Qt在vs里写一个小游戏
时间: 2024-03-27 10:36:37 浏览: 96
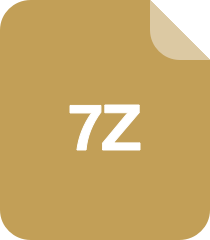
基于QT5.4的C++贪吃蛇小游戏
下面是一个使用Qt在Visual Studio中编写的简单小游戏的示例代码,具体实现了一个简单的扫雷游戏。
```cpp
#include <QApplication>
#include <QMainWindow>
#include <QGridLayout>
#include <QLabel>
#include <QPushButton>
#include <QTime>
class MineSweep : public QMainWindow
{
Q_OBJECT
public:
MineSweep(QWidget *parent = 0);
~MineSweep();
private slots:
void buttonClicked();
private:
int countNearbyMines(int row, int col) const;
void showAllMines();
static const int ROWS = 10;
static const int COLS = 10;
static const int MINES = 10;
QPushButton *m_buttons[ROWS][COLS];
int m_mines[ROWS][COLS];
bool m_visited[ROWS][COLS];
};
MineSweep::MineSweep(QWidget *parent)
: QMainWindow(parent)
{
QGridLayout *grid = new QGridLayout();
QWidget *centralWidget = new QWidget(this);
centralWidget->setLayout(grid);
setCentralWidget(centralWidget);
// 初始化雷区
for (int i = 0; i < ROWS; ++i) {
for (int j = 0; j < COLS; ++j) {
m_mines[i][j] = 0;
m_visited[i][j] = false;
QPushButton *button = new QPushButton(this);
button->setFixedSize(30, 30);
button->setSizePolicy(QSizePolicy::Fixed, QSizePolicy::Fixed);
button->setStyleSheet("text-align: center;");
button->setProperty("row", i);
button->setProperty("col", j);
button->setText("");
connect(button, SIGNAL(clicked()), this, SLOT(buttonClicked()));
m_buttons[i][j] = button;
grid->addWidget(button, i, j);
}
}
// 放置雷
qsrand(QTime::currentTime().msec());
for (int i = 0; i < MINES; ++i) {
int row = qrand() % ROWS;
int col = qrand() % COLS;
m_mines[row][col] = 1;
}
}
MineSweep::~MineSweep()
{
}
void MineSweep::buttonClicked()
{
QPushButton *button = qobject_cast<QPushButton*>(sender());
int row = button->property("row").toInt();
int col = button->property("col").toInt();
// 如果已经被访问过,则直接返回
if (m_visited[row][col]) {
return;
}
// 如果是雷,游戏结束
if (m_mines[row][col] == 1) {
button->setText("*");
showAllMines();
return;
}
// 计算周围雷数
int count = countNearbyMines(row, col);
// 如果周围没有雷,则递归访问周围的方格
if (count == 0) {
button->setText("0");
m_visited[row][col] = true;
for (int i = -1; i <= 1; ++i) {
for (int j = -1; j <= 1; ++j) {
int newRow = row + i;
int newCol = col + j;
if (newRow >= 0 && newRow < ROWS && newCol >= 0 && newCol < COLS) {
buttonClicked(m_buttons[newRow][newCol]);
}
}
}
} else { // 否则显示周围的雷数
button->setText(QString::number(count));
m_visited[row][col] = true;
}
}
int MineSweep::countNearbyMines(int row, int col) const
{
int count = 0;
for (int i = -1; i <= 1; ++i) {
for (int j = -1; j <= 1; ++j) {
int newRow = row + i;
int newCol = col + j;
if (newRow >= 0 && newRow < ROWS && newCol >= 0 && newCol < COLS) {
count += m_mines[newRow][newCol];
}
}
}
return count;
}
void MineSweep::showAllMines()
{
for (int i = 0; i < ROWS; ++i) {
for (int j = 0; j < COLS; ++j) {
if (m_mines[i][j] == 1) {
m_buttons[i][j]->setText("*");
}
}
}
}
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MineSweep w;
w.show();
return a.exec();
}
```
这个小游戏使用了Qt的QPushButton控件来显示方格,并使用QGridLayout对它们进行布局。放置雷的过程中,使用了Qt提供的随机数生成函数qrand()和QTime::currentTime()。在按钮被点击时,会调用buttonClicked()函数来处理,其中会根据当前方格周围的雷数来显示数字或者雷的图标。如果点击的方格是一个雷,则游戏结束,显示所有的雷的位置。
阅读全文
相关推荐
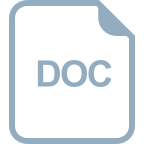
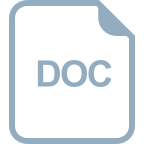
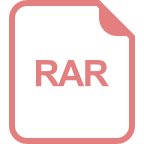
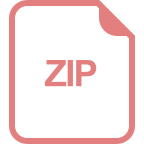
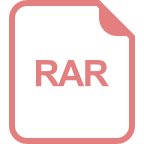
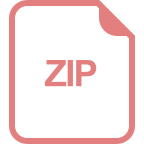
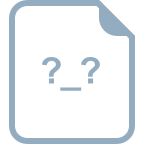
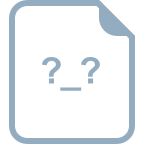
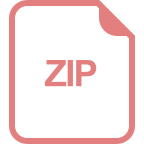
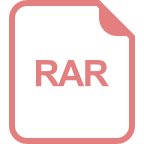
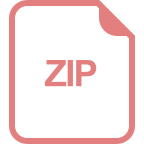
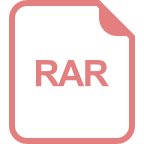
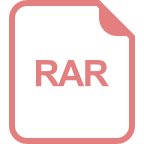

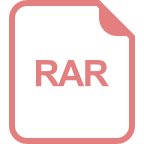
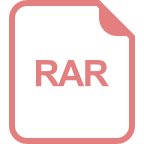
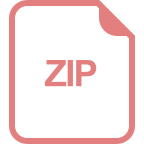