Java instanceof()方法 找两个非常简单的例子
时间: 2023-10-29 17:05:48 浏览: 56
例子1:判断对象是否为某个类的实例
```java
String str = "Hello World";
if (str instanceof String) {
System.out.println("str is an instance of String");
}
```
输出结果为:
```
str is an instance of String
```
例子2:判断对象是否为某个基本数据类型的实例
```java
int num = 10;
if (num instanceof Integer) {
System.out.println("num is an instance of Integer");
}
```
这段代码会编译失败,因为基本数据类型不能使用 instanceof 进行判断。
相关问题
java instanceof()方法 找两个相关例子
例子1:判断对象是否为某个类或其子类的实例
```java
public class Animal {
public void eat() {
System.out.println("Animal eat");
}
}
public class Cat extends Animal {
public void meow() {
System.out.println("Cat meow");
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("Dog bark");
}
}
public class InstanceOfExample {
public static void main(String[] args) {
Animal animal1 = new Cat();
Animal animal2 = new Dog();
if (animal1 instanceof Cat) {
((Cat) animal1).meow();
}
if (animal2 instanceof Dog) {
((Dog) animal2).bark();
}
}
}
```
输出结果为:
```
Cat meow
Dog bark
```
例子2:判断对象是否为某个接口的实现类的实例
```java
public interface Shape {
void draw();
}
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Circle draw");
}
}
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Rectangle draw");
}
}
public class InstanceOfExample {
public static void main(String[] args) {
Shape shape1 = new Circle();
Shape shape2 = new Rectangle();
if (shape1 instanceof Circle) {
shape1.draw();
}
if (shape2 instanceof Rectangle) {
shape2.draw();
}
}
}
```
输出结果为:
```
Circle draw
Rectangle draw
```
java 的instanceof
`instanceof`是Java中的一个关键字,用于测试一个对象是否是一个类的实例。它的语法如下:
```
object instanceof class
```
其中,`object`是要测试的对象,`class`是要测试的类。如果`object`是`class`的一个实例,则返回`true`,否则返回`false`。
以下是一个使用`instanceof`的例子:
```java
public class Animal {
public void makeSound() {
System.out.println("The animal makes a sound");
}
}
public class Dog extends Animal {
public void makeSound() {
System.out.println("The dog barks");
}
}
public class Cat extends Animal {
public void makeSound() {
System.out.println("The cat meows");
}
}
public class InstanceofExample {
public static void main(String[] args) {
Animal animal = new Dog();
if (animal instanceof Dog) {
Dog dog = (Dog) animal;
dog.makeSound();
} else if (animal instanceof Cat) {
Cat cat = (Cat) animal;
cat.makeSound();
} else {
animal.makeSound();
}
}
}
```
在上面的例子中,我们定义了一个`Animal`类和两个子类`Dog`和`Cat`。在`InstanceofExample`类中,我们创建了一个`Animal`对象,并将其赋值为`Dog`类型。然后,我们使用`instanceof`测试`animal`是否是`Dog`的一个实例。由于`animal`是`Dog`的一个实例,因此`instanceof`返回`true`。然后,我们将`animal`强制转换为`Dog`类型,并调用`makeSound()`方法,输出`The dog barks`。
阅读全文
相关推荐
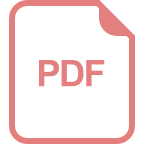
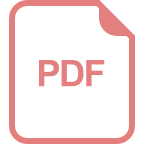
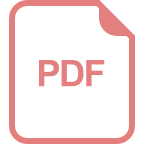

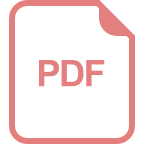
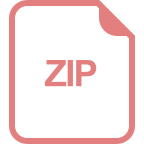
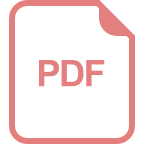
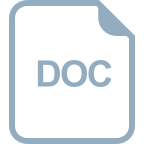





