java液态效果
时间: 2023-10-11 21:18:00 浏览: 117
Java中可以通过使用JavaFX技术来实现液态效果。JavaFX提供了一个名为Canvas的类,可用于绘制2D图形。液态效果可以通过绘制随机的圆形并将其填充为半透明的颜色来实现。当圆形相互重叠时,它们会混合颜色,从而产生流动的液态效果。
下面是一个简单的JavaFX程序,演示了如何实现液态效果:
```java
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import java.util.Random;
public class LiquidEffect extends Application {
private final int WIDTH = 800; // 画布宽度
private final int HEIGHT = 600; // 画布高度
private final int NUM_CIRCLES = 1000; // 圆形数量
private Random random = new Random();
private Circle[] circles = new Circle[NUM_CIRCLES];
@Override
public void start(Stage primaryStage) throws Exception {
Canvas canvas = new Canvas(WIDTH, HEIGHT);
GraphicsContext gc = canvas.getGraphicsContext2D();
// 初始化圆形
for (int i = 0; i < NUM_CIRCLES; i++) {
circles[i] = new Circle(random.nextInt(WIDTH), random.nextInt(HEIGHT), random.nextInt(20) + 10);
}
// 动画循环
new AnimationTimer() {
@Override
public void handle(long now) {
// 清空画布
gc.clearRect(0, 0, WIDTH, HEIGHT);
// 绘制圆形
for (int i = 0; i < NUM_CIRCLES; i++) {
// 随机改变圆形位置
circles[i].x += random.nextInt(3) - 1;
circles[i].y += random.nextInt(3) - 1;
// 确保圆形在画布内
circles[i].x = Math.max(circles[i].r, Math.min(WIDTH - circles[i].r, circles[i].x));
circles[i].y = Math.max(circles[i].r, Math.min(HEIGHT - circles[i].r, circles[i].y));
// 绘制圆形
gc.setFill(new Color(1, 1, 1, 0.1)); // 半透明颜色
gc.fillOval(circles[i].x - circles[i].r, circles[i].y - circles[i].r, circles[i].r * 2, circles[i].r * 2);
}
}
}.start();
Group root = new Group(canvas);
Scene scene = new Scene(root);
primaryStage.setScene(scene);
primaryStage.show();
}
private static class Circle {
int x, y, r;
public Circle(int x, int y, int r) {
this.x = x;
this.y = y;
this.r = r;
}
}
public static void main(String[] args) {
launch(args);
}
}
```
该程序随机生成1000个圆形,并在每一帧中改变它们的位置,然后用半透明颜色绘制在画布上,产生流动的液态效果。
阅读全文
相关推荐



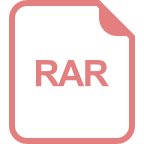

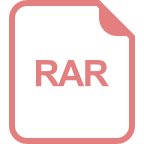







