写一个Promise方法
时间: 2023-06-06 10:07:55 浏览: 90
好的,我可以帮您写一个Promise方法。以下是示例代码:
```
function myPromise() {
return new Promise(function(resolve, reject) {
// 这里可以放入异步操作
setTimeout(function() {
// 如果操作成功
resolve('操作成功');
// 如果操作失败
// reject('操作失败');
}, 2000);
});
}
```
您可以使用myPromise()这个方法来执行异步操作,并且使用then()方法获取操作成功后返回的结果,或者使用catch()方法捕获操作失败时的错误。例如:
```
myPromise()
.then(function(result) {
console.log(result);
})
.catch(function(error) {
console.log(error);
});
```
注:以上代码仅为示例,实际使用时需要根据具体场景进行改写。
相关问题
手写一个promise
Promise 是一种异步编程的解决方案,它可以避免回调地狱,让异步操作更加简洁和可读。下面是手写一个 Promise 的基本实现:
```javascript
class MyPromise {
constructor(executor) {
this.status = 'pending';
this.value = undefined;
this.reason = undefined;
this.onResolvedCallbacks = [];
this.onRejectedCallbacks = [];
const resolve = (value) => {
if (this.status === 'pending') {
this.status = 'fulfilled';
this.value = value;
this.onResolvedCallbacks.forEach(fn => fn());
}
};
const reject = (reason) => {
if (this.status === 'pending') {
this.status = 'rejected';
this.reason = reason;
this.onRejectedCallbacks.forEach(fn => fn());
}
};
try {
executor(resolve, reject);
} catch (error) {
reject(error);
}
}
then(onFulfilled, onRejected) {
onFulfilled = typeof onFulfilled === 'function' ? onFulfilled : value => value;
onRejected = typeof onRejected === 'function' ? onRejected : reason => { throw reason };
const promise2 = new MyPromise((resolve, reject) => {
if (this.status === 'fulfilled') {
setTimeout(() => {
try {
const x = onFulfilled(this.value);
this.resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
} else if (this.status === 'rejected') {
setTimeout(() => {
try {
const x = onRejected(this.reason);
this.resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
} else {
this.onResolvedCallbacks.push(() => {
setTimeout(() => {
try {
const x = onFulfilled(this.value);
this.resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
});
this.onRejectedCallbacks.push(() => {
setTimeout(() => {
try {
const x = onRejected(this.reason);
this.resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
});
}
});
return promise2;
}
resolvePromise(promise2, x, resolve, reject) {
if (promise2 === x) {
return reject(new TypeError('Chaining cycle detected for promise'));
}
let called = false;
if (x !== null && (typeof x === 'object' || typeof x === 'function')) {
try {
const then = x.then;
if (typeof then === 'function') {
then.call(x, y => {
if (called) return;
called = true;
this.resolvePromise(promise2, y, resolve, reject);
}, r => {
if (called) return;
called = true;
reject(r);
});
} else {
resolve(x);
}
} catch (error) {
if (called) return;
called = true;
reject(error);
}
} else {
resolve(x);
}
}
catch(onRejected) {
return this.then(null, onRejected);
}
finally(onFinally) {
return this.then(
value => MyPromise.resolve(onFinally()).then(() => value),
reason => MyPromise.resolve(onFinally()).then(() => { throw reason })
);
}
static resolve(value) {
return new MyPromise(resolve => resolve(value));
}
static reject(reason) {
return new MyPromise((resolve, reject) => reject(reason));
}
static all(promises) {
return new MyPromise((resolve, reject) => {
const results = [];
let count = 0;
const processResult = (index, result) => {
results[index] = result;
count++;
if (count === promises.length) {
resolve(results);
}
};
for (let i = 0; i < promises.length; i++) {
promises[i].then(result => processResult(i, result), reject);
}
});
}
static race(promises) {
return new MyPromise((resolve, reject) => {
for (let i = 0; i < promises.length; i++) {
promises[i].then(resolve, reject);
}
});
}
}
```
这个 Promise 实现包含了基本的状态管理、链式调用、错误处理、异步执行、静态方法等功能。当然,这只是一个简单的实现,还有很多细节和边界情况需要考虑。
手写一个promise实现
好的,以下是一个简单的手写Promise实现:
