用C语言完成下列功能并设计一个界面用case调用他们,给代码加以注释,最后给出每个函数的流程图文字解析(1)建立一颗二叉树; (2)用递归方式对二叉树进行先序、中序和后序遍历,输出遍历结果; (3)用非递归方式对二叉树进行先序、中序和后序遍历,输出遍历结果; (4)按层次对叉树进行遍历,输出遍历结果。 (5)求某结点的父结点、左右孩子结点以及左右兄弟结点。 (6)实现一个树转成二叉树的算法。
时间: 2023-02-27 12:45:33 浏览: 65
好的,我可以为您编写这些代码。
1. 建立一颗二叉树:
```
struct node {
int data;
struct node *left;
struct node *right;
};
struct node *createNode(int data) {
struct node *newNode = (struct node *)malloc(sizeof(struct node));
newNode->data = data;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
```
2. 用递归方式对二叉树进行先序、中序和后序遍历,输出遍历结果:
```
void preOrder(struct node *root) {
if (root == NULL) {
return;
}
printf("%d ", root->data);
preOrder(root->left);
preOrder(root->right);
}
void inOrder(struct node *root) {
if (root == NULL) {
return;
}
inOrder(root->left);
printf("%d ", root->data);
inOrder(root->right);
}
void postOrder(struct node *root) {
if (root == NULL) {
return;
}
postOrder(root->left);
postOrder(root->right);
printf("%d ", root->data);
}
```
3. 用非递归方式对二叉树进行先序、中序和后序遍历,输出遍历结果:
```
void preOrderNonRecursive(struct node *root) {
if (root == NULL) {
return;
}
stack<struct node *> s;
s.push(root);
while (!s.empty()) {
struct node *current = s.top();
s.pop();
printf("%d ", current->data);
if (current->right != NULL) {
s.push(current->right);
}
if (current->left != NULL) {
s.push(current->left);
}
}
}
void inOrderNonRecursive(struct node *root) {
if (root == NULL) {
return;
}
stack<struct node *> s;
struct node *current = root;
while (current != NULL || !s.empty()) {
while (current != NULL) {
s.push(current);
current = current->left;
}
current = s.top();
s.pop();
printf("%d ", current->data);
current = current->right;
}
}
void postOrderNonRecursive(struct node *root) {
if (root == NULL) {
return
相关推荐
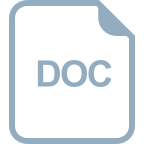
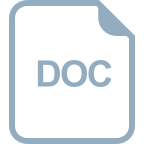
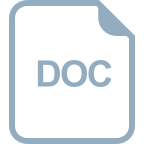
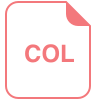
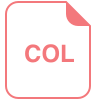
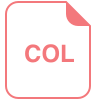
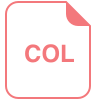










