soap转换rest协议,cxf
时间: 2023-11-11 20:38:08 浏览: 158
Apache CXF是一个开源的Web服务框架,它提供了一个开发和部署SOAP和RESTful Web服务的工具。CXF支持SOAP协议,但也可以将SOAP服务转换为RESTful服务。
要将SOAP服务转换为RESTful服务,可以使用CXF的JAX-RS支持。JAX-RS是Java API for RESTful Web Services的缩写,它是Java EE 6规范的一部分。JAX-RS提供了一种简单的方式来创建RESTful Web服务。
以下是将SOAP服务转换为RESTful服务的基本步骤:
1. 在CXF中创建一个新的JAX-RS Web服务。
2. 在新的Web服务中添加适当的注释和方法,以将SOAP请求转换为RESTful请求。
3. 在新的Web服务中添加RESTful响应,以将SOAP响应转换为RESTful响应。
4. 部署新的Web服务并测试它是否按预期工作。
需要注意的是,SOAP和RESTful服务之间存在一些差异。SOAP服务使用XML格式进行通信,而RESTful服务使用JSON或XML格式。此外,SOAP服务通常需要使用WSDL文件来定义服务接口,而RESTful服务通常使用HTTP方法和URL来定义接口。因此,在将SOAP服务转换为RESTful服务时,需要考虑这些差异,并相应地修改代码。
相关问题
使用Apache CXF编写一个REST到SOAP或SOAP到REST的转换程序
Apache CXF是一个流行的Java Web Services框架,它支持REST和SOAP协议,并且提供了丰富的功能和工具,可以帮助开发者快速开发Web Services应用程序。
以下是使用Apache CXF编写一个REST到SOAP或SOAP到REST的转换程序的示例:
1. 添加依赖
首先需要在项目中添加Apache CXF的依赖,可以在项目的pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-core</artifactId>
<version>3.4.0</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-transports-http</artifactId>
<version>3.4.0</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxrs</artifactId>
<version>3.4.0</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxws</artifactId>
<version>3.4.0</version>
</dependency>
```
2. 编写接口
定义一个接口,包含需要暴露的方法,例如:
```java
@Path("/user")
public interface UserService {
@GET
@Path("/{id}")
@Produces("application/json")
User getUserById(@PathParam("id") String id);
@POST
@Path("/")
@Consumes("application/json")
void addUser(User user);
}
```
3. 实现接口
实现接口,并使用CXF提供的注解将其暴露为REST或SOAP服务,例如:
```java
public class UserServiceImpl implements UserService {
@Override
public User getUserById(String id) {
// 从数据库或其他数据源获取用户信息
User user = new User();
user.setId(id);
user.setName("Alice");
user.setAge(20);
return user;
}
@Override
public void addUser(User user) {
// 将用户信息保存到数据库或其他数据源
System.out.println("Add user: " + user);
}
}
```
4. 配置CXF
使用CXF提供的配置方式,将实现类暴露为REST或SOAP服务,例如:
```xml
<jaxrs:server id="restUserService" address="/">
<jaxrs:serviceBeans>
<ref bean="userService"/>
</jaxrs:serviceBeans>
</jaxrs:server>
<jaxws:endpoint id="soapUserService" address="/UserService">
<jaxws:serviceBean>
<ref bean="userService"/>
</jaxws:serviceBean>
</jaxws:endpoint>
```
5. 测试
启动应用程序,并使用浏览器或SOAP客户端发送请求,测试REST或SOAP服务的功能。例如:
REST服务:http://localhost:8080/user/1
SOAP服务:
```xml
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:user="http://example.com/user">
<soapenv:Header/>
<soapenv:Body>
<user:getUserById>
<user:id>1</user:id>
</user:getUserById>
</soapenv:Body>
</soapenv:Envelope>
```
以上就是使用Apache CXF编写REST到SOAP或SOAP到REST的转换程序的示例。需要注意的是,该示例仅用于演示,实际应用中还需要进行更多的配置和调整,以满足实际需求。
帮我编写一个rest转soap的程序
好的,我可以为您提供一些思路和参考代码,但是需要您提供更具体的需求和条件。
以下是一个示例程序,使用了Java语言和Apache CXF框架,将REST请求转换为SOAP请求:
```java
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.xml.namespace.QName;
import javax.xml.soap.SOAPBody;
import javax.xml.soap.SOAPEnvelope;
import javax.xml.soap.SOAPException;
import javax.xml.soap.SOAPFactory;
import javax.xml.soap.SOAPHeader;