```javascript
class MyPromise {
constructor(executor) {
this.state = 'pending';
this.value = null;
this.reason = null;
this.onFulfilledCallbacks = [];
this.onRejectedCallbacks = [];
const resolve = (value) => {
if (this.state === 'pending') {
this.state = 'fulfilled';
this.value = value;
this.onFulfilledCallbacks.forEach((callback) => callback(this.value));
}
};
const reject = (reason) => {
if (this.state === 'pending') {
this.state = 'rejected';
this.reason = reason;
this.onRejectedCallbacks.forEach((callback) => callback(this.reason));
}
};
try {
executor(resolve, reject);
} catch (error) {
reject(error);
}
}
then(onFulfilled, onRejected) {
onFulfilled = typeof onFulfilled === 'function' ? onFulfilled : (value) => value;
onRejected = typeof onRejected === 'function' ? onRejected : (reason) => { throw reason; };
const promise2 = new MyPromise((resolve, reject) => {
if (this.state === 'fulfilled') {
setTimeout(() => {
try {
const x = onFulfilled(this.value);
this.resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
} else if (this.state === 'rejected') {
setTimeout(() => {
try {
const x = onRejected(this.reason);
this.resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
} else {
this.onFulfilledCallbacks.push(() => {
setTimeout(() => {
try {
const x = onFulfilled(this.value);
this.resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
});
this.onRejectedCallbacks.push(() => {
setTimeout(() => {
try {
const x = onRejected(this.reason);
this.resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
});
}
});
return promise2;
}
catch(onRejected) {
return this.then(null, onRejected);
}
resolvePromise(promise2, x, resolve, reject) {
if (promise2 === x) {
reject(new TypeError('Chaining cycle detected'));
}
let called = false;
if (x instanceof MyPromise) {
x.then((value) => {
this.resolvePromise(promise2, value, resolve, reject);
}, (reason) => {
if (!called) {
called = true;
reject(reason);
}
});
} else if (x !== null && (typeof x === 'object' || typeof x === 'function')) {
try {
const then = x.then;
if (typeof then === 'function') {
then.call(x, (value) => {
if (!called) {
called = true;
this.resolvePromise(promise2, value, resolve, reject);
}
}, (reason) => {
if (!called) {
called = true;
reject(reason);
}
});
} else {
resolve(x);
}
} catch (error) {
if (!called) {
called = true;
reject(error);
}
}
} else {
resolve(x);
}
}
static resolve(value) {
return new MyPromise((resolve) => {
resolve(value);
});
}
static reject(reason) {
return new MyPromise((resolve, reject) => {
reject(reason);
});
}
static all(promises) {
const results = [];
return new MyPromise((resolve, reject) => {
for (let i = 0; i < promises.length; i++) {
const promise = promises[i];
if (!(promise instanceof MyPromise)) {
promise = MyPromise.resolve(promise);
}
promise.then((value) => {
results[i] = value;
if (results.length === promises.length) {
resolve(results);
}
}, (reason) => {
reject(reason);
});
}
});
}
static race(promises) {
return new MyPromise((resolve, reject) => {
for (let i = 0; i < promises.length; i++) {
const promise = promises[i];
if (!(promise instanceof MyPromise)) {
promise = MyPromise.resolve(promise);
}
promise.then((value) => {
resolve(value);
}, (reason) => {
reject(reason);
});
}
});
}
}
```
这个实现包含了Promise的基本功能,包括resolve、reject、then、catch、resolvePromise等方法,并且还实现了Promise.all和Promise.race方法。但是这个实现并不完整,还有很多细节需要考虑和完善。
阅读全文
相关推荐
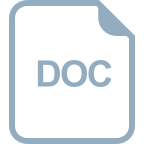
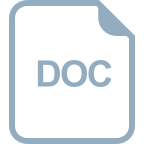
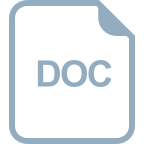
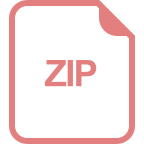











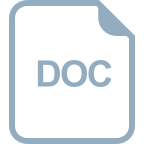
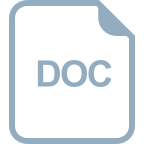
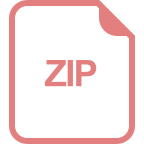