tensorflow estimator的案例代码
时间: 2023-06-09 18:02:48 浏览: 94
下面是一个使用TensorFlow Estimator实现线性回归的案例代码:
```python
import tensorflow as tf
import numpy as np
# 数据集
x_data = np.random.rand(100).astype(np.float32)
y_data = x_data * 0.1 + 0.3
# 定义模型
def model_fn(features, labels, mode):
# 定义权重和偏置
W = tf.Variable(tf.random_uniform([1], -1.0, 1.0))
b = tf.Variable(tf.zeros([1]))
# 构建线性模型
y = W * features['x'] + b
# 预测
predictions = tf.reshape(y, [-1])
if mode == tf.estimator.ModeKeys.PREDICT:
return tf.estimator.EstimatorSpec(mode=mode, predictions=predictions)
# 定义损失函数
loss = tf.reduce_mean(tf.square(predictions - labels))
# 定义优化器
optimizer = tf.train.GradientDescentOptimizer(0.5)
train_op = optimizer.minimize(loss=loss, global_step=tf.train.get_global_step())
# 定义评估指标
eval_metric_ops = {
"mse": tf.metrics.mean_squared_error(labels=labels, predictions=predictions)
}
# 返回EstimatorSpec对象
return tf.estimator.EstimatorSpec(
mode=mode,
predictions=predictions,
loss=loss,
train_op=train_op,
eval_metric_ops=eval_metric_ops
)
# 创建Estimator
estimator = tf.estimator.Estimator(model_fn=model_fn)
# 定义输入函数
input_fn = tf.estimator.inputs.numpy_input_fn(
x={"x": x_data},
y=y_data,
batch_size=8,
num_epochs=None,
shuffle=True
)
# 训练模型
estimator.train(input_fn=input_fn, steps=1000)
# 预测
predict_input_fn = tf.estimator.inputs.numpy_input_fn(
x={"x": np.array([0.1, 0.2, 0.3, 0.4, 0.5], dtype=np.float32)},
y=None,
batch_size=1,
num_epochs=1,
shuffle=False
)
predictions = estimator.predict(predict_input_fn)
for i, p in enumerate(predictions):
print("Prediction %s: %s" % (i+1, p))
```
这个代码中实现了一个简单的线性回归模型,使用了TensorFlow Estimator。第一步是定义模型,这里定义了一个简单的线性模型。然后定义了损失函数和优化器。接着创建了`Estimator`对象,并传入模型函数。然后创建了输入函数,用于传入训练数据和标签。最后调用`train`方法训练模型和`predict`方法进行预测。
阅读全文
相关推荐
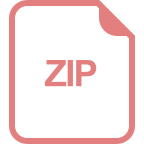
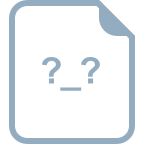
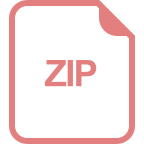
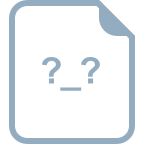
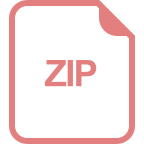
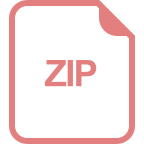
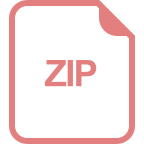
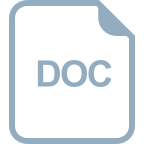
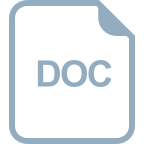
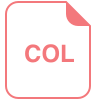
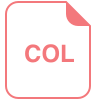
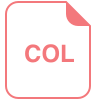
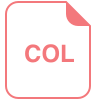
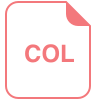
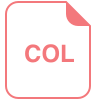
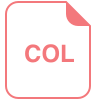