纠正代码#include <iostream> #include <vector> #include <algorithm> using namespace std; int main(); struct Entry; vector<Entry>phone_book(1000); void print_entry(int i) { cout<<phone_book[i].name<<' '<<phone_book[i].number<<'\n'; } void add_entries(int i) { phone_book.resize(phone_book.size()+n); }
时间: 2024-02-24 14:59:08 浏览: 23
这段代码还是有问题的。假设您想要实现一个电话簿,您需要给出 Entry 结构体的定义,包括成员变量 name 和 number。同时,add_entries 函数需要接收一个参数 n,表示要添加的新条目数。以下是可能的纠正代码:
```
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Entry {
string name;
string number;
};
vector<Entry> phone_book(1000);
void print_entry(int i) {
cout << phone_book[i].name << ' ' << phone_book[i].number << '\n';
}
void add_entries(int n) {
phone_book.resize(phone_book.size() + n);
}
int main() {
// 在这里调用函数,添加和打印电话簿条目
return 0;
}
```
请注意,main 函数的声明没有分号,而且需要在函数体内调用其他函数,比如 add_entries 和 print_entry。
相关问题
#include <iostream> #include <vector> #include <algorithm>
#include <iostream> 是C++中的一个头文件,它包含了输入输出流的相关函数和对象,例如cout和cin。通过包含这个头文件,我们可以在程序中使用标准输入输出流来进行输入和输出操作。
#include <vector> 是C++中的一个头文件,它定义了一个名为vector的容器类模板。vector是一个动态数组,可以存储不同类型的元素,并且可以根据需要动态调整大小。
#include <algorithm> 是C++中的一个头文件,它包含了一些常用的算法函数,例如排序、查找、替换等。通过包含这个头文件,我们可以在程序中使用这些算法函数来对容器中的元素进行操作。
#include<iostream> #include<cstdio> using namespace std;是什么意思
#include<iostream> #include<cstdio> using namespace std; 是C++中的预处理指令,用于引入头文件和命名空间。
1. #include<iostream> 是引入iostream头文件,其中包含了输入输出流的定义,例如cout和cin等。
2. #include<cstdio> 是引入cstdio头文件,其中包含了C语言标准输入输出函数的定义,例如printf和scanf等。
3. using namespace std; 是使用std命名空间,std是C++标准库的命名空间,其中包含了很多常用的函数和类。
这些预处理指令的作用是为了在程序中能够使用输入输出流和标准库函数,使得程序更加方便和简洁。
相关推荐
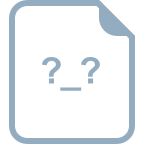