import javax.xml.soap.SOAPMessage;
import org.apache.cxf.binding.soap.Soap11;
import org.apache.cxf.binding.soap.SoapBinding;
import org.apache.cxf.binding.soap.SoapMessage;
import org.apache.cxf.jaxrs.JAXRSServiceFactoryBean;
import org.apache.cxf.jaxrs.JAXRSServerFactoryBean;
import org.apache.cxf.jaxrs.lifecycle.SingletonResourceProvider;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import org.apache.cxf.service.Service;
import org.apache.cxf.service.model.BindingInfo;
import org.apache.cxf.service.model.BindingOperationInfo;
import org.apache.cxf.service.model.EndpointInfo;
import org.apache.cxf.service.model.MessagePartInfo;
import org.apache.cxf.service.model.OperationInfo;
import org.apache.cxf.transport.http.HTTPTransportFactory;
@Path("/")
public class RestToSoapConverter {
private static final String SOAP_NAMESPACE_URI = "http://schemas.xmlsoap.org/soap/envelope/";
@GET
@Path("{name}")
@Produces("text/xml")
public String convertRestToSoap(@PathParam("name") String name) throws SOAPException {
// create a SOAP message
SOAPFactory soapFactory = SOAPFactory.newInstance();
SOAPMessage soapMessage = soapFactory.createMessage();
SOAPEnvelope soapEnvelope = soapMessage.getSOAPPart().getEnvelope();
SOAPHeader soapHeader = soapEnvelope.getHeader();
SOAPBody soapBody = soapEnvelope.getBody();
// set the SOAP action header
soapHeader.setAction("urn:hello");
// set the SOAP body content
QName qname = new QName("http://example.com/", "hello", "ns");
MessagePartInfo partInfo = new MessagePartInfo(qname);
OperationInfo operationInfo = new OperationInfo();
BindingOperationInfo bindingOperationInfo = new BindingOperationInfo(null, operationInfo);
bindingOperationInfo.setOperationInfo(operationInfo);
bindingOperationInfo.setBinding(new SoapBinding(null, Soap11.getInstance()));
operationInfo.setName(qname);
operationInfo.setInput("Hello", partInfo);
EndpointInfo endpointInfo = new EndpointInfo(null, "http://localhost:8080/hello");
BindingInfo bindingInfo = new BindingInfo(endpointInfo, "HelloBinding");
bindingInfo.setTransportFactory(new HTTPTransportFactory());
MessagePartInfo messagePartInfo = new MessagePartInfo(qname);
operationInfo.setInput("hello", messagePartInfo);
Service service = new Service();
service.put(QName.class, qname);
service.put(BindingInfo.class, bindingInfo);
service.setInvoker(new HelloInvoker(soapBody));
EndpointInfo ei = new EndpointInfo(service.getServiceInfos().get(0), "");
ei.setAddress("http://localhost:8080/hello");
bindingOperationInfo.setEndpoint(ei);
SoapMessage soapMsg = new SoapMessage(bindingOperationInfo);
soapMsg.setContent(Object.class, soapBody);
soapMsg.setVersion(Soap11.getInstance());
soapMsg.setExchange(new HelloExchange());
// create a JAX-WS client
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(Hello.class);
factory.setAddress("http://localhost:8080/hello");
Hello client = (Hello) factory.create();
// invoke the JAX-WS client and get the SOAP response
SOAPMessage soapResponse = client.sayHello(name);
// convert the SOAP response to a string and return it
return soapMessageToString(soapResponse);
}
private String soapMessageToString(SOAPMessage soapMessage) throws SOAPException {
ByteArrayOutputStream out = new ByteArrayOutputStream();
soapMessage.writeTo(out);
return new String(out.toByteArray());
}
public static void main(String[] args) {
// create a JAX-RS server
JAXRSServerFactoryBean factory = new JAXRSServerFactoryBean();
factory.setResourceClasses(RestToSoapConverter.class);
factory.setResourceProvider(RestToSoapConverter.class, new SingletonResourceProvider(new RestToSoapConverter()));
factory.setAddress("http://localhost:8080/");
factory.create();
// create a JAX-WS server
JAXRSServiceFactoryBean serviceFactory = new JAXRSServiceFactoryBean();
serviceFactory.setResourceClasses(Hello.class);
serviceFactory.setResourceProvider(Hello.class, new SingletonResourceProvider(new HelloImpl()));
serviceFactory.setAddress("http://localhost:8080/hello");
serviceFactory.create();
// start the servers
System.out.println("Server ready...");
System.in.read();
System.out.println("Server shutting down...");
System.exit(0);
}
private static class HelloExchange extends org.apache.cxf.message.ExchangeImpl {
}
private static class HelloInvoker extends org.apache.cxf.service.invoker.AbstractInvoker {
private final SOAPBody soapBody;
public HelloInvoker(SOAPBody soapBody) {
super(null);
this.soapBody = soapBody;
}
@Override
public Object invoke(org.apache.cxf.message.Message message, Object... params) throws Exception {
SOAPMessage soapMessage = message.getContent(SOAPMessage.class);
soapBody.addDocument(soapMessage.getSOAPBody().extractContentAsDocument());
return null;
}
}
public static interface Hello {
public SOAPMessage sayHello(String name);
}
public static class HelloImpl implements Hello {
@Override
public SOAPMessage sayHello(String name) {
try {
SOAPFactory soapFactory = SOAPFactory.newInstance();
SOAPMessage soapMessage = soapFactory.createMessage();
SOAPEnvelope soapEnvelope = soapMessage.getSOAPPart().getEnvelope();
SOAPBody soapBody = soapEnvelope.getBody();
QName qname = new QName("http://example.com/", "helloResponse", "ns");
soapBody.addBodyElement(qname).setTextContent("Hello, " + name + "!");
return soapMessage;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
}
```
以上程序将REST请求转换为SOAP请求,并将SOAP响应转换为字符串返回。您可以根据您的实际需求进行修改和扩展。
阅读全文
相关推荐
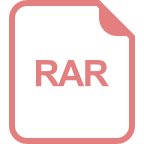
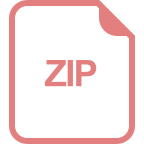
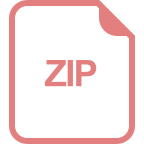
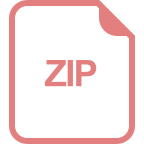
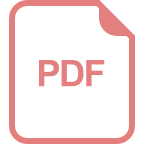
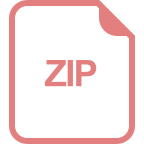
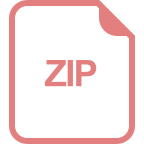
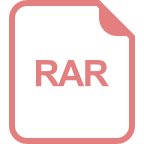
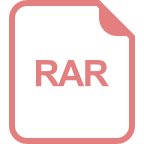
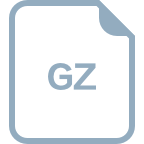
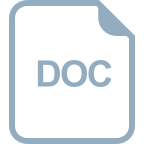
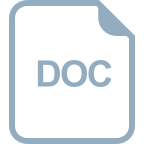
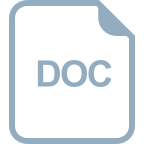
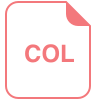