druid.stat.mergeSql=true;druid.stat.slowSqlMillis=5000
```
然后我们需要创建一个名为jdbc.properties的文件,用来配置JDBC和Spring的属性:
```properties
jdbc.driverClassName=${jdbc.driverClassName}
jdbc.url=${jdbc.url}
jdbc.username=${jdbc.username}
jdbc.password=${jdbc.password}
# Spring
spring.datasource.druid.initial-size=${druid.initialSize}
spring.datasource.druid.min-idle=${druid.minIdle}
spring.datasource.druid.max-active=${druid.maxActive}
spring.datasource.druid.max-wait=${druid.maxWait}
spring.datasource.druid.time-between-eviction-runs-millis=${druid.timeBetweenEvictionRunsMillis}
spring.datasource.druid.min-evictable-idle-time-millis=${druid.minEvictableIdleTimeMillis}
spring.datasource.druid.validation-query=${druid.validationQuery}
spring.datasource.druid.test-while-idle=${druid.testWhileIdle}
spring.datasource.druid.test-on-borrow=${druid.testOnBorrow}
spring.datasource.druid.test-on-return=${druid.testOnReturn}
spring.datasource.druid.pool-prepared-statements=${druid.poolPreparedStatements}
spring.datasource.druid.max-pool-prepared-statement-per-connection-size=${druid.maxPoolPreparedStatementPerConnectionSize}
spring.datasource.druid.filters=${druid.filters}
spring.datasource.druid.connection-properties=${druid.connectionProperties}
```
最后,我们需要创建一个名为applicationContext.xml的文件,用来配置Spring的Bean:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.3.xsd">
<!-- 数据源 -->
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="${jdbc.driverClassName}" />
<property name="url" value="${jdbc.url}" />
<property name="username" value="${jdbc.username}" />
<property name="password" value="${jdbc.password}" />
<property name="initialSize" value="${druid.initialSize}" />
<property name="minIdle" value="${druid.minIdle}" />
<property name="maxActive" value="${druid.maxActive}" />
<property name="maxWait" value="${druid.maxWait}" />
<property name="timeBetweenEvictionRunsMillis" value="${druid.timeBetweenEvictionRunsMillis}" />
<property name="minEvictableIdleTimeMillis" value="${druid.minEvictableIdleTimeMillis}" />
<property name="validationQuery" value="${druid.validationQuery}" />
<property name="testWhileIdle" value="${druid.testWhileIdle}" />
<property name="testOnBorrow" value="${druid.testOnBorrow}" />
<property name="testOnReturn" value="${druid.testOnReturn}" />
<property name="poolPreparedStatements" value="${druid.poolPreparedStatements}" />
<property name="maxPoolPreparedStatementPerConnectionSize" value="${druid.maxPoolPreparedStatementPerConnectionSize}" />
<property name="filters" value="${druid.filters}" />
<property name="connectionProperties" value="${druid.connectionProperties}" />
</bean>
<!-- JdbcDaoSupport -->
<bean id="studentDao" class="com.example.dao.StudentDaoImpl">
<property name="dataSource" ref="dataSource" />
</bean>
<!-- Service -->
<bean id="studentService" class="com.example.service.StudentServiceImpl">
<property name="studentDao" ref="studentDao" />
</bean>
<!-- Controller -->
<bean id="studentController" class="com.example.controller.StudentController">
<property name="studentService" ref="studentService" />
</bean>
<!-- 视图解析器 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/" />
<property name="suffix" value=".jsp" />
</bean>
<!-- 开启注解 -->
<context:component-scan base-package="com.example" />
<mvc:annotation-driven />
</beans>
```
在这个配置文件中,我们定义了一个名为dataSource的Bean,它是一个Druid数据源。我们还定义了StudentDaoImpl、StudentServiceImpl、StudentController以及视图解析器的Bean。我们还使用了<context:component-scan>和<mvc:annotation-driven>标签来开启注解。
最后,我们还需要编写一个名为list.jsp的JSP页面,用来显示所有的学生信息:
```html
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<title>学生列表</title>
</head>
<body>
<h1>学生列表</h1>
<c:if test="${not empty message}">
<div style="color: red;">${message}</div>
</c:if>
<table border="1">
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>编辑</th>
<th>删除</th>
</tr>
<c:forEach items="${students}" var="student">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>${student.age}</td>
<td><a href="${pageContext.request.contextPath}/student/edit/${student.id}">编辑</a></td>
<td><a href="${pageContext.request.contextPath}/student/delete/${student.id}" onclick="return confirm('确定要删除吗?')">删除</a></td>
</tr>
</c:forEach>
</table>
<br>
<a href="${pageContext.request.contextPath}/student/add">添加学生</a>
</body>
</html>
```
这个页面使用了JSTL标签库来遍历所有的学生信息,并且提供了编辑和删除链接。
现在,我们完成了一个使用JDBCTemplate、Spring和Druid整合的CRUD示例。您可以根据自己的需要修改和扩展代码。
阅读全文
相关推荐
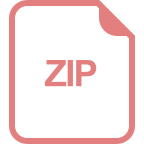
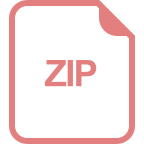














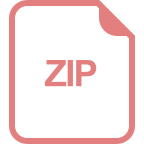
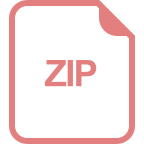