将掩模后的籽粒图像旋转至水平放置的代码
时间: 2024-03-31 17:34:34 浏览: 97
以下是将掩模后的籽粒图像旋转至水平放置的 Python 代码示例:
```python
import cv2
import numpy as np
# 读取掩模后的籽粒图像
img = cv2.imread("grain_masked.png", 0)
# 使用 Canny 边缘检测算法找到图像边缘
edges = cv2.Canny(img, 50, 150, apertureSize=3)
# 使用霍夫直线变换找到图像中最长的一条线
lines = cv2.HoughLines(edges, 1, np.pi / 180, 200)
for line in lines:
rho, theta = line[0]
if theta > np.pi / 4 and theta < 3 * np.pi / 4:
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
cv2.line(img, (x1, y1), (x2, y2), 255, 1)
# 计算旋转角度
angle = np.arctan2(y2 - y1, x2 - x1) * 180 / np.pi
# 旋转图像
(h, w) = img.shape[:2]
center = (w // 2, h // 2)
M = cv2.getRotationMatrix2D(center, -angle, 1.0)
rotated = cv2.warpAffine(img, M, (w, h), flags=cv2.INTER_CUBIC, borderMode=cv2.BORDER_REPLICATE)
# 显示旋转后的图像
cv2.imshow("Rotated Image", rotated)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个代码示例中,我们首先使用 Canny 边缘检测算法找到图像的边缘,然后使用霍夫直线变换找到图像中最长的一条线,计算这条线的斜率,进而计算出需要旋转的角度。最后,使用 OpenCV 中的 `cv2.getRotationMatrix2D` 和 `cv2.warpAffine` 函数来旋转图像。
阅读全文
相关推荐

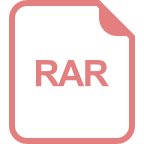
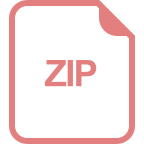
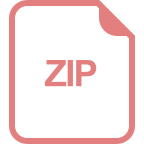
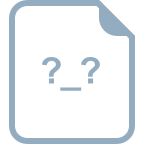
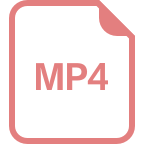
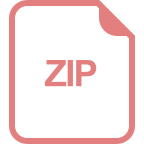
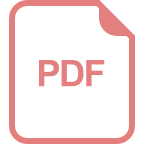
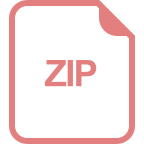
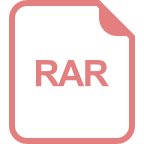
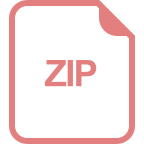
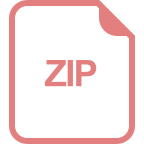